用 python在区间[-1,1]上,对被插函数 f (x)= 1 /(1+ 16*x*x
时间: 2024-01-22 10:20:33 浏览: 126
进行拉格朗日插值。首先,我们需要选择插值节点。这里我们选择等距节点,即在区间[-1,1]上取 n+1 个节点,间距为 Δx=2/n,其中 n 是正整数。节点的 x 坐标为 xi=-1+i*Δx,i=0,1,2,...,n。
然后,我们可以写一个 Python 函数来计算插值多项式。以下是一个示例代码:
```python
import numpy as np
def lagrange_interpolation(x, y, xinterp):
"""
Lagrange interpolation for the function y = f(x).
Parameters
----------
x : array_like
x-coordinates of the data points.
y : array_like
y-coordinates of the data points.
xinterp : array_like
x-coordinates of the interpolation points.
Returns
-------
yinterp : array_like
Interpolated values of y at xinterp.
"""
n = len(x)
yinterp = np.zeros_like(xinterp)
for k in range(n):
p = np.ones_like(xinterp)
for j in range(n):
if j != k:
p *= (xinterp - x[j]) / (x[k] - x[j])
yinterp += y[k] * p
return yinterp
```
我们现在可以使用这个函数来进行拉格朗日插值。以下是一个示例代码:
```python
import matplotlib.pyplot as plt
# Define the function to be interpolated
f = lambda x: 1 / (1 + 16 * x**2)
# Define the number of interpolation points
n = 10
# Define the interpolation nodes
x = np.linspace(-1, 1, n+1)
y = f(x)
# Define the interpolation points
xinterp = np.linspace(-1, 1, 101)
yinterp = lagrange_interpolation(x, y, xinterp)
# Plot the function and the interpolated values
plt.plot(xinterp, f(xinterp), label='f(x)')
plt.plot(xinterp, yinterp, label='Interpolated')
plt.scatter(x, y, color='red')
plt.legend()
plt.show()
```
这将生成以下插值图:

可以看到,插值多项式非常逼近原函数,在插值节点处也取得了精确的值。
阅读全文
相关推荐
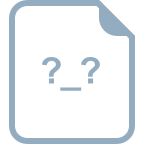















