利用随机1~9整数生成3*3的二维数组,使二维数组每行,每列、以及斜线上的数字之和均为15,并输出这个二维数组,用java实现
时间: 2023-05-31 09:06:53 浏览: 281
代码如下:
```java
import java.util.Arrays;
import java.util.Random;
public class MagicSquare {
public static void main(String[] args) {
int[][] magicSquare = generateMagicSquare();
for (int[] row : magicSquare) {
System.out.println(Arrays.toString(row));
}
}
public static int[][] generateMagicSquare() {
int[][] magicSquare = new int[3][3];
Random random = new Random();
// 先随机生成第一行,保证它的和为15
for (int i = 0; i < 3; i++) {
magicSquare[0][i] = random.nextInt(9) + 1;
}
while (magicSquare[0][0] + magicSquare[0][1] + magicSquare[0][2] != 15) {
magicSquare[0][random.nextInt(3)] = random.nextInt(9) + 1;
}
// 根据第一行推导出第二行和第三行
magicSquare[1][0] = 15 - magicSquare[0][0] - magicSquare[0][2];
magicSquare[1][1] = random.nextInt(9) + 1;
while (magicSquare[0][1] + magicSquare[1][1] + magicSquare[2][1] != 15) {
magicSquare[1][1] = random.nextInt(9) + 1;
}
magicSquare[1][2] = 15 - magicSquare[0][2] - magicSquare[1][1];
magicSquare[2][0] = 15 - magicSquare[0][1] - magicSquare[1][0];
magicSquare[2][1] = 15 - magicSquare[1][1] - magicSquare[0][1];
magicSquare[2][2] = 15 - magicSquare[0][0] - magicSquare[1][1];
return magicSquare;
}
}
```
解释一下代码实现:
首先,我们定义了一个 `generateMagicSquare` 方法,返回一个 3x3 的二维数组,用来生成魔方阵。
我们利用 `Random` 类生成随机整数来填充第一行。为了保证第一行的和为 15,我们用一个 while 循环不断调整第一行的数字,直到它们的和为 15。
接下来,我们根据第一行推导出第二行和第三行。具体来说,我们根据魔方阵的性质,第二行的中间数字一定为第一行两端数字的补数。然后我们随机生成第二行中间数字,同样用一个 while 循环不断调整它,直到第二行的第二个数字与第一列和第三列的数字之和为 15。最后,我们根据第二行推导出第三行。
最后,我们在 `main` 方法中调用 `generateMagicSquare` 方法,得到生成的魔方阵,然后遍历输出每一行。
阅读全文
相关推荐
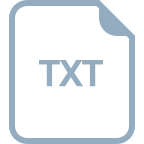
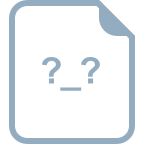














