给出c++的SPFA算法模板
时间: 2023-12-06 14:40:40 浏览: 81
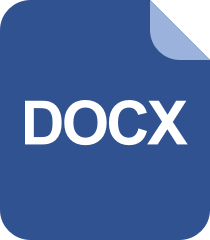
c++ SPFA算法
以下是C++中的SPFA(Shortest Path Faster Algorithm)算法的基本模板:
```cpp
#include <iostream>
#include <vector>
#include <queue>
#include <limits>
const int INF = std::numeric_limits<int>::max(); // 无穷大值
struct Edge {
int to;
int weight;
};
void addEdge(std::vector<std::vector<Edge>>& graph, int from, int to, int weight) {
graph[from].push_back({to, weight});
}
void spfa(std::vector<std::vector<Edge>>& graph, std::vector<int>& dist, int start) {
int n = graph.size();
dist[start] = 0;
std::vector<bool> inQueue(n, false);
std::queue<int> q;
q.push(start);
inQueue[start] = true;
while (!q.empty()) {
int node = q.front();
q.pop();
inQueue[node] = false;
for (const auto& edge : graph[node]) {
int to = edge.to;
int weight = edge.weight;
if (dist[node] + weight < dist[to]) {
dist[to] = dist[node] + weight;
if (!inQueue[to]) {
q.push(to);
inQueue[to] = true;
}
}
}
}
}
int main() {
int n = 6; // 节点数量
int start = 0; // 起始节点
std::vector<std::vector<Edge>> graph(n);
std::vector<int> dist(n, INF);
// 添加边
addEdge(graph, 0, 1, 2);
addEdge(graph, 0, 2, 5);
addEdge(graph, 1, 2, 1);
addEdge(graph, 1, 3, 6);
addEdge(graph, 2, 3, 2);
addEdge(graph, 2, 4, 3);
addEdge(graph, 3, 4, 1);
addEdge(graph, 3, 5, 4);
addEdge(graph, 4, 5, 2);
spfa(graph, dist, start);
std::cout << "最短路径长度:" << std::endl;
for (int i = 0; i < n; ++i) {
std::cout << "从起点 " << start << " 到节点 " << i << ": " << dist[i] << std::endl;
}
return 0;
}
```
在上面的模板中,我们首先定义了一个`Edge`结构体,表示图的边。然后,我们实现了`addEdge`函数来添加边到图中。接下来,我们定义了`spfa`函数来执行SPFA算法。最后,在`main`函数中,我们创建了一个图,并调用`spfa`函数来计算最短路径。最终,我们打印出从起点到每个节点的最短路径长度。
请注意,这只是SPFA算法的基本模板,具体的实现和使用可能会根据实际情况有所不同。
阅读全文
相关推荐
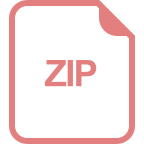
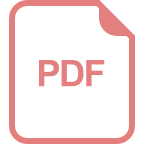
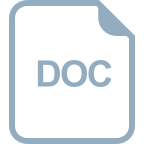
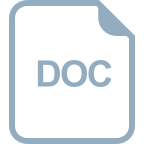
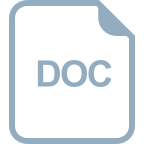


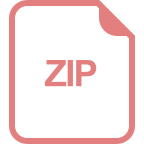
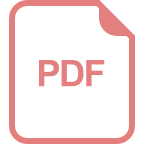
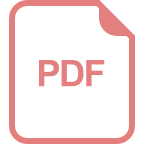
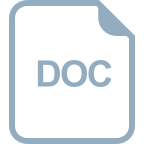
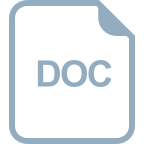
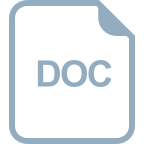
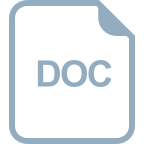
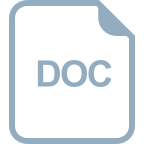
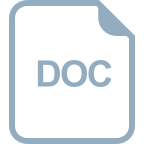
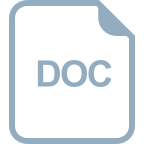