高斯赛德尔迭代法c++
时间: 2023-10-18 20:24:42 浏览: 91
高斯赛德尔迭代法是一种求解线性方程组的迭代方法,可以用于解决大规模的线性方程组。下面是使用C++实现高斯赛德尔迭代法的示例代码:
```c++
#include <iostream>
#include <cmath>
using namespace std;
const int MAXN = 100;
const double eps = 1e-6;
int n; // 方程组的未知数个数
double a[MAXN][MAXN]; // 系数矩阵
double b[MAXN]; // 右端向量
double x[MAXN]; // 解向量
void GaussSeidel()
{
double p[MAXN]; // 存储上一次迭代的解向量
int k = 0;
// 初始化解向量
for (int i = 0; i < n; i++)
x[i] = 0;
// 迭代求解
do {
k++;
for (int i = 0; i < n; i++)
p[i] = x[i];
for (int i = 0; i < n; i++) {
double tmp = 0;
for (int j = 0; j < n; j++)
if (i != j)
tmp += a[i][j] * x[j];
x[i] = (b[i] - tmp) / a[i][i];
}
} while (k == 1 || fabs(x[0] - p[0]) > eps);
// 输出结果
cout << "Solution:" << endl;
for (int i = 0; i < n; i++)
cout << x[i] << " ";
cout << endl;
cout << "Iterations:" << k << endl;
}
int main()
{
// 读入输入
cout << "Enter the number of unknowns:";
cin >> n;
cout << "Enter the coefficient matrix:" << endl;
for (int i = 0; i < n; i++)
for (int j = 0; j < n; j++)
cin >> a[i][j];
cout << "Enter the right-hand side vector:" << endl;
for (int i = 0; i < n; i++)
cin >> b[i];
// 调用高斯赛德尔迭代法求解
GaussSeidel();
return 0;
}
```
在上面的代码中,我们首先定义了一些常量和变量,然后实现了高斯赛德尔迭代法的函数`GaussSeidel()`。在该函数中,我们首先初始化解向量`x`,然后进行迭代求解,直到解向量的变化小于预设的精度`eps`为止。最后输出求解结果和迭代次数。
在主函数中,我们读入输入,然后调用`GaussSeidel()`函数求解线性方程组。
需要注意的是,高斯赛德尔迭代法只有在系数矩阵是对角占优或严格对角占优时才能保证收敛。因此,在实际使用中需要先检查系数矩阵是否满足这个条件。
相关推荐
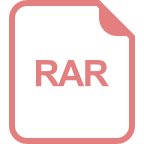











