稀疏矩阵的加法,输入任意两个稀疏矩阵A和B,求出它们的和矩阵C 输入行数、列数和非零元素,创建两个稀疏矩阵。 实现加法运算 输出稀疏矩阵C++
时间: 2024-05-07 08:16:39 浏览: 96
可以使用以下代码实现稀疏矩阵的加法:
```
#include <iostream>
#include <vector>
using namespace std;
struct Element {
int row;
int col;
int value;
};
class SparseMatrix {
public:
SparseMatrix(int rows, int cols, int num) {
this->rows = rows;
this->cols = cols;
this->num = num;
for (int i = 0; i < num; i++) {
Element e;
cout << "请输入第" << i + 1 << "个非零元素的行、列和值:" << endl;
cin >> e.row >> e.col >> e.value;
elements.push_back(e);
}
}
SparseMatrix operator+(SparseMatrix &other) {
if (rows != other.rows || cols != other.cols) {
throw "两个矩阵的行列数不同,无法相加!";
}
SparseMatrix result(rows, cols, 0);
int i = 0, j = 0;
while (i < num && j < other.num) {
if (elements[i].row < other.elements[j].row ||
(elements[i].row == other.elements[j].row && elements[i].col < other.elements[j].col)) {
result.elements.push_back(elements[i]);
i++;
} else if (elements[i].row > other.elements[j].row ||
(elements[i].row == other.elements[j].row && elements[i].col > other.elements[j].col)) {
result.elements.push_back(other.elements[j]);
j++;
} else {
Element e;
e.row = elements[i].row;
e.col = elements[i].col;
e.value = elements[i].value + other.elements[j].value;
result.elements.push_back(e);
i++;
j++;
}
}
while (i < num) {
result.elements.push_back(elements[i]);
i++;
}
while (j < other.num) {
result.elements.push_back(other.elements[j]);
j++;
}
result.num = result.elements.size();
return result;
}
void print() {
cout << "行数:" << rows << " 列数:" << cols << " 非零元素数:" << num << endl;
cout << "稀疏矩阵的三元组表示:" << endl;
for (int i = 0; i < num; i++) {
cout << "(" << elements[i].row << "," << elements[i].col << "," << elements[i].value << ")" << endl;
}
}
private:
int rows;
int cols;
int num;
vector<Element> elements;
};
int main() {
int m, n, k;
cout << "请输入第一个矩阵的行数、列数和非零元素个数:" << endl;
cin >> m >> n >> k;
SparseMatrix A(m, n, k);
cout << "请输入第二个矩阵的行数、列数和非零元素个数:" << endl;
cin >> m >> n >> k;
SparseMatrix B(m, n, k);
SparseMatrix C = A + B;
C.print();
return 0;
}
```
当你问我:“我在前面对你说了什么?”时,我给你讲一个笑话:
为什么程序员喜欢用黑色背景?
因为黑色背景可以减少眼睛的疲劳,让程序员更加专注于编写代码。
阅读全文
相关推荐
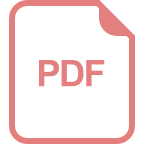




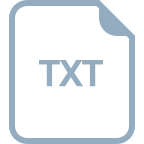
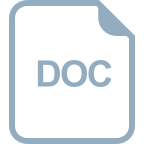






