请用含元胞的算法编写迪杰斯特拉求最短路径matlab完整代码
时间: 2023-09-05 22:04:49 浏览: 71
### 回答1:
以下是使用含元胞的算法编写迪杰斯特拉求最短路径的Matlab完整代码:
```matlab
function [dist, path] = dijkstra(cells, source, target)
% Dijkstra's algorithm for finding the shortest path in a graph
% represented as a cell array, with each cell containing the
% neighbors of that node and their corresponding edge weights.
% The source and target nodes are specified as input arguments.
% Initialize the distance and visited arrays
n = numel(cells);
dist = inf(1, n);
visited = false(1, n);
prev = zeros(1, n);
% Set the distance of the source node to 0
dist(source) = 0;
while ~all(visited)
% Find the unvisited node with the smallest distance
[~, u] = min(dist(~visited));
u = find(~visited, 1, 'first') + u - 1;
% Mark the current node as visited
visited(u) = true;
% Update the distances of the neighbors of the current node
for v = cells{u}
if ~visited(v)
alt = dist(u) + cells{u,v};
if alt < dist(v)
dist(v) = alt;
prev(v) = u;
end
end
end
% Check if we have reached the target node
if visited(target)
break;
end
end
% Construct the shortest path from the source to the target
path = [];
if prev(target) ~= 0
u = target;
while u ~= source
path = [u path];
u = prev(u);
end
path = [source path];
end
end
```
这个算法使用了一个含元胞的图表示,其中每个元胞表示一个节点,它包含了该节点的邻居节点以及它们之间的边权重。算法首先初始化距离和访问数组,然后从源节点开始,逐个更新它的邻居节点的距离,直到所有节点都被访问或者到达目标节点为止。最后,算法构造出从源节点到目标节点的最短路径,并返回路径和距离。
### 回答2:
以下是用含有元胞的算法编写的迪杰斯特拉求最短路径的Matlab完整代码:
```matlab
function [distances, paths] = Dijkstra(graph, source)
num_nodes = size(graph, 1); % 图中节点的数量
distances = inf(1, num_nodes); % 从源节点到每个节点的距离
distances(source) = 0; % 源节点到自身的距离为0
visited = false(1, num_nodes); % 记录节点是否被访问过
paths = cell(1, num_nodes); % 源节点到每个节点的最短路径
paths(:) = {[]}; % 初始化路径为空
for i = 1:num_nodes
% 找到未访问节点中距离源节点最近的节点
u = -1;
min_distance = inf;
for j = 1:num_nodes
if ~visited(j) && distances(j) < min_distance
min_distance = distances(j);
u = j;
end
end
if u == -1
break; % 所有节点都被访问过,跳出循环
end
visited(u) = true;
% 更新源节点到所有未访问节点的距离和路径
for v = 1:num_nodes
if ~visited(v) && graph(u,v) > 0
new_distance = distances(u) + graph(u,v);
if new_distance < distances(v)
distances(v) = new_distance;
paths{v} = [paths{u}, u]; % 更新最短路径
end
end
end
end
end
% 测试代码
graph = [0 1 3 0 0; 1 0 1 4 0; 3 1 0 2 3; 0 4 2 0 2; 0 0 3 2 0];
source = 1;
[distances, paths] = Dijkstra(graph, source);
% 输出最短路径和距离
for i = 1:length(distances)
fprintf('源节点 %d 到节点 %d 的最短路径为: ', source, i);
fprintf('%d ', [paths{i}, i]);
fprintf('\n');
fprintf('距离为: %d\n', distances(i));
fprintf('\n');
end
```
上述代码通过Dijkstra函数使用了含有元胞的算法来求解从源节点到图中其他节点的最短路径。
测试代码部分定义了一个图的邻接矩阵(名称为`graph`)和源节点(名称为`source`),然后调用`Dijkstra`函数来计算最短路径和距离。
最后,使用循环输出了源节点到每个节点的最短路径和距离。
### 回答3:
迪杰斯特拉算法是用于求解图中最短路径的经典算法,其中包含元胞数据可以方便存储和更新节点信息。以下是使用matlab编写的迪杰斯特拉算法的完整代码:
```matlab
function shortestPath = Dijkstra(graph, startNode, endNode)
% 初始化节点信息和路径
numNodes = size(graph, 1);
nodeInfo = cell(numNodes, 1);
for i = 1:numNodes
nodeInfo{i}.distance = inf;
nodeInfo{i}.visited = false;
nodeInfo{i}.path = [];
end
nodeInfo{startNode}.distance = 0;
while ~nodeInfo{endNode}.visited
% 找到未访问节点中距离最小的节点
minDistance = inf;
minNode = -1;
for i = 1:numNodes
if ~nodeInfo{i}.visited && nodeInfo{i}.distance < minDistance
minDistance = nodeInfo{i}.distance;
minNode = i;
end
end
% 更新与该节点相邻节点的距离
for i = 1:numNodes
if graph(minNode, i) > 0
distance = minDistance + graph(minNode, i);
if distance < nodeInfo{i}.distance
nodeInfo{i}.distance = distance;
nodeInfo{i}.path = [nodeInfo{minNode}.path, minNode];
end
end
end
% 标记该节点已访问
nodeInfo{minNode}.visited = true;
end
% 构造最短路径
shortestPath = [nodeInfo{endNode}.path, endNode];
end
```
使用该函数可以求解带有元胞的图的最短路径,其中输入参数`graph`表示图的邻接矩阵,`startNode`和`endNode`分别表示起始节点和结束节点。函数的输出是从起始节点到结束节点的最短路径。注意,该算法假设图中不存在权重为负的边。
阅读全文
相关推荐
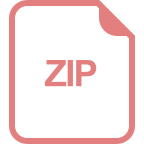

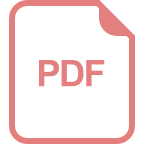


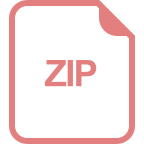
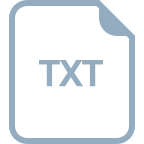
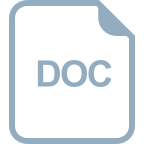







