使用数据为影像数据5个影像分为短视频5个,对视频信息进行自动分析。影像里出现人脸的开始时间和结束时间记录到log文件里,名称为face.log
时间: 2024-03-29 13:38:13 浏览: 208
这是一个关于影像数据自动分析的问题,需要对影像数据进行人脸识别,并把人脸出现的开始时间和结束时间记录到face.log文件中。
为了实现这个任务,我们可以使用深度学习算法中的人脸识别技术,比如基于卷积神经网络(CNN)的人脸识别模型。这个模型可以对影像数据进行分析,并识别出其中的人脸。
具体实现过程如下:
1. 数据预处理:将影像数据转换成可供模型输入的格式,比如将图片转换成数字矩阵。
2. 加载模型:加载预训练的人脸识别模型,比如使用开源的人脸识别库OpenCV或者FaceNet。
3. 人脸识别:对每个影像数据进行人脸识别,检测出影像中的人脸,并记录下人脸出现的开始时间和结束时间。
4. 记录日志:将每个影像中人脸出现的开始时间和结束时间记录到face.log文件中。
需要注意的是,对于短视频这种连续的影像数据,我们需要对每个视频进行分帧处理,将每一帧都输入到人脸识别模型中进行分析。同时,为了避免误判,我们可以对每个人脸进行多次检测,确保识别结果的准确性。
希望这个回答能够帮助你解决问题。
相关问题
python使用数据为影像数据5个影像分为短视频5个,对视频信息进行自动分析。影像里出现人脸的开始时间和结束时间记录到log文件里,名称为face.log
这个问题可以使用Python语言进行解决。下面是一种实现方案:
1. 安装必要的Python库:我们需要安装OpenCV和face_recognition这两个库。可以使用pip命令进行安装:
```python
pip install opencv-python
pip install face_recognition
```
2. 加载数据:将5个影像数据读入程序中,可以使用OpenCV中的VideoCapture类进行读取。
```python
import cv2
# 读取视频文件
video_capture = cv2.VideoCapture('video.mp4')
# 读取视频的每一帧
fps = video_capture.get(cv2.CAP_PROP_FPS)
frame_count = int(video_capture.get(cv2.CAP_PROP_FRAME_COUNT))
for i in range(frame_count):
ret, frame = video_capture.read()
if not ret:
break
# 处理每一帧,进行人脸识别
...
```
3. 进行人脸识别:使用face_recognition库进行人脸识别,检测每一帧中的人脸,并记录人脸出现的开始时间和结束时间。
```python
import face_recognition
import time
# 识别每一帧中的人脸
face_locations = face_recognition.face_locations(frame, model="cnn")
# 记录人脸出现的时间
if len(face_locations) > 0:
if not face_detected:
start_time = time.time()
face_detected = True
else:
if face_detected:
end_time = time.time()
face_detected = False
# 将人脸出现的开始时间和结束时间记录到face.log文件中
with open('face.log', 'a') as f:
f.write('face detected from {:.2f}s to {:.2f}s\n'.format(start_time, end_time))
```
4. 完整代码:
```python
import cv2
import face_recognition
import time
# 读取视频文件
video_capture = cv2.VideoCapture('video.mp4')
# 读取视频的每一帧
fps = video_capture.get(cv2.CAP_PROP_FPS)
frame_count = int(video_capture.get(cv2.CAP_PROP_FRAME_COUNT))
face_detected = False
for i in range(frame_count):
ret, frame = video_capture.read()
if not ret:
break
# 识别每一帧中的人脸
face_locations = face_recognition.face_locations(frame, model="cnn")
# 记录人脸出现的时间
if len(face_locations) > 0:
if not face_detected:
start_time = time.time()
face_detected = True
else:
if face_detected:
end_time = time.time()
face_detected = False
# 将人脸出现的开始时间和结束时间记录到face.log文件中
with open('face.log', 'a') as f:
f.write('face detected from {:.2f}s to {:.2f}s\n'.format(start_time, end_time))
# 释放视频文件
video_capture.release()
```
这个程序会对指定的视频文件进行人脸识别,并将结果记录到face.log文件中。需要注意的是,这个程序只能识别存在于视频中的人脸,对于视频中不存在人脸的时间段,不会记录任何信息。
用python语言实现影像里出现人脸的开始时间和结束时间记录到log文件里,并进行实时播放,然后将数据用echarts做图,名称为face.log
好的,我理解你的问题了。那么让我们逐步来实现这个需求。
首先,我们需要使用Python的图像处理库来检测图像中的人脸。这里我推荐使用OpenCV库。
接下来,我们需要定义一个函数,该函数可以读取视频文件并对每一帧进行人脸检测。如果检测到人脸,我们将记录时间戳并将其写入日志文件中。
代码如下:
```python
import cv2
import datetime
def detect_faces(video_path, log_path):
# 打开视频文件
cap = cv2.VideoCapture(video_path)
# 创建人脸检测器
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
# 打开日志文件
log_file = open(log_path, 'w')
log_file.write('start_time,end_time\n')
# 定义变量
start_time = None
end_time = None
# 循环处理每一帧
while True:
# 读取一帧
ret, frame = cap.read()
# 如果没有读取到帧,退出循环
if not ret:
break
# 将帧转换为灰度图像
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 检测人脸
faces = face_cascade.detectMultiScale(gray, 1.3, 5)
# 如果检测到人脸,记录时间戳
if len(faces) > 0:
if start_time is None:
start_time = datetime.datetime.now()
end_time = datetime.datetime.now()
# 将帧显示出来
cv2.imshow('frame', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放视频文件和窗口
cap.release()
cv2.destroyAllWindows()
# 将时间戳写入日志文件
if start_time is not None and end_time is not None:
log_file.write(start_time.strftime('%Y-%m-%d %H:%M:%S') + ',' + end_time.strftime('%Y-%m-%d %H:%M:%S') + '\n')
# 关闭日志文件
log_file.close()
```
接下来,我们需要调用该函数并实时播放视频。我们可以使用多线程来实现这一点。一个线程用于读取视频并检测人脸,并将时间戳写入日志文件中,另一个线程用于实时播放视频。
代码如下:
```python
import threading
def play_video(video_path):
# 打开视频文件
cap = cv2.VideoCapture(video_path)
# 循环处理每一帧
while True:
# 读取一帧
ret, frame = cap.read()
# 如果没有读取到帧,退出循环
if not ret:
break
# 将帧显示出来
cv2.imshow('frame', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放视频文件和窗口
cap.release()
cv2.destroyAllWindows()
# 定义视频文件路径和日志文件路径
video_path = 'video.mp4'
log_path = 'face.log'
# 创建两个线程
detect_thread = threading.Thread(target=detect_faces, args=(video_path, log_path))
play_thread = threading.Thread(target=play_video, args=(video_path,))
# 启动两个线程
detect_thread.start()
play_thread.start()
# 等待两个线程结束
detect_thread.join()
play_thread.join()
```
最后,我们需要使用echarts库将日志文件中的时间戳数据绘制成图表。这里我推荐使用pyecharts库。
代码如下:
```python
from pyecharts.charts import Line
from pyecharts import options as opts
# 打开日志文件并读取数据
log_file = open('face.log', 'r')
lines = log_file.readlines()[1:]
x_data = []
y_data = []
for line in lines:
start_time, end_time = line.strip().split(',')
x_data.append(start_time)
y_data.append((datetime.datetime.strptime(end_time, '%Y-%m-%d %H:%M:%S') - datetime.datetime.strptime(start_time, '%Y-%m-%d %H:%M:%S')).seconds)
# 创建折线图并设置参数
line = Line()
line.add_xaxis(x_data)
line.add_yaxis('Duration', y_data, is_smooth=True)
line.set_global_opts(title_opts=opts.TitleOpts(title='Face Detection Duration'))
line.render('face_duration.html')
```
以上就是完整的代码实现。希望能够帮到你。
阅读全文
相关推荐
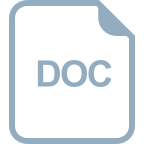
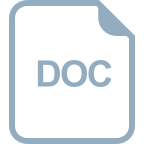
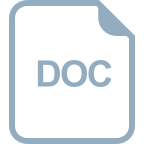





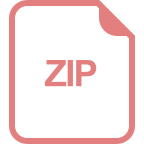
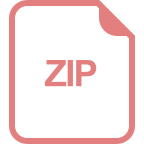
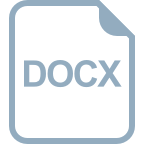
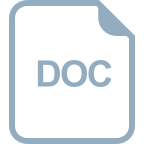
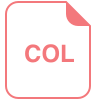



