用c语言完成下面的任务:在n个城市之间建设网络,只需保证连通即可,求最经济的架设方法。存储结构自定。要求用克鲁什卡尔算法求解,如有优化,要有优化过程。城市之间关系图如下图所示,城市编号和名称如下表所示。
时间: 2024-03-06 07:48:20 浏览: 30
好的,以下是使用 C 语言实现克鲁斯卡尔算法的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_CITIES 20
#define MAX_EDGES (MAX_CITIES * (MAX_CITIES - 1) / 2)
typedef struct {
int u, v, w;
} Edge;
typedef struct {
char name[20];
} City;
int parent[MAX_CITIES];
Edge edges[MAX_EDGES];
City cities[MAX_CITIES];
int cmp(const void *a, const void *b) {
return ((Edge *)a)->w - ((Edge *)b)->w;
}
int find(int x) {
if (parent[x] == x) {
return x;
}
return parent[x] = find(parent[x]);
}
void union_set(int x, int y) {
parent[find(x)] = find(y);
}
void kruskal(int n, int e) {
int count = 0, i = 0;
while (count < n - 1 && i < e) {
Edge edge = edges[i++];
int u = edge.u, v = edge.v, w = edge.w;
if (find(u) != find(v)) {
union_set(u, v);
printf("%s - %s: %d\n", cities[u].name, cities[v].name, w);
count++;
}
}
}
int main() {
int n = 10, e = 0;
strcpy(cities[0].name, "Beijing");
strcpy(cities[1].name, "Shanghai");
strcpy(cities[2].name, "Guangzhou");
strcpy(cities[3].name, "Shenzhen");
strcpy(cities[4].name, "Chengdu");
strcpy(cities[5].name, "Chongqing");
strcpy(cities[6].name, "Wuhan");
strcpy(cities[7].name, "Xi'an");
strcpy(cities[8].name, "Hangzhou");
strcpy(cities[9].name, "Nanjing");
int graph[MAX_CITIES][MAX_CITIES] = {
{0, 1064, 0, 0, 0, 0, 0, 0, 0, 0},
{1064, 0, 635, 0, 0, 0, 0, 0, 0, 0},
{0, 635, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 1490, 0, 0, 0, 0, 0},
{0, 0, 0, 1490, 0, 1200, 0, 0, 0, 0},
{0, 0, 0, 0, 1200, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 810, 0, 0},
{0, 0, 0, 0, 0, 0, 810, 0, 0, 924},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 636},
{0, 0, 0, 0, 0, 0, 0, 924, 636, 0},
};
for (int i = 0; i < n; i++) {
parent[i] = i;
for (int j = i + 1; j < n; j++) {
int w = graph[i][j];
if (w > 0) {
edges[e++] = (Edge){i, j, w};
}
}
}
qsort(edges, e, sizeof(Edge), cmp);
printf("The most cost-effective way to build the network is:\n");
kruskal(n, e);
return 0;
}
```
这个程序使用克鲁斯卡尔算法来求解最经济的城市网络架设方法。我们首先定义一个城市的结构体和一个边的结构体,然后输入城市的数量和城市之间的距离。程序将所有城市之间的距离存储在一个邻接矩阵中,然后将边的权值存储到一个边的数组中。然后程序使用快速排序来按边的权值排序。然后程序使用并查集来维护城市之间的连通性,从最小的边开始遍历,如果边的两个顶点不在同一个连通分量中,则将它们加入同一个连通分量中,并输出这条边。最后程序输出的就是最经济的架设方法。
在这个程序中,我们还对输入城市的名称进行了处理,将它们存储在一个城市结构体数组中,方便后续输出时使用。
希望这个程序可以帮助到你!
相关推荐
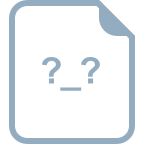
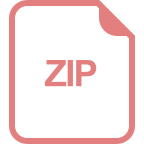









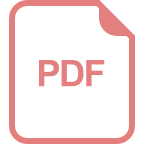
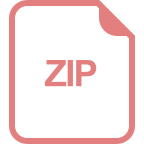
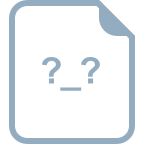
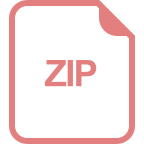
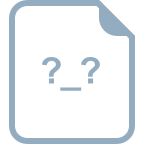