最小外接矩形cv::minAreaRect minRect的最大外接矩形Rect
时间: 2023-08-08 21:07:42 浏览: 56
最小外接矩形cv::minAreaRect返回的是一个旋转矩形(RotatedRect),包含了最小外接矩形的信息,其中包括矩形中心点坐标、宽度、高度、旋转角度等信息。如果要获取最小外接矩形的最大外接矩形,可以使用RotatedRect的boundingRect()函数,将旋转矩形转换为最大外接矩形(Rect)。
相关问题
从原图中截取cv::RotatedRect minRect,不对原图进行变换
好的,您可以使用 OpenCV 中的 cv::minAreaRect() 函数来获取一个包含轮廓的最小矩形,也就是 cv::RotatedRect 对象。这个函数的输入参数是一个二值化的图像或者是一个轮廓向量,返回的就是最小矩形。具体实现可以参考以下代码:
```c++
cv::Mat src; // 原图
cv::Mat binary; // 二值化后的图像
std::vector<std::vector<cv::Point>> contours; // 轮廓向量
cv::findContours(binary, contours, cv::RETR_EXTERNAL, cv::CHAIN_APPROX_SIMPLE); // 查找轮廓
// 获取最小矩形
cv::RotatedRect minRect = cv::minAreaRect(contours[0]); // 假设只有一个轮廓
// 在原图中绘制最小矩形
cv::Point2f vertices[4];
minRect.points(vertices);
for (int i = 0; i < 4; ++i)
cv::line(src, vertices[i], vertices[(i + 1) % 4], cv::Scalar(0, 255, 0), 2);
```
这样就可以在原图中绘制出最小矩形了,而不需要对原图进行任何变换。
opencvsharp计算最小外接矩形
在OpenCvSharp中,可以使用`MinAreaRect`方法来计算最小外接矩形。
示例代码如下:
```csharp
using OpenCvSharp;
// 读取图像
Mat srcImage = Cv2.ImRead("image.jpg");
// 转为灰度图像
Mat grayImage = new Mat();
Cv2.CvtColor(srcImage, grayImage, ColorConversionCodes.BGR2GRAY);
// 二值化图像
Mat binaryImage = new Mat();
Cv2.Threshold(grayImage, binaryImage, 0, 255, ThresholdTypes.Binary | ThresholdTypes.Otsu);
// 查找轮廓
Point[][] contours;
HierarchyIndex[] hierarchy;
Cv2.FindContours(binaryImage, out contours, out hierarchy, RetrievalModes.Tree, ContourApproximationModes.ApproxSimple);
// 计算最小外接矩形
RotatedRect minRect = Cv2.MinAreaRect(contours[0]);
// 绘制最小外接矩形
Point2f[] rectPoints = minRect.Points();
for (int i = 0; i < 4; i++)
{
Cv2.Line(srcImage, rectPoints[i], rectPoints[(i + 1) % 4], Scalar.Red, 2);
}
// 显示图像
Cv2.ImShow("srcImage", srcImage);
Cv2.WaitKey(0);
Cv2.DestroyAllWindows();
```
在这个示例中,我们首先加载图像并将其转换为灰度图像。然后,我们使用`Threshold`方法将图像二值化。接下来,我们使用`FindContours`方法查找图像中的轮廓。然后,我们使用`MinAreaRect`方法计算最小外接矩形,并使用`Line`方法绘制它。最后,我们使用`ImShow`方法显示图像,等待用户按下任意键,然后使用`DestroyAllWindows`方法关闭所有窗口。
相关推荐














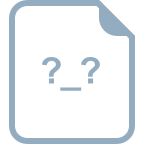