牛顿迭代法解非线性方程组python代码
时间: 2024-09-27 15:00:46 浏览: 52
牛顿迭代法是一种常用的数值优化方法,用于求解非线性方程组的根。在Python中,我们可以使用该算法来逐步逼近方程组的解。这里是一个基本的牛顿迭代法的实现示例:
```python
import numpy as np
def newton_raphson(funcs, x0, Jacobian, tol=1e-6, max_iter=100):
"""
Newton-Raphson method for solving a system of nonlinear equations.
Args:
- funcs (list of functions): List of functions that represent the equations f_i(x) = 0.
- x0 (numpy array): Initial guess for the solution.
- Jacobian (function): Function that returns the Jacobian matrix of the functions with respect to x.
- tol (float): Tolerance for convergence (default 1e-6).
- max_iter (int): Maximum number of iterations (default 100).
Returns:
- numpy array: Approximate solution after convergence or max_iter reached.
"""
x = x0.copy()
for _ in range(max_iter):
J = Jacobian(x)
delta_x = np.linalg.solve(J, -funcs(x)) # Update using the inverse Jacobian
if np.all(np.abs(delta_x) < tol):
break # Converged
x += delta_x
else:
print("Warning: Max iterations reached without convergence.")
return x
# Example usage:
# Define your functions f_1 and f_2
def func1(x):
return x[0]**2 + x[1] - 1
def func2(x):
return x[0] + x[1]**2 - 2
# Jacobian matrix calculation
def jacobian(x):
dfdx = np.array([[2*x[0], 1], [1, 2*x[1]]])
return dfdx
# Initial guess
x0 = np.array([1, 1])
# Solve the system
solution = newton_raphson([func1, func2], x0, jacobian)
solution,
阅读全文
相关推荐
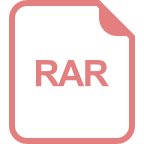
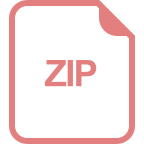





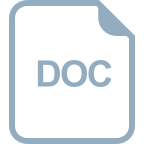



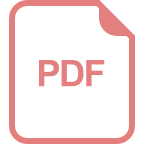
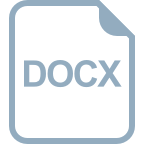




