python多线程同时读取四个摄像头并简单拼接成一幅图像显示
时间: 2023-09-16 12:09:02 浏览: 190
在Python中,你可以使用OpenCV库和多线程来实现同时读取四个摄像头并简单拼接成一幅图像显示。下面是一个示例代码,展示了如何使用多线程并发读取四个摄像头的视频流,并将它们简单拼接成一幅图像显示出来:
```python
import cv2
import threading
# 摄像头索引
camera_indices = [0, 1, 2, 3]
# 读取视频流的线程类
class CameraThread(threading.Thread):
def __init__(self, camera_index):
threading.Thread.__init__(self)
self.camera_index = camera_index
self.video_capture = cv2.VideoCapture(camera_index)
self.frame = None
self.running = True
def run(self):
while self.running:
ret, frame = self.video_capture.read()
if ret:
self.frame = frame
def stop(self):
self.running = False
self.join()
# 创建多个摄像头线程并启动
camera_threads = []
for index in camera_indices:
thread = CameraThread(index)
thread.start()
camera_threads.append(thread)
while True:
# 获取每个摄像头线程的最新帧
frames = [thread.frame for thread in camera_threads]
# 拼接图像
top_row = cv2.hconcat([frames[0], frames[1]])
bottom_row = cv2.hconcat([frames[2], frames[3]])
combined_frame = cv2.vconcat([top_row, bottom_row])
# 显示拼接后的图像
cv2.imshow("Combined Frame", combined_frame)
# 按下 'q' 键退出循环
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 停止并释放摄像头线程和资源
for thread in camera_threads:
thread.stop()
thread.video_capture.release()
cv2.destroyAllWindows()
```
在上面的示例中,我们定义了一个`CameraThread`类,用于每个摄像头创建一个线程,并在后台并发读取视频帧。主循环中,我们通过遍历每个摄像头线程,获取其最新的视频帧。然后,我们使用`cv2.hconcat()`和`cv2.vconcat()`函数将四个摄像头的视频帧进行拼接,并在窗口中显示拼接后的图像。
请确保你已经正确安装了OpenCV库,并根据需要修改代码中的摄像头索引。此外,你还可以根据需要对拼接后的图像进行进一步处理或调整。
请注意,多线程并发读取摄像头的视频流可能会对系统资源产生一定的压力。如果遇到性能问题,你可以考虑减少并发读取的摄像头数量或者使用更高性能的设备。
阅读全文
相关推荐


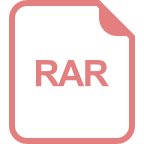
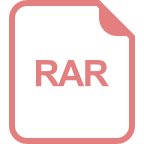
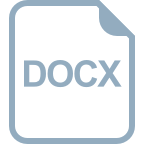
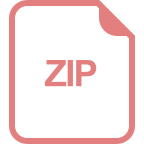
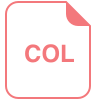
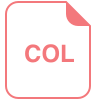
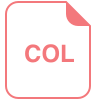
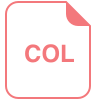
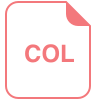
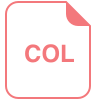
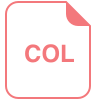
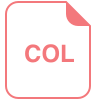
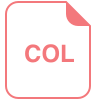
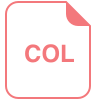