yolov7如何使用softNMS
时间: 2023-12-18 15:47:17 浏览: 41
在 YOLOv7 中使用 Soft-NMS(Soft Non-Maximum Suppression)的步骤如下:
1. 在 `detect.py` 中,增加一个 `soft_nms` 的参数,用于选择是否使用 Soft-NMS。
2. 在 `utils/general.py` 中,增加一个 `soft_nms` 函数,用于执行 Soft-NMS。
3. 在 `models/yolov7.py` 中,修改 `non_max_suppression` 函数,增加 Soft-NMS 的支持。
具体实现过程可以参考以下代码示例:
1. 在 `detect.py` 中增加 `soft_nms` 参数:
```python
parser.add_argument('--soft_nms', action='store_true', help='use Soft-NMS instead of NMS')
```
2. 在 `utils/general.py` 中增加 `soft_nms` 函数:
```python
def soft_nms(boxes, scores, sigma=0.5, thresh=0.001, method='linear'):
"""
Soft-NMS algorithm implementation.
:param boxes: bounding boxes
:param scores: confidence scores
:param sigma: Gaussian function hyperparameter
:param thresh: score threshhold for box filtering
:param method: Soft-NMS method ('linear' or 'gaussian')
:return: indices of boxes to keep after Soft-NMS
"""
N = boxes.shape[0]
indices = np.arange(N)
for i in range(N):
# get max score index
max_idx = np.argmax(scores[i:])
if i != max_idx:
# swap i-th and max_idx boxes and scores
boxes[[i, max_idx]] = boxes[[max_idx, i]]
scores[[i, max_idx]] = scores[[max_idx, i]]
indices[[i, max_idx]] = indices[[max_idx, i]]
# apply Soft-NMS
box_i = boxes[i]
score_i = scores[i]
for j in range(i+1, N):
box_j = boxes[j]
score_j = scores[j]
if iou(box_i, box_j) > thresh:
if method == 'linear':
decay = 1 - iou(box_i, box_j)
elif method == 'gaussian':
decay = np.exp(-(iou(box_i, box_j)**2) / sigma)
scores[j] = scores[j] * decay
# filter boxes by score threshold
mask = scores > thresh
indices = indices[mask]
return indices
```
3. 在 `models/yolov7.py` 中修改 `non_max_suppression` 函数:
```python
def non_max_suppression(prediction, conf_thres=0.1, iou_thres=0.6, classes=None, agnostic=False, soft_nms=False):
"""
Performs Non-Maximum Suppression (NMS) on inference results
:param prediction: tensor of shape (N, 6) containing (x1, y1, x2, y2, conf, cls) where N is the number of detections
:param conf_thres: confidence threshold
:param iou_thres: IoU threshold for NMS
:param classes: list of classes to perform NMS on
:param agnostic: boolean indicating whether to perform class-agnostic NMS
:param soft_nms: boolean indicating whether to use Soft-NMS instead of NMS
:return: list of detections with shape (x1, y1, x2, y2, conf, cls, cls_conf)
"""
# filter out boxes with low confidence
conf_mask = (prediction[:, 4] > conf_thres)
prediction = prediction[conf_mask]
if prediction.shape[0] == 0:
return []
# perform NMS
if classes is None:
classes = prediction[:, 5].astype('int').tolist()
unique_classes = list(set(classes))
detections = []
for cls in unique_classes:
mask = (prediction[:, 5] == cls)
class_pred = prediction[mask]
if agnostic:
class_mask = np.ones(class_pred.shape[0], dtype=bool)
else:
class_mask = (class_pred[:, 6] == cls)
class_pred = class_pred[class_mask]
if class_pred.shape[0] == 0:
continue
if soft_nms:
boxes = class_pred[:, :4].copy()
scores = class_pred[:, 4].copy()
keep = soft_nms(boxes, scores)
class_pred = class_pred[keep]
else:
keep = nms(class_pred[:, :4], class_pred[:, 4], iou_thres)
class_pred = class_pred[keep]
detections.extend(class_pred)
return detections
```
这样,就可以在 YOLOv7 中使用 Soft-NMS 了,只需要在运行 `detect.py` 时增加 `--soft_nms` 参数即可。
相关推荐
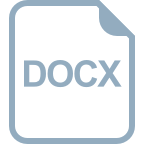
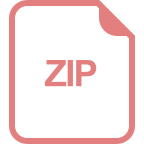














