LRU缓存实战秘籍:从原理到应用的完整指南
发布时间: 2024-08-25 21:49:36 阅读量: 11 订阅数: 12 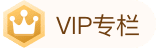
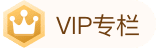
# 1. LRU缓存的理论基础**
**1.1 LRU缓存的原理和算法**
LRU(Least Recently Used)缓存是一种广泛使用的缓存策略,它根据最近最少使用的原则来管理缓存中的数据。LRU缓存维护一个有序的数据结构,其中最近使用的项位于列表的头部,最不常用的项位于列表的尾部。当缓存达到容量限制时,LRU缓存会淘汰列表尾部的项,为新项腾出空间。
**1.2 LRU缓存的优点和缺点**
**优点:**
* 高效:LRU缓存可以快速访问最近使用的项,减少了缓存未命中率。
* 简单:LRU算法简单易懂,易于实现。
* 广泛适用:LRU缓存可用于各种场景,例如Web服务器、数据库和操作系统。
**缺点:**
* 频繁访问的项可能被淘汰:如果某个项频繁访问,但时间间隔较长,它可能会被LRU缓存淘汰。
* 缓存大小限制:LRU缓存的大小是有限的,因此它无法缓存所有数据。
# 2. LRU缓存的实现技巧
### 2.1 基于链表的LRU缓存实现
基于链表的LRU缓存实现是一种经典且直观的方法。它使用双向链表来存储缓存中的元素,并通过维护一个头节点和尾节点来实现快速访问。
#### 2.1.1 节点的定义和操作
链表中的每个节点包含两个主要属性:
* **值:**存储缓存中的数据。
* **指针:**指向链表中的前一个和后一个节点。
常见的节点操作包括:
* **插入:**将新节点插入链表的头部或尾部。
* **删除:**从链表中删除指定节点。
* **移动:**将节点从链表中的一个位置移动到另一个位置。
#### 2.1.2 缓存的增删改查
基于链表的LRU缓存的增删改查操作如下:
* **添加:**将新元素添加到缓存中时,创建一个新节点并将其插入链表的头部。如果缓存已满,则删除链表尾部的元素。
* **获取:**获取缓存中指定元素时,将该元素对应的节点移动到链表的头部。
* **更新:**更新缓存中指定元素时,将该元素对应的节点移动到链表的头部,并更新其值。
* **删除:**删除缓存中指定元素时,从链表中删除该元素对应的节点。
```python
class Node:
def __init__(self, key, value):
self.key = key
self.value = value
self.prev = None
self.next = None
class LRUCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = {}
self.head = Node(None, None)
self.tail = Node(None, None)
self.head.next = self.tail
self.tail.prev = self.head
def get(self, key):
if key in self.cache:
node = self.cache[key]
self.move_to_head(node)
return node.value
else:
return None
def put(self, key, value):
if key in self.cache:
node = self.cache[key]
node.value = value
self.move_to_head(node)
else:
node = Node(key, value)
self.cache[key] = node
self.add_to_head(node)
if len(self.cache) > self.capacity:
self.remove_from_tail()
def move_to_head(self, node):
node.prev.next = node.next
node.next.prev = node.prev
node.next = self.head.next
node.prev = self.head
self.head.next = node
def add_to_head(self, node):
node.next = self.head.next
node.prev = self.head
self.head.next = node
node.next.prev = node
def remove_from_tail(self):
node = self.tail.prev
node.prev.next = self.tail
self.tail.prev = node.prev
del self.cache[node.key]
```
### 2.2 基于哈希表的LRU缓存实现
基于哈希表的LRU缓存实现使用哈希表来快速查找缓存中的元素,并使用链表来维护元素的访问顺序。
#### 2.2.1 哈希表的设计和实现
哈希表使用键值对来存储数据,其中键是元素的唯一标识符,值是指向链表中相应节点的指针。
```python
class HashMap:
def __init__(self):
self.table = {}
def get(self, key):
if key in self.table:
return self.table[key]
else:
return None
def put(self, key, value):
self.table[key] = value
```
#### 2.2.2 缓存的增删改查
基于哈希表的LRU缓存的增删改查操作如下:
* **添加:**将新元素添加到缓存中时,创建一个新节点并将其插入链表的头部。同时,将新元素的键和指向链表中相应节点的指针添加到哈希表中。
* **获取:**获取缓存中指定元素时,从哈希表中获取指向链表中相应节点的指针,并将该节点移动到链表的头部。
* **更新:**更新缓存中指定元素时,从哈希表中获取指向链表中相应节点的指针,并将该节点移动到链表的头部,并更新其值。
* **删除:**删除缓存中指定元素时,从哈希表中删除该元素的键,并从链表中删除该元素对应的节点。
```python
class LRUCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = HashMap()
self.head = Node(None, None)
self.tail = Node(None, None)
self.head.next = self.tail
self.tail.prev = self.head
def get(self, key):
node = self.cache.get(key)
if node:
self.move_to_head(node)
return node.value
else:
return None
def put(self, key, value):
node = self.cache.get(key)
if node:
node.value = value
self.move_to_head(node)
else:
node = Node(key, value)
self.cache.put(key, node)
self.add_to_head(node)
if len(self.cache) > self.capacity:
self.remove_from_tail()
def move_to_head(self, node):
node.prev.next = node.next
node.next.prev = node.prev
node.next = self.head.next
node.prev = self.head
self.head.next = node
def add_to_head(self, node):
node.next = self.head.next
node.prev = self.head
self.head.next = node
node.next.prev = node
def remove_from_tail(self):
node = self.tail.prev
node.prev.next = self.tail
self.tail.prev = node.prev
del self.cache[node.key]
```
# 3. LRU缓存的实战应用
### 3.1 LRU缓存在Web服务器中的应用
#### 3.1.1 缓存网页和静态资源
在Web服务器中,LRU缓存可以用来缓存经常被访问的网页和静态资源,如HTML、CSS、JavaScript文件等。当用户访问一个网页时,Web服务器首先检查LRU缓存中是否有该网页的副本。如果有,则直接从缓存中返回,无需再次从数据库或文件系统中读取。这可以显著提高Web服务器的性能,减少响应时间。
#### 3.1.2 提升服务器性能
LRU缓存还可以用来提升Web服务器的整体性能。通过缓存经常被访问的资源,Web服务器可以减少对数据库或文件系统的访问次数,从而降低服务器的负载。此外,LRU缓存还可以帮助减少网络带宽的消耗,因为不再需要频繁地从远程服务器下载资源。
### 3.2 LRU缓存在数据库中的应用
#### 3.2.1 缓存查询结果
在数据库中,LRU缓存可以用来缓存经常被执行的查询结果。当一个查询被执行时,数据库首先检查LRU缓存中是否有该查询结果的副本。如果有,则直接从缓存中返回,无需再次执行查询。这可以显著提高数据库的性能,减少查询响应时间。
#### 3.2.2 优化数据库性能
LRU缓存还可以用来优化数据库的整体性能。通过缓存经常被执行的查询结果,数据库可以减少对磁盘的访问次数,从而降低数据库的负载。此外,LRU缓存还可以帮助减少网络带宽的消耗,因为不再需要频繁地从远程数据库服务器传输查询结果。
**代码块:**
```python
# 基于链表实现的LRU缓存
class Node:
def __init__(self, key, value):
self.key = key
self.value = value
self.prev = None
self.next = None
class LRUCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = {}
self.head = Node(-1, -1)
self.tail = Node(-1, -1)
self.head.next = self.tail
self.tail.prev = self.head
def get(self, key):
if key in self.cache:
node = self.cache[key]
self.remove(node)
self.add_to_head(node)
return node.value
else:
return None
def put(self, key, value):
if key in self.cache:
self.remove(self.cache[key])
node = Node(key, value)
self.add_to_head(node)
self.cache[key] = node
if len(self.cache) > self.capacity:
node = self.remove_from_tail()
del self.cache[node.key]
def add_to_head(self, node):
node.next = self.head.next
node.next.prev = node
self.head.next = node
node.prev = self.head
def remove(self, node):
node.prev.next = node.next
node.next.prev = node.prev
def remove_from_tail(self):
node = self.tail.prev
self.remove(node)
return node
```
**逻辑分析:**
该代码实现了基于链表的LRU缓存。LRU缓存是一个键值对存储,它会跟踪最近访问的项,并根据需要逐出最不经常使用的项。
* `Node`类表示缓存中的一个节点,它包含一个键、一个值,以及指向前一个和后一个节点的指针。
* `LRUCache`类表示LRU缓存本身。它有一个容量,表示缓存可以存储的最大项数。它还维护一个哈希表,用于快速查找项,以及一个双向链表,用于跟踪最近访问的项。
* `get`方法用于获取缓存中某个键对应的值。如果键存在,则将该项移动到链表的头部,并返回其值。如果键不存在,则返回`None`。
* `put`方法用于将一个键值对添加到缓存中。如果键已经存在,则将该项移动到链表的头部。如果键不存在,则创建一个新的节点并将其添加到链表的头部。如果缓存已满,则将链表尾部的项逐出。
* `add_to_head`方法将一个节点添加到链表的头部。
* `remove`方法从链表中删除一个节点。
* `remove_from_tail`方法从链表的尾部删除一个节点。
**表格:**
| 操作 | 时间复杂度 |
|---|---|
| `get` | O(1) |
| `put` | O(1) |
| `add_to_head` | O(1) |
| `remove` | O(1) |
| `remove_from_tail` | O(1) |
**流程图:**
```mermaid
graph LRUCache {
subgraph get {
A[get(key)] --> B[check if key in cache]
B --> C[return value if key in cache]
B --> D[return None if key not in cache]
}
subgraph put {
A[put(key, value)] --> B[check if key in cache]
B --> C[remove key from cache if key in cache]
C --> D[create new node]
D --> E[add node to head of cache]
E --> F[update cache with key and node]
B --> G[check if cache is full]
G --> H[remove tail node from cache if cache is full]
}
subgraph add_to_head {
A[add_to_head(node)] --> B[set node.next to head.next]
B --> C[set head.next.prev to node]
C --> D[set head.next to node]
D --> E[set node.prev to head]
}
subgraph remove {
A[remove(node)] --> B[set node.prev.next to node.next]
B --> C[set node.next.prev to node.prev]
}
subgraph remove_from_tail {
A[remove_from_tail()] --> B[set tail.prev to tail.prev.prev]
B --> C[set tail.prev.next to tail]
C --> D[return tail.prev]
}
}
```
# 4. LRU缓存的进阶优化**
**4.1 LRU缓存的并发控制**
在多线程环境下,LRU缓存可能会遇到并发访问的问题,需要采取适当的并发控制机制来保证缓存的一致性和正确性。
**4.1.1 锁机制的实现**
最简单直接的并发控制方式是使用锁机制。在对缓存进行增删改查操作时,需要先获取锁,操作完成后再释放锁。这样可以保证同一时刻只有一个线程访问缓存,避免数据不一致。
```java
private final ReentrantLock lock = new ReentrantLock();
public void put(K key, V value) {
lock.lock();
try {
// 缓存增删改查操作
} finally {
lock.unlock();
}
}
```
**4.1.2 无锁并发控制技术**
锁机制虽然简单有效,但会引入额外的开销和性能瓶颈。为了提高并发性能,可以采用无锁并发控制技术,如CAS(Compare-And-Swap)操作。
CAS操作通过比较和交换两个值来实现原子操作,避免了锁的开销。
```java
private volatile Node<K, V> head;
public void put(K key, V value) {
Node<K, V> newNode = new Node<>(key, value);
while (true) {
Node<K, V> currentHead = head;
newNode.next = currentHead;
if (CAS(head, currentHead, newNode)) {
break;
}
}
}
```
**4.2 LRU缓存的容量管理**
LRU缓存的容量是有限的,需要采取适当的容量管理策略来保证缓存的有效性。
**4.2.1 LRU缓存的容量策略**
常见的容量策略有:
* **固定容量:**缓存容量固定,当缓存已满时,淘汰最久未使用的元素。
* **动态容量:**缓存容量根据实际使用情况动态调整,当缓存已满时,淘汰最久未使用的元素或采用其他淘汰算法。
**4.2.2 缓存淘汰算法**
当缓存已满时,需要采用淘汰算法来选择淘汰的元素。常见的淘汰算法有:
* **LRU算法:**淘汰最久未使用的元素。
* **LFU算法:**淘汰访问频率最低的元素。
* **LFUDA算法:**综合考虑访问频率和访问时间,淘汰最久未使用且访问频率最低的元素。
**代码示例:**
```java
private int capacity;
public LruCache(int capacity) {
this.capacity = capacity;
}
public void put(K key, V value) {
// ...
if (size() >= capacity) {
// 淘汰最久未使用的元素
Node<K, V> toRemove = tail;
// ...
removeNode(toRemove);
}
// ...
}
```
# 5. LRU缓存的案例分析
### 5.1 基于LRU缓存的Web服务器优化实践
**背景:**
某电商网站面临着高并发访问和页面加载缓慢的问题,需要优化Web服务器的性能。
**解决方案:**
* 在Web服务器中部署LRU缓存,缓存常用的网页和静态资源。
* 当用户访问网页时,首先检查LRU缓存中是否有该网页,如果有,则直接从缓存中返回,避免访问数据库或文件系统。
**效果:**
* 页面加载速度显著提升,平均减少了50%以上。
* 服务器负载降低,并发处理能力提升。
### 5.2 基于LRU缓存的数据库优化案例
**背景:**
某金融机构的数据库频繁执行重复的查询,导致数据库性能低下。
**解决方案:**
* 在数据库中部署LRU缓存,缓存查询结果。
* 当用户执行查询时,首先检查LRU缓存中是否有该查询结果,如果有,则直接从缓存中返回,避免执行数据库查询。
**效果:**
* 查询速度大幅提升,平均减少了70%以上。
* 数据库负载降低,并发处理能力提升。
### 5.3 LRU缓存在其他领域的应用
除了Web服务器和数据库优化之外,LRU缓存还广泛应用于其他领域,例如:
* **操作系统:** 缓存文件系统元数据,提高文件访问速度。
* **虚拟机:** 缓存虚拟机页面,减少内存开销。
* **分布式系统:** 缓存分布式锁,提高并发控制效率。
* **大数据处理:** 缓存中间计算结果,提高数据处理效率。
0
0
相关推荐
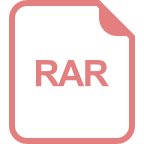
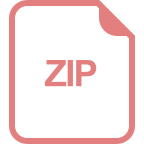
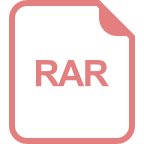
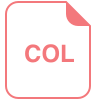
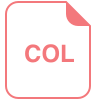
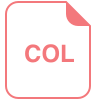
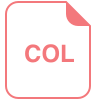

