从控制台分别输入两个关于x的多项式,其中各项系数均为整数,输入时乘号不可忽略,乘方以“^”表示,x的指数按降序排列。各操作数、运算符之间用空格分隔。输出两个多项式相乘的结果,其中x的指数按降序排列。要求:使用队列的顺序存储实现。输出代码
时间: 2023-05-25 18:06:02 浏览: 62
#include <iostream>
#include <string>
#include <queue>
using namespace std;
struct term {
int coef; // 系数
int exp; // 指数
};
int str_to_int(const string& s) { // 将字符串转换为整数
int num = 0;
for (int i = 0; i < s.length(); i++) {
num = num * 10 + s[i] - '0';
}
return num;
}
void input_poly(queue<term>& poly) { // 输入多项式
string s;
cin >> s;
while (s != "#") {
term t;
int pos = s.find("^"); // 查找指数的位置
t.coef = str_to_int(s.substr(0, pos)); // 截取系数部分并转换为整数
t.exp = str_to_int(s.substr(pos + 1)); // 截取指数部分并转换为整数
s.clear();
cin >> s;
poly.push(t); // 将项插入队尾
}
}
void add_terms(queue<term>& poly, const term& t) { // 将相同指数的项相加
term last;
if (!poly.empty()) {
last = poly.back(); // 取出队尾元素
}
if (!poly.empty() && last.exp == t.exp) { // 如果队尾项指数与当前项相同
poly.pop(); // 弹出队尾项
last.coef += t.coef; // 相加并更新系数
if (last.coef != 0) { // 如果系数不为0
poly.push(last); // 将更新后的项插入队尾
}
} else { // 否则直接将当前项加入队尾
poly.push(t);
}
}
queue<term> multiply_polys(const queue<term>& poly1, const queue<term>& poly2) { // 多项式相乘
queue<term> result;
if (poly1.empty() || poly2.empty()) { // 如果有一个多项式为空,则返回空队列
return result;
}
for (queue<term> p = poly1; !p.empty(); p.pop()) { // 遍历第一个多项式
for (queue<term> q = poly2; !q.empty(); q.pop()) { // 遍历第二个多项式
term t;
t.coef = p.front().coef * q.front().coef; // 系数相乘
t.exp = p.front().exp + q.front().exp; // 指数相加
add_terms(result, t); // 将相加后的项插入结果多项式
}
}
return result;
}
int main() {
queue<term> poly1, poly2;
cout << "Input the first polynomial (end with #): ";
input_poly(poly1);
cout << "Input the second polynomial (end with #): ";
input_poly(poly2);
queue<term> result = multiply_polys(poly1, poly2);
if (result.empty()) { // 如果结果多项式为空,则输出 “0”
cout << "0" << endl;
} else {
while (!result.empty()) {
term t = result.front();
result.pop();
if (t.coef != 0) { // 如果系数不为0,则输出这一项
if (t.exp == 0) { // 如果指数为0,则只输出系数
cout << t.coef;
} else if (t.coef == 1) { // 如果系数为1,则只输出"x"
cout << "x^" << t.exp;
} else { // 否则输出系数和"x"
cout << t.coef << "x^" << t.exp;
}
if (!result.empty() && result.front().coef > 0) { // 如果下一项系数为正,则输出加号
cout << " + ";
}
}
}
cout << endl;
}
return 0;
}
阅读全文
相关推荐

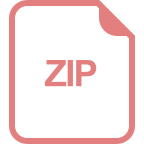
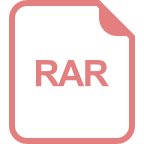
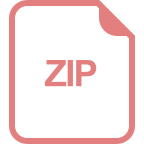
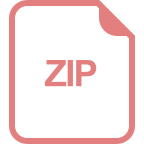
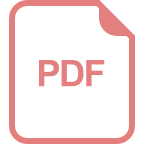
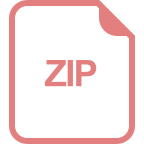
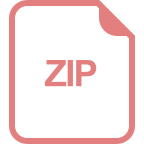
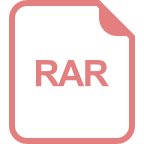
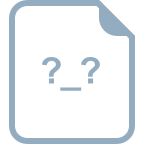
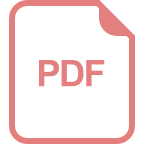
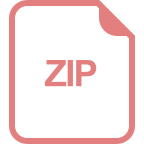