Case Analysis of YOLOv8 Applications in the Industrial Field: Intelligent Monitoring and Identification Technology Applications
发布时间: 2024-09-14 00:56:45 阅读量: 22 订阅数: 21 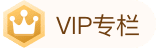
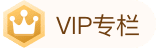
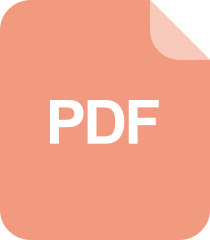
High-dimensional sensitivity analysis of complex optronic systems by experimental design: applications to the case of the design and the robustness of optical coatings
# Analysis of YOLOv8 Application Cases in the Industrial Sector: Intelligent Monitoring and Recognition Technology
## 2.1 YOLOv8 Algorithm Principles
### 2.1.1 Network Structure
YOLOv8 employs a novel network structure known as CSPDarknet53. This structure is based on Darknet53 but includes the following improvements:
- **CSP (Cross-Stage Partial) Structure:** The network is divided into multiple stages, each containing several convolutional layers and a residual connection. This structure enhances the network's feature extraction capabilities and robustness.
- **Mish Activation Function:** The traditional ReLU activation function is replaced with the Mish activation function. Mish has smoother gradients and stronger non-linearity, improving the network's training stability and convergence speed.
- **PAN (Path Aggregation Network):** Jump connections are added between shallow and deep feature maps in the network. These connections merge features at different scales, enhancing the network's detection accuracy and generalization ability.
### 2.1.2 Loss Function
YOLOv8 uses a new loss function called CIOU Loss (Complete Intersection over Union Loss). This loss function combines IOU Loss and DIOU Loss to better measure the overlap between predicted and actual bounding boxes, thus improving the network's positioning accuracy.
# 2. Theoretical Application of YOLOv8 in the Industrial Sector
### 2.1 YOLOv8 Algorithm Principles
#### 2.1.1 Network Structure
YOLOv8 utilizes a novel network architecture called Cross-Stage Partial Connections (CSP). The CSP structure divides the feature maps into multiple stages, each containing several convolutional layers. The output of each stage is partially connected to the input of the next stage, forming a skip connection. This architecture effectively merges features from different stages, enhancing the network's feature extraction capabilities.
```python
import torch
from torch import nn
class CSPDarknet(nn.Module):
def __init__(self, in_channels, out_channels, n=1):
super(CSPDarknet, self).__init__()
self.conv1 = nn.Conv2d(in_channels, out_channels, 1, stride=1, padding=0, bias=False)
self.conv2 = nn.Conv2d(in_channels, out_channels // 2, 1, stride=1, padding=0, bias=False)
self.conv3 = nn.Conv2d(out_channels // 2, out_channels // 2, 3, stride=1, padding=1, bias=False)
self.conv4 = nn.Conv2d(out_channels // 2, out_channels // 2, 1, stride=1, padding=0, bias=False)
self.conv5 = nn.Conv2d(out_channels // 2, out_channels, 3, stride=1, padding=1, bias=False)
self.csp_block = nn.Sequential(
nn.Conv2d(out_channels, out_channels // 2, 1, stride=1, padding=0, bias=False),
nn.Conv2d(out_channels // 2, out_channels // 2, 3, stride=1, padding=1, bias=False),
nn.Conv2d(out_channels // 2, out_channels // 2, 1, stride=1, padding=0, bias=False),
nn.Conv2d(out_channels // 2, out_channels, 3, stride=1, padding=1, bias=False),
)
self.shortcut = nn.Sequential(
nn.Conv2d(in_channels, out_channels, 1, stride=1, padding=0, bias=False),
nn.BatchNorm2d(out_channels),
)
self.bn = nn.BatchNorm2d(out_channels)
self.activation = nn.LeakyReLU(0.1)
def forward(self, x):
x1 = self.conv1(x)
x2 = self.conv2(x)
x2 = self.conv3(x2)
x2 = self.conv4(x2)
x2 = self.conv5(x2)
x3 = self.csp_block(x2)
x4 = self.shortcut(x)
x = self.bn(x3 + x4)
x = self.activation(x)
return x
```
#### 2.1.2 Loss Function
YOLOv8 uses a new loss function called Complete IoU Loss (CIOU Loss). CIOU Loss adds two new terms to the traditional IoU Loss: a distance penalty term and an aspect ratio penalty term. The distance penalty term penalizes the distance between the predicted and actual bounding boxes, while the aspect ratio penalty term penalizes the difference in aspect ratio between the predicted and actual bounding boxes.
```python
import torch
from torch import nn
class CIOULoss(nn.Module):
def __init__(self, reduction='mean'):
super(CIOULoss, self).__init__()
self.reduction = reduction
def forward(self, pred, target):
# Calculate IoU
iou = torch.clamp(torch.min(pred[:, :, :, 0:2], target[:, :, :, 0:2]) /
torch.max(pred[:, :, :, 0:2], target[:, :, :, 0:2]), min=0, max=1)
# Calculate center point distance
dist = torch.sum(torch.pow(pred[:, :, :, 2:4] - target[:, :, :, 2:4], 2), dim=3)
# Calculate aspect ratio
pred_wh = pred[:, :, :, 2:4]
target_wh = target[:, :, :, 2:4]
ar = torch.min(pred_wh[:, :, :, 0] / pred_wh[:, :, :, 1], target_wh[:, :, :, 0] / target_wh[:, :, :, 1])
ar_loss = 4 / (math.pi ** 2) * (torch.atan(ar) - torch.atan(pred_wh[:, :, :, 0] / pred_wh[:, :, :, 1])) ** 2
#
```
0
0
相关推荐
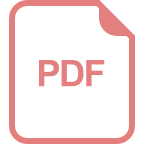
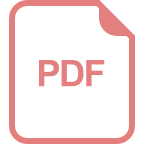
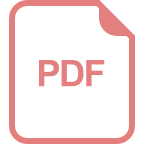
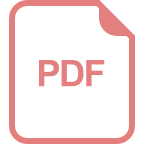
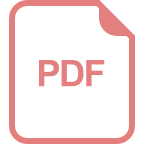
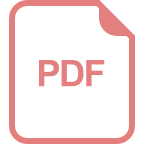
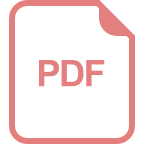
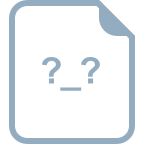
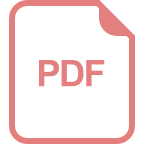