决策树剪枝技术精进指南
发布时间: 2024-09-04 10:42:59 阅读量: 75 订阅数: 39 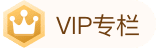
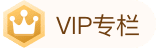
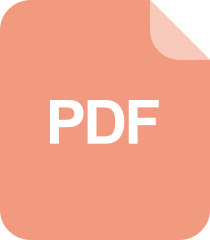
决策树剪枝算法的python实现方法详解

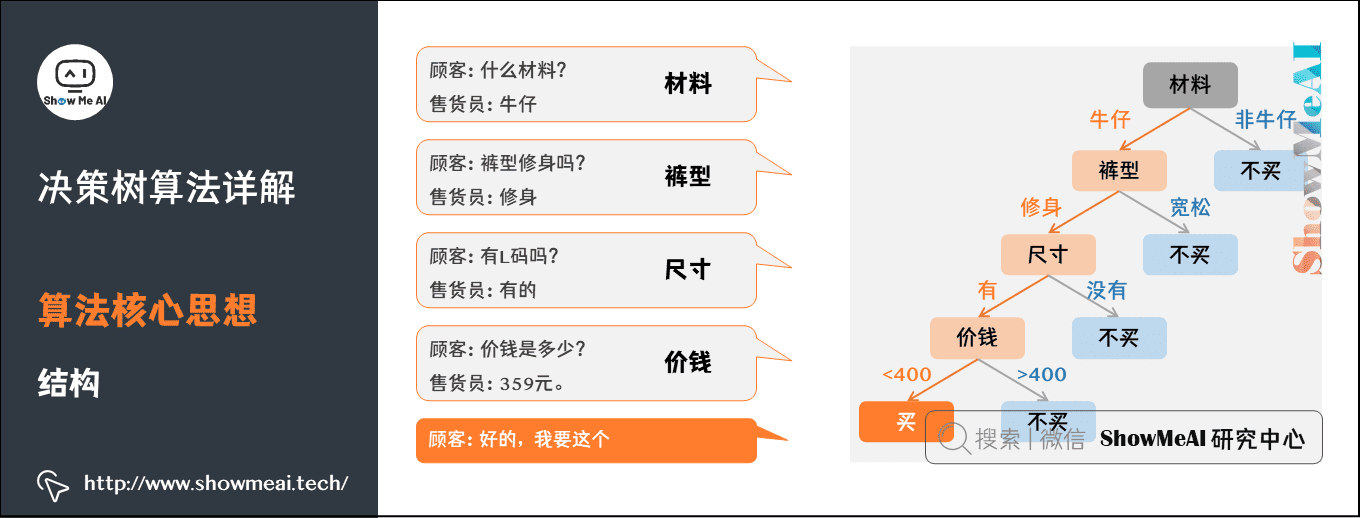
# 1. 决策树剪枝技术概述
在机器学习领域,决策树是一种广泛应用于分类和回归问题的监督学习算法。然而,不加控制的模型复杂度往往会导致过拟合现象,即模型在训练数据上表现良好,而在未见数据上表现较差。为了解决这一问题,决策树剪枝技术应运而生。剪枝是一种防止过拟合、简化决策树模型的常用方法,通过对决策树的分支进行修剪,以提高模型的泛化能力。本章将介绍决策树剪枝技术的基本概念、常见剪枝方法以及剪枝在实际应用中的重要性。接下来的章节将深入探讨剪枝技术的理论基础、实践应用及高级应用场景,为理解剪枝技术提供全面的视角。
# 2. 理论基础与剪枝方法论
## 2.1 决策树的构建过程
### 2.1.1 信息增益与基尼不纯度
决策树的构建过程是选择最佳特征,并根据这个特征对数据进行分割的过程。其中,信息增益和基尼不纯度是衡量分割好坏的两种常用标准。
信息增益的概念来源于信息论,它度量了在知道某个特征的信息后,数据集的不确定性减少了多少。具体计算方法如下:
- 首先计算数据集的熵,熵的公式为:
\[ H(D) = -\sum_{k=1}^{K} p_k \log_2 p_k \]
其中 \( H(D) \) 是数据集 \( D \) 的熵,\( p_k \) 是数据集中第 \( k \) 个类别出现的概率。
- 然后计算每个特征的信息增益,公式为:
\[ IG(D,a) = H(D) - \sum_{t=1}^{T} \frac{|D_t|}{|D|} H(D_t) \]
其中 \( IG(D,a) \) 是特征 \( a \) 的信息增益,\( D_t \) 是按照特征 \( a \) 的取值划分的数据集。
基尼不纯度是另一种衡量数据集纯度的方式,它的取值范围是从0(纯度最高)到1(纯度最低),公式为:
\[ Gini(D) = 1 - \sum_{k=1}^{K} p_k^2 \]
其中 \( Gini(D) \) 是数据集 \( D \) 的基尼不纯度。
在决策树中,通常选择使数据集信息增益最大或者基尼不纯度最小的特征作为节点特征。
### 2.1.2 决策树的递归划分机制
一旦选定最佳特征,决策树就会根据这个特征的取值递归地对数据集进行划分,创建节点和子节点。这个递归划分的过程一直进行,直到满足停止条件,比如:
- 每个子节点中的数据都属于同一个类别。
- 没有更多的特征可以用来分割。
- 节点中的数据量小于预设的阈值。
- 树的深度达到了预设的最大值。
通过这种递归划分机制,决策树逐步建立起来,每个内部节点代表一个特征的测试,每个分支代表测试的结果,而每个叶节点代表最终的决策或者类别。
### 示例代码
以下是使用Python中的`scikit-learn`库实现决策树构建过程的一个简单示例:
```python
from sklearn.tree import DecisionTreeClassifier
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# 加载数据集
iris = load_iris()
X = iris.data
y = iris.target
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=1)
# 创建决策树模型并训练
clf = DecisionTreeClassifier(criterion='entropy') # 使用信息增益
clf.fit(X_train, y_train)
# 预测测试集
y_pred = clf.predict(X_test)
# 计算准确率
print(f'Accuracy: {accuracy_score(y_test, y_pred):.2f}')
```
在这段代码中,我们使用了Iris数据集,并通过`train_test_split`函数将其分为训练集和测试集。接着创建了一个`DecisionTreeClassifier`的实例,并指定了使用信息增益(`criterion='entropy'`)作为决策树的划分标准。最后,我们训练了模型并对其在测试集上的表现进行了评估。
### 参数说明
- `DecisionTreeClassifier`: scikit-learn库中的决策树分类器。
- `criterion='entropy'`: 设置决策树的划分标准为信息增益。
- `train_test_split`: 用于划分数据集,`test_size=0.3`表示测试集占总数据集的30%。
- `accuracy_score`: 用于计算模型预测的准确率。
## 2.2 剪枝技术的理论框架
### 2.2.1 过拟合与剪枝的必要性
在构建决策树的过程中,如果不对树的复杂度进行控制,很容易造成过拟合(overfitting)。过拟合是指模型在训练数据上表现很好,但在未见过的数据上表现较差。这通常是由于模型过于复杂,捕捉到了训练数据中的噪声和异常值,而没有学到数据的真实分布。
剪枝技术因此被引入,其目的是减少决策树的复杂度,提升模型的泛化能力。剪枝可以分为预剪枝和后剪枝两种:
- **预剪枝(Pre-pruning)**:在决策树构建的过程中,通过提前停止树的增长来防止过拟合。例如,可以设定最小分裂样本数,当节点中的样本数量小于这个阈值时停止进一步分裂。
- **后剪枝(Post-pruning)**:先构建一棵完整的树,然后从叶节点开始,自底向上地删除那些对模型输出影响最小的节点,直到达到特定的性能标准。
### 2.2.2 剪枝策略的分类:预剪枝与后剪枝
预剪枝和后剪枝的策略选择会直接影响决策树模型的性能和泛化能力。
预剪枝通常基于以下原则进行:
- 限制树的最大深度。
- 强制提前终止节点的分裂。
- 设置最小子节点样本数。
- 限制叶节点中的最小样本数。
后剪枝则基于如下原则:
- 评估每个节点的错误降低量。
- 移除只导致模型性能轻微下降的节点。
- 通过交叉验证来确定最佳剪枝水平。
- 利用验证集的误差来选择剪枝的节点。
预剪枝策略相对简单,易于实现,但有可能因过早停止而剪掉了太多有用的节点。后剪枝虽然计算成本更高,但更灵活,并且在剪枝后通常能得到更好的模型性能。
### 示例代码
下面展示了一个使用预剪枝的决策树构建示例:
```python
from sklearn.tree import DecisionTreeClassifier
# 创建决策树模型并应用预剪枝
clf_prepruned = DecisionTreeClassifier(max_depth=3, min_samples_split=10)
clf_prepruned.fit(X_train, y_train)
# 预测测试集
y_pred_prepruned = clf_prepruned.predict(X_test)
# 计算准确率
print(f'Pre-Pruned Accuracy: {accuracy_score(y_test, y_pred_prepruned):.2f}')
```
在这个例子中,我们限制了决策树的最大深度为3,并设置了`min_samples_split=10`,即每个节点至少需要有10个样本才能进行分割。这样就应用了预剪枝策略。
而应用后剪枝策略的例子为:
```python
from sklearn.tree import DecisionTreeClassifier, export_graphviz
from sklearn.model_selection import cross_val_score
# 创建后剪枝决策树模型
clf_postpruned = DecisionTreeClassifier ccp_alpha=0.01)
clf_postpruned.fit(X_train, y_train)
# 使用交叉验证来评估模型表现
scores = cross_val_score(clf_postpruned, X, y, cv=5)
print(f'Mean Accuracy: {scores.mean():.2f}')
```
在这段代码中,我们通过设置`ccp_alpha`参数为正值来启用后剪枝策略。`ccp_alpha`值越大,剪枝效果越明显,模型复杂度越低。
### 参数说明
- `max_depth`: 决策树的最大深度,用于预剪枝。
- `min_samples_split`: 内部节点再划分所需的最小样本数,用于预剪枝。
- `ccp_alpha`: 后剪枝参数,用于控制树复杂度与模型错误率之间的平衡,正值表示后剪枝。
- `cross_val_score`: 用于交叉验证,评估模型的平均准确率。
## 2.3 剪枝算法详解
### 2.3.1 错误复杂度剪枝(Cost Complexity Pruning)
错误复杂度剪枝是后剪枝中一种重要的算法,它基于对每个节点引入一个成本复杂度(cost complexity)的概念。具体来说,每个节点的成本复杂度是其误分类错误数与该节点的叶子节点数的乘积。
公式如下:
\[ CC_{\alpha}(T) = E(T) + \alpha |T| \]
其中,\( CC_{\alpha}(T) \) 是节点 \( T \) 的成本复杂度,\( E(T) \) 是节点 \( T \) 的错误率,\( |T| \) 是节点 \( T \) 的叶子节点数,\( \alpha \) 是正则化参数,控制对剪枝的惩罚力度。
通过递归地删除成本复杂度最大的节点,直到满足剪枝停止条件,算法可以找到在特定 \( \alpha \) 下的最优子树。
### 2.3.2 最小错误剪枝(Minimal Error Pruning)
最小错误剪枝算法的目标是寻找一个子树,它在保留下来的验证集上具有最
0
0
相关推荐
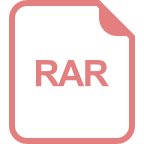
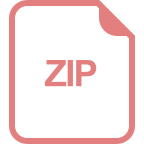





