unity 按下Esc键
时间: 2023-07-01 20:16:34 浏览: 102
在Unity中,按下Esc键可以触发一些操作,比如退出游戏、关闭菜单等。
你可以通过以下代码来实现:
```
if (Input.GetKeyDown(KeyCode.Escape))
{
// 在这里添加你的代码
}
```
在上述代码中,我们使用了Input类的GetKeyDown方法来检测Esc键是否被按下。当Esc键被按下时,就会执行if语句中的代码。你可以在if语句中添加你想要实现的操作代码。比如,如果你想要退出游戏,可以使用Application.Quit()方法来实现:
```
if (Input.GetKeyDown(KeyCode.Escape))
{
Application.Quit(); // 退出游戏
}
```
希望能帮到你!
相关问题
unity实现按Esc光标出现,再次按Esc键光标消失
你可以通过以下代码来实现:
```csharp
void Update()
{
if(Input.GetKeyDown(KeyCode.Escape))
{
Cursor.visible = !Cursor.visible;
Cursor.lockState = Cursor.visible ? CursorLockMode.None : CursorLockMode.Locked;
}
}
```
在 Update 函数中,我们检测是否按下了 Esc 键。如果按下了,就通过 `Cursor.visible` 属性来控制光标的可见性,通过 `Cursor.lockState` 属性来控制光标锁定的状态。当光标可见时,将 `Cursor.lockState` 设置为 `CursorLockMode.None`,这样可以让光标自由移动。当光标不可见时,将 `Cursor.lockState` 设置为 `CursorLockMode.Locked`,这样可以锁定光标在中心位置,防止误操作。
unity代码要求使用DOTween实现打字机效果,按下ESC键后,文字立即全部显示
在Unity中使用DOTween实现打字机效果,可以使用Tween的`Append`方法来逐个打印每个字符,并使用`Input.GetKeyDown(KeyCode.Escape)`来监听ESC键的按下。代码如下:
```c#
using UnityEngine;
using UnityEngine.UI;
using DG.Tweening;
public class TypewriterEffect : MonoBehaviour
{
public float delay = 0.1f; // 每个字的打印速度
public Text textComponent;
public string message;
private bool isTyping = true;
private Tween typingTween;
private void Start()
{
typingTween = DOTween.Sequence();
for (int i = 0; i < message.Length; i++)
{
typingTween.Append(textComponent.DOText(message.Substring(0, i + 1), delay));
}
typingTween.OnComplete(() => isTyping = false);
}
private void Update()
{
if (isTyping && Input.GetKeyDown(KeyCode.Escape))
{
typingTween.Kill(); // 立即显示全部文字
textComponent.text = message;
isTyping = false;
}
}
}
```
首先,在`Start`函数中创建Tween序列`typingTween`,使用`Append`方法逐个打印每个字符,并根据`delay`变量设置打印速度。使用`OnComplete`方法设置当Tween序列完成时执行的回调函数,并将`isTyping`标志位设置为`false`。
然后,在`Update`函数中监听ESC键的按下,如果按下了ESC键,则使用`Kill`方法立即停止Tween序列,并将`textComponent.text`设置为完整的字符串,即可立即显示全部文字。
需要注意的是,在使用Tween时需要使用`DOTween.Sequence()`方法创建Tween序列,并使用`Append`方法逐个添加Tween。另外,在使用Tween时需要注意Tween的生命周期,确保在需要时及时停止Tween。
相关推荐
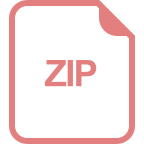












