用c语言编写,求在(-10,10)上,方程2x^3+e^x-6=0的近似解
时间: 2024-11-10 19:24:51 浏览: 10
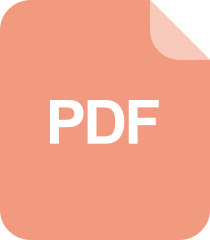
C语言:用牛顿迭代法求方程在1.5附近的根:2x^3-4x^2+3x-6=0.
在C语言中,我们可以使用数值计算方法,如牛顿迭代法或者二分查找,来寻找给定函数在特定区间内的近似解。对于高阶多项式或复杂的非线性函数,直接找到精确根可能会比较困难,因此通常会采用迭代算法。这里我们以牛顿迭代法为例:
牛顿迭代法公式如下:
\[ x_{n+1} = x_n - \frac{f(x_n)}{f'(x_n)} \]
其中 \( f(x) = 2x^3 + e^x - 6 \),\( f'(x) \) 是该函数的一阶导数。
以下是C语言的基本实现框架:
```c
#include <stdio.h>
#include <math.h>
double function(double x) {
return 2 * pow(x, 3) + exp(x) - 6;
}
double derivative(double x) {
return 6 * x * x + exp(x);
}
double newton_raphson(double initial_guess, double tolerance, int max_iterations) {
double current = initial_guess;
for (int i = 0; i < max_iterations && abs(function(current)) > tolerance; ++i) {
current -= function(current) / derivative(current);
}
return current;
}
int main() {
double initial_guess = 0.0; // 初始猜测点
double tolerance = 1e-6; // 精度要求
int max_iterations = 100; // 最大迭代次数
double root = newton_raphson(initial_guess, tolerance, max_iterations);
printf("The approximate root of the equation within (-10, 10) is %.6lf\n", root);
return 0;
}
```
注意,这个例子假设方程在给定区间内有实数解,并且牛顿迭代法收敛。实际上,你需要验证这一点并可能需要调整初始猜测、精度和最大迭代次数。
阅读全文
相关推荐
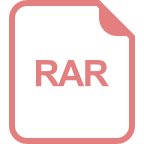
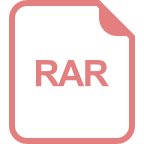















