class Item: def init(self,l,t,w,h,index,font): self.rect = pygame.Rect(l,t,w,h) self.index = index self.font = font def display_names(self,surface,name,cost,selected): color = TEXT_COLOR_SELECTED if selected else TEXT_COLOR title_surf = self.font.render(name,False,color) title_rect = title_surf.get_rect(midtop = self.rect.midtop + pygame.math.Vector2(0,20)) cost_surf = self.font.render(f'{int(cost)}',False,color) cost_rect = cost_surf.get_rect(midbottom = self.rect.midbottom - pygame.math.Vector2(0,20)) surface.blit(title_surf,title_rect) surface.blit(cost_surf,cost_rect) def display_bar(self,surface,value,max_value,selected): # drawing setup top = self.rect.midtop + pygame.math.Vector2(0,60) bottom = self.rect.midbottom - pygame.math.Vector2(0,60) color = BAR_COLOR_SELECTED if selected else BAR_COLOR # bar setup full_height = bottom[1] - top[1] relative_number = (value / max_value) * full_height value_rect = pygame.Rect(top[0] - 15,bottom[1] - relative_number,30,10) # draw elements pygame.draw.line(surface,color,top,bottom,5) pygame.draw.rect(surface,color,value_rect) def trigger(self,player): upgrade_attribute = list(player.stats.keys())[self.index] if player.exp >= player.upgrade_cost[upgrade_attribute] and player.stats[upgrade_attribute] < player.max_stats[upgrade_attribute]: player.exp -= player.upgrade_cost[upgrade_attribute] player.stats[upgrade_attribute] *= 1.2 player.upgrade_cost[upgrade_attribute] *= 1.4 if player.stats[upgrade_attribute] > player.max_stats[upgrade_attribute]: player.stats[upgrade_attribute] = player.max_stats[upgrade_attribute] def display(self,surface,selection_num,name,value,max_value,cost): if self.index == selection_num: pygame.draw.rect(surface,UPGRADE_BG_COLOR_SELECTED,self.rect) pygame.draw.rect(surface,UI_BORDER_COLOR,self.rect,4) else: pygame.draw.rect(surface,UI_BG_COLOR,self.rect) pygame.draw.rect(surface,UI_BORDER_COLOR,self.rect,4) self.display_names(surface,name,cost,self.index == selection_num) self.display_bar(surface,value,max_value,self.index == selection_num)
时间: 2024-02-29 16:53:55 浏览: 30
这段代码是用 Python 编写的一个类 Item,其中定义了一些方法,包括 display_names、display_bar 和 trigger。这些方法分别用于显示物品名称和花费、显示进度条和触发物品效果。该类的实例可以在界面上显示物品信息,并且在玩家选中该物品时会显示不同的背景颜色。该类的实例还可以被玩家触发,以实现物品效果。
相关问题
帮我把下面代码添加注释class Button: def __init__(self, text='按钮', left=0, top=0, width=90, height=30, color=(200, 200, 200)): self.text = text self.color = color self.rect = pygame.Rect(left, top, width, height) self.font = pygame.font.Font(r"C:\Windows\Fonts\simsun.ttc", 16) self.text_surface = self.font.render(self.text, True, (0, 0, 0)) self.text_rect = self.text_surface.get_rect() # self.text_rect.center = self.rect.center self.active = False self.function = None button_group.append(self) def set_text(self, new_text): self.text = new_text self.text_surface = self.font.render(self.text, True, (0, 0, 0)) self.text_rect = self.text_surface.get_rect() def draw(self, screen): if self.active: pygame.draw.rect(screen, (180, 180, 200), self.rect, 0) else: pygame.draw.rect(screen, self.color, self.rect, 0) self.text_rect.center = self.rect.center screen.blit(self.text_surface, self.text_rect) def get_click(self, event): if event.type == pygame.MOUSEBUTTONDOWN: if event.button == 1: mx, my = event.pos if self.rect.left <= mx <= self.rect.right and self.rect.top <= my <= self.rect.bottom: self.active = True elif event.type == pygame.MOUSEBUTTONUP and self.active: if event.button == 1: mx, my = event.pos if self.rect.left <= mx <= self.rect.right and self.rect.top <= my <= self.rect.bottom: # print(f'{self.text}按钮被点击') if callable(self.function): self.function() self.active = False def click_connect(self, function): self.function = function
以下是代码的注释:
```
class Button:
def __init__(self, text='按钮', left=0, top=0, width=90, height=30, color=(200, 200, 200)):
# 初始化函数,设置按钮的文本、位置、大小和颜色
self.text = text
self.color = color
self.rect = pygame.Rect(left, top, width, height) # 按钮的矩形区域
self.font = pygame.font.Font(r"C:\Windows\Fonts\simsun.ttc", 16) # 按钮文本的字体
self.text_surface = self.font.render(self.text, True, (0, 0, 0)) # 渲染按钮文本
self.text_rect = self.text_surface.get_rect() # 获取按钮文本的矩形区域
# self.text_rect.center = self.rect.center
self.active = False # 按钮是否被点击
self.function = None # 按钮被点击时调用的函数
button_group.append(self) # 将按钮对象添加到按钮组中
def set_text(self, new_text):
# 设置按钮的文本
self.text = new_text
self.text_surface = self.font.render(self.text, True, (0, 0, 0))
self.text_rect = self.text_surface.get_rect()
def draw(self, screen):
# 绘制按钮
if self.active:
pygame.draw.rect(screen, (180, 180, 200), self.rect, 0)
else:
pygame.draw.rect(screen, self.color, self.rect, 0)
self.text_rect.center = self.rect.center
screen.blit(self.text_surface, self.text_rect)
def get_click(self, event):
# 获取鼠标点击事件
if event.type == pygame.MOUSEBUTTONDOWN:
if event.button == 1:
mx, my = event.pos
if self.rect.left <= mx <= self.rect.right and self.rect.top <= my <= self.rect.bottom:
self.active = True
elif event.type == pygame.MOUSEBUTTONUP and self.active:
if event.button == 1:
mx, my = event.pos
if self.rect.left <= mx <= self.rect.right and self.rect.top <= my <= self.rect.bottom:
# print(f'{self.text}按钮被点击')
if callable(self.function):
self.function()
self.active = False
def click_connect(self, function):
# 将按钮与函数连接,当按钮被点击时调用函数
self.function = function
```
import pygame from pygame.mixer import music import random class Ball(pygame.sprite.Sprite): def __init__(self,image_file,location,speed): pygame.sprite.Sprite.__init__(self) self.image = pygame.image.load(image_file) self.rect = self.image.get_rect() self.rect.left,self.rect.top = location self.speed = speed def move(self): self.rect = self.rect.move(self.speed) if self.rect.left < 0 or self.rect.right > width: self.speed[0] = -self.speed[0] if self.rect.top < 0 and (self.rect.left < 240 or self.rect.right > 400) : self.speed[1] = -self.speed[1] pygame.init() pygame.mixer.init() # 初始化混音器 clock = pygame.time.Clock() pygame.key.set_repeat(500,50) size = width,height = 640,480 screen = pygame.display.set_mode(size) screen.fill([255,255,255]) ball = Ball("desk_ball.png",[320,240],[10,8]) def new_func(Ball): bat = Ball("bat.png",[320,460],[0,0]) return bat bat = new_func(Ball) goal = Ball("goal.png",[240,0],[0,0]) screen.blit(ball.image,ball.rect) pygame.display.set_caption('乒乓球小游戏') #游戏标题 pygame.display.update() score = 0 lives = 5#总共有5个球 music.load("bg.mp3") # 加载背景音乐 music.play(-1) # 循环播放背景音乐,直到程序退出 done = False running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False if event.type == pygame.MOUSEMOTION: bat.rect.centerx = event.pos[0] if event.type == pygame.KEYDOWN: if event.key == pygame.K_y and lives == 0: lives = 5 done = False elif event.key == pygame.K_n and lives == 0: running = False if not done: ball.move() if pygame.sprite.collide_rect(ball,bat): ball.speed[1] = -10 if pygame.sprite.collide_rect(ball,goal): score += 1 ball.speed[1] = 10 screen.blit(ball.image,ball.rect) screen.blit(bat.image,bat.rect) for num in range(lives-1): screen.blit(ball.image,[600-num*40,0]) if ball.rect.bottom > height: lives -= 1 ball.rect.left,ball.rect.top = 320,240 if lives == 0: done = True else: over_font = pygame.font.Font(None,50) over_surf = over_font.render("Game over",1,[255,0,0]) screen.blit(over_surf,[240,240]) yn_font = pygame.font.Font(None,40) yn_surf = yn_font.render("Y:continue N:quit",1,[255,0,0]) screen.blit(yn_surf,[210,280]) score_font = pygame.font.Font(None,40) score_surf = score_font.render("score:"+str(score),1,[255,0,0]) screen.blit(score_surf,[0,0]) screen.blit(goal.image,goal.rect) pygame.display.update() clock.tick(20) screen.fill([255,255,255]) pygame.quit()基于这些代码补充在游戏界面加一条分割线
在代码中添加以下代码可以在游戏界面上添加一条分割线:
```python
# 画一条分割线
pygame.draw.line(screen, [0, 0, 0], [0, 240], [640, 240], 5)
```
将其添加在`screen.fill([255,255,255])`之后,完整代码如下:
```python
import pygame
from pygame.mixer import music
import random
class Ball(pygame.sprite.Sprite):
def __init__(self,image_file,location,speed):
pygame.sprite.Sprite.__init__(self)
self.image = pygame.image.load(image_file)
self.rect = self.image.get_rect()
self.rect.left,self.rect.top = location
self.speed = speed
def move(self):
self.rect = self.rect.move(self.speed)
if self.rect.left < 0 or self.rect.right > width:
self.speed[0] = -self.speed[0]
if self.rect.top < 0 and (self.rect.left < 240 or self.rect.right > 400) :
self.speed[1] = -self.speed[1]
pygame.init()
pygame.mixer.init() # 初始化混音器
clock = pygame.time.Clock()
pygame.key.set_repeat(500,50)
size = width,height = 640,480
screen = pygame.display.set_mode(size)
screen.fill([255,255,255])
ball = Ball("desk_ball.png",[320,240],[10,8])
def new_func(Ball):
bat = Ball("bat.png",[320,460],[0,0])
return bat
bat = new_func(Ball)
goal = Ball("goal.png",[240,0],[0,0])
screen.blit(ball.image,ball.rect)
pygame.display.set_caption('乒乓球小游戏') #游戏标题
pygame.display.update()
score = 0
lives = 5#总共有5个球
music.load("bg.mp3") # 加载背景音乐
music.play(-1) # 循环播放背景音乐,直到程序退出
done = False
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.MOUSEMOTION:
bat.rect.centerx = event.pos[0]
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_y and lives == 0:
lives = 5
done = False
elif event.key == pygame.K_n and lives == 0:
running = False
if not done:
ball.move()
if pygame.sprite.collide_rect(ball,bat):
ball.speed[1] = -10
if pygame.sprite.collide_rect(ball,goal):
score += 1
ball.speed[1] = 10
screen.blit(ball.image,ball.rect)
screen.blit(bat.image,bat.rect)
for num in range(lives-1):
screen.blit(ball.image,[600-num*40,0])
if ball.rect.bottom > height:
lives -= 1
ball.rect.left,ball.rect.top = 320,240
if lives == 0:
done = True
else:
over_font = pygame.font.Font(None,50)
over_surf = over_font.render("Game over",1,[255,0,0])
screen.blit(over_surf,[240,240])
yn_font = pygame.font.Font(None,40)
yn_surf = yn_font.render("Y:continue N:quit",1,[255,0,0])
screen.blit(yn_surf,[210,280])
score_font = pygame.font.Font(None,40)
score_surf = score_font.render("score:"+str(score),1,[255,0,0])
screen.blit(score_surf,[0,0])
# 画一条分割线
pygame.draw.line(screen, [0, 0, 0], [0, 240], [640, 240], 5)
screen.blit(goal.image,goal.rect)
pygame.display.update()
clock.tick(20)
screen.fill([255,255,255])
pygame.quit()
```
相关推荐
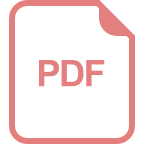
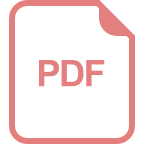
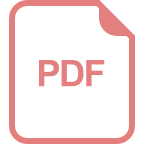













