void drawScene(void) { float R = 20.0; // Radius of helix. float t; // Angle parameter. glClear(GL_COLOR_BUFFER_BIT); glColor3f(0.0, 0.0, 0.0); glBegin(GL_LINE_STRIP); for (t = -10 * M_PI; t <= 10 * M_PI; t += M_PI / 20.0) glVertex3f(R * cos(t), R * sin(t), t - 60.0); // glVertex3f(R * cos(t), t, R * sin(t) - 60.0); glEnd(); glFlush(); }
时间: 2024-01-09 10:04:12 浏览: 27
这段代码实现了一个绕z轴螺旋上升的线条,具体来说:
1. 首先清空窗口,设置画笔颜色为黑色。
2. 然后使用GL_LINE_STRIP模式开始绘制线条。
3. 在循环中,从-10π到10π,每隔M_PI / 20.0一个单位,计算出当前点的坐标,即(x,y,z),其中x和y分别是以R为半径的圆上的点的坐标,z是当前的角度减去60。
4. 将计算出的点添加到线条中。
5. 绘制结束后,刷新缓冲区,使得图像显示出来。
需要注意的是,这段代码使用了OpenGL库,因此需要先安装OpenGL库并配置好开发环境才能正常编译运行。
相关问题
#define _USE_MATH_DEFINES #include <cstdlib> #include <cmath> #include <iostream> #include <GL/glew.h> #include <GL/freeglut.h> // Globals. static float R = 40.0; // Radius of circle. static float X = 50.0; // X-coordinate of center of circle. static float Y = 50.0; // Y-coordinate of center of circle. static const int numVertices = 50; // Number of vertices on circle. static int verticesColors[6 * numVertices]; void generateVertices() { float t = 0; // Angle parameter. for (int i = 0; i < 6*numVertices; i+=6) { verticesColors[] = X + R * cos(t); //x verticesColors[] = Y + R * sin(t); //y verticesColors[] = 0.0; //z verticesColors[] = 1.0; //r verticesColors[] = 0.0; //g verticesColors[] = 0.0; //b t += 2 * M_PI / numVertices; //angle } } // Drawing routine. void drawScene(void) { glClear(GL_COLOR_BUFFER_BIT); glColor3f(1, 0, 0); glLineWidth(5); glDrawArrays(GL_LINE_LOOP, 0, 50); glFlush(); } // Initialization routine. void setup(void) { glClearColor(1.0, 1.0, 1.0, 0.0); glEnableClientState(GL_VERTEX_ARRAY); glEnableClientState(GL_COLOR_ARRAY); glVertexPointer(3, GL_FLOAT, 6 * sizeof(float), &verticesColors[0]); glVertexPointer(3, GL_FLOAT, 6 * sizeof(float), &verticesColors[3]) } // OpenGL window reshape routine. void resize(int w, int h) { glViewport(0, 0, w, h); glMatrixMode(GL_PROJECTION); glLoadIdentity(); glOrtho(0.0, 100.0, 0.0, 100.0, -1.0, 1.0); glMatrixMode(GL_MODELVIEW); glLoadIdentity(); } // Keyboard input processing routine. void keyInput(unsigned char key, int x, int y) { switch (key) { case 27: exit(0); break; default: break; } } // Main routine. int main(int argc, char** argv) { generateVertices(); glutInit(&argc, argv); glutInitContextVersion(4, 3); glutInitContextProfile(GLUT_COMPATIBILITY_PROFILE); glutInitDisplayMode(GLUT_SINGLE | GLUT_RGBA); glutInitWindowSize(500, 500); glutInitWindowPosition(100, 100); glutCreateWindow("circle.cpp"); glutDisplayFunc(drawScene); glutReshapeFunc(resize); glutKeyboardFunc(keyInput); glewExperimental = GL_TRUE; glewInit(); setup(); glutMainLoop(); }怎么修改
这段代码存在一些错误,需要进行修改,具体如下:
1. 在函数 generateVertices 中,数组 verticesColors[] 的下标没有被正确设置,应该是 verticesColors[i]。
2. 在函数 setup 中,第二个 glEnableClientState(GL_COLOR_ARRAY) 应该是 glEnableClientState(GL_COLOR_ARRAY);。
3. 在函数 setup 中,第一个 glVertexPointer 函数应该是 glColorPointer。
4. 在函数 drawScene 中,应该使用 glDrawArrays(GL_POLYGON, 0, numVertices) 来绘制圆形。
修改后的代码如下:
使用opengl语言实现鼠标左键取点显示并储存,并在点击右键时将点一一相连,在点击右键前不进行连线
以下是使用OpenGL语言实现鼠标左键取点显示并储存,并在点击右键时将点一一相连,在点击右键前不进行连线的代码示例:
```c++
#include <GL/glut.h>
#include <iostream>
#include <vector>
using namespace std;
// 定义窗口大小
int windowWidth = 800, windowHeight = 600;
// 定义存储点的数组
vector<pair<int, int>> points;
// 定义当前鼠标位置
int mouseX = 0, mouseY = 0;
// 定义是否进行连线的标志
bool connectPoints = false;
// 绘制场景
void drawScene() {
glClear(GL_COLOR_BUFFER_BIT);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluOrtho2D(0, windowWidth, 0, windowHeight);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
// 绘制点
glColor3f(1.0f, 0.0f, 0.0f);
glPointSize(5.0f);
glBegin(GL_POINTS);
for (int i = 0; i < points.size(); i++) {
glVertex2i(points[i].first, points[i].second);
}
glEnd();
// 绘制连线
if (connectPoints) {
glColor3f(0.0f, 1.0f, 0.0f);
glBegin(GL_LINE_STRIP);
for (int i = 0; i < points.size(); i++) {
glVertex2i(points[i].first, points[i].second);
}
glEnd();
}
glutSwapBuffers();
}
// 鼠标事件处理函数
void mouse(int button, int state, int x, int y) {
if (button == GLUT_LEFT_BUTTON && state == GLUT_DOWN) {
// 左键按下,添加点
points.push_back(make_pair(x, windowHeight - y));
glutPostRedisplay();
} else if (button == GLUT_RIGHT_BUTTON && state == GLUT_DOWN) {
// 右键按下,进行连线
connectPoints = true;
glutPostRedisplay();
}
}
// 鼠标移动事件处理函数
void mouseMove(int x, int y) {
mouseX = x;
mouseY = windowHeight - y;
}
// 主函数
int main(int argc, char** argv) {
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGBA);
glutInitWindowSize(windowWidth, windowHeight);
glutCreateWindow("OpenGL");
glutDisplayFunc(drawScene);
glutMouseFunc(mouse);
glutPassiveMotionFunc(mouseMove);
glutMainLoop();
return 0;
}
```
这段代码实现了鼠标左键取点显示并储存,右键点击时将点一一相连,在点击右键前不进行连线。在程序运行时,可以使用鼠标左键点击窗口中的任意位置添加点,右键点击时将所有点连接起来。
相关推荐
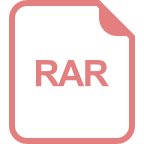
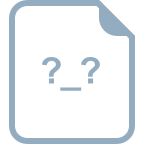
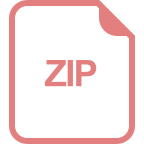












