class Mish(nn.Module): # 定义Mish激活函数的类 def __init__(self): super().__init__() def forward(self,x): x = x*(torch.tanh(F.softplus(x))) return x
时间: 2024-04-28 20:20:23 浏览: 58
这段代码是用 PyTorch 实现的 Mish 激活函数的类,Mish 激活函数的公式为:
Mish(x) = x * tanh(softplus(x))
其中,softplus(x) = log(1 + exp(x)),tanh(x) = (exp(x) - exp(-x)) / (exp(x) + exp(-x))。
这个类的 forward 方法接收一个张量 x,并将其作为输入,然后使用 PyTorch 已经实现好的 tanh 和 softplus 函数来计算 Mish 函数的值,最后返回计算结果。
相关问题
class ThreelinearModel(nn.Module): def __init__(self): super.__init__() self.linear1 = nn.Linear(12, 12) self.mish1 = Mish() self.linear2 = nn.Linear(12, 8) self.mish2 = Mish() self.linear3 = nn.Linear(8, 2) self.softmax = nn.Softmax(dim=1) self.criterion = nn.CrossEntropyLoss # 确定交叉熵系数 def forward(self,x): # 定义一个全连接网络 lin1_out = self.linear1(x) out1 = self.mish1(lin1_out) out2 = self.mish2(self.linear2(out1)) out3 = self.softmax(self.linear3(out2)) def getloss(self,x,y): y_pred = self.forward(x) loss = self.criterion(y_pred,y) return loss
这是一个使用 PyTorch 框架搭建的三层全连接神经网络模型,包括两个隐藏层和一个输出层。输入层有12个神经元,输出层有2个神经元,使用了 Mish 激活函数和 Softmax 函数,损失函数为交叉熵损失函数。该模型可以用于分类任务。在 forward 函数中,通过调用自定义的 Mish 激活函数和 Softmax 函数,将输入的 x 逐层传递到输出层,得到预测结果 y_pred。在 getloss 函数中,通过调用 forward 函数和交叉熵损失函数,计算预测值 y_pred 和真实值 y 之间的损失。
class CSPDarkNet(nn.Module):
CSPDarkNet 是一个深度神经网络模型,它是 YOLOv4 目标检测算法的基础模型之一,其核心是 CSP 模块(Cross Stage Partial Network)。它具有以下特点:
1. 使用 CSP 模块分离卷积计算,减少了计算量和参数数量。
2. 采用 DarkNet53 作为主干网络,具有较高的精度和速度。
3. 通过 SPP、PAN 等技术增强了模型的感受野和多尺度特征表达能力。
4. 基于 YOLOv4 的思想,使用 Mish 激活函数和多尺度训练等技术进一步提升了精度。
下面是 CSPDarkNet 的代码实现:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class CSPBlock(nn.Module):
def __init__(self, in_channels, out_channels, n=1, shortcut=True):
super(CSPBlock, self).__init__()
self.shortcut = shortcut
hidden_channels = out_channels // 2
self.conv1 = nn.Conv2d(in_channels, hidden_channels, 1, bias=False)
self.bn1 = nn.BatchNorm2d(hidden_channels)
self.conv2 = nn.Conv2d(in_channels, hidden_channels, 1, bias=False)
self.bn2 = nn.BatchNorm2d(hidden_channels)
self.conv3 = nn.Conv2d(hidden_channels, hidden_channels, 3, padding=1, groups=n, bias=False)
self.bn3 = nn.BatchNorm2d(hidden_channels)
self.conv4 = nn.Conv2d(hidden_channels, hidden_channels, 1, bias=False)
self.bn4 = nn.BatchNorm2d(hidden_channels)
self.conv5 = nn.Conv2d(hidden_channels, hidden_channels, 3, padding=1, groups=n, bias=False)
self.bn5 = nn.BatchNorm2d(hidden_channels)
self.conv6 = nn.Conv2d(hidden_channels, out_channels, 1, bias=False)
self.bn6 = nn.BatchNorm2d(out_channels)
self.act = nn.LeakyReLU(0.1, inplace=True)
def forward(self, x):
if self.shortcut:
shortcut = x
else:
shortcut = 0
x1 = self.conv1(x)
x1 = self.bn1(x1)
x1 = self.act(x1)
x2 = self.conv2(x)
x2 = self.bn2(x2)
x2 = self.act(x2)
x3 = self.conv3(x2)
x3 = self.bn3(x3)
x3 = self.act(x3)
x4 = self.conv4(x3)
x4 = self.bn4(x4)
x4 = self.act(x4)
x5 = self.conv5(x4)
x5 = self.bn5(x5)
x5 = self.act(x5)
x6 = self.conv6(x5)
x6 = self.bn6(x6)
x6 = self.act(x6)
out = torch.cat([x1, x6], dim=1)
return out + shortcut
class CSPDarkNet(nn.Module):
def __init__(self, num_classes=80):
super(CSPDarkNet, self).__init__()
self.stem = nn.Sequential(
nn.Conv2d(3, 32, 3, padding=1, bias=False),
nn.BatchNorm2d(32),
nn.LeakyReLU(0.1, inplace=True),
nn.Conv2d(32, 64, 3, stride=2, padding=1, bias=False),
nn.BatchNorm2d(64),
nn.LeakyReLU(0.1, inplace=True)
)
self.layer1 = nn.Sequential(
CSPBlock(64, 64, n=1, shortcut=False),
*[CSPBlock(64, 64, n=1) for _ in range(1, 3)]
)
self.layer2 = nn.Sequential(
CSPBlock(64, 128, n=2, shortcut=False),
*[CSPBlock(128, 128, n=2) for _ in range(1, 9)]
)
self.layer3 = nn.Sequential(
CSPBlock(128, 256, n=4, shortcut=False),
*[CSPBlock(256, 256, n=4) for _ in range(1, 9)]
)
self.layer4 = nn.Sequential(
CSPBlock(256, 512, n=8, shortcut=False),
*[CSPBlock(512, 512, n=8) for _ in range(1, 5)],
nn.Conv2d(512, 1024, 1, bias=False),
nn.BatchNorm2d(1024),
nn.LeakyReLU(0.1, inplace=True)
)
self.avgpool = nn.AdaptiveAvgPool2d((1, 1))
self.fc = nn.Linear(1024, num_classes)
def forward(self, x):
x = self.stem(x)
x = self.layer1(x)
x = self.layer2(x)
x = self.layer3(x)
x = self.layer4(x)
x = self.avgpool(x)
x = x.view(x.size(0), -1)
x = self.fc(x)
return x
```
阅读全文
相关推荐
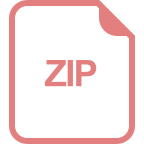
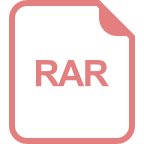
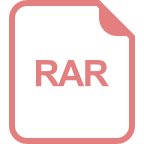






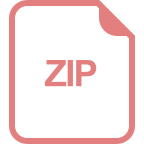
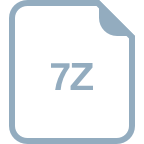
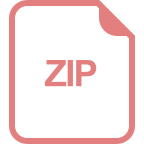
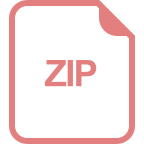
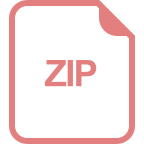
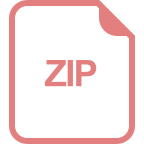
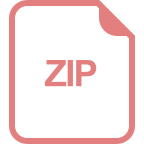