YOLOv8 Applications in Smart City Construction: Technological Innovations in Urban Management and Environmental Monitoring
发布时间: 2024-09-14 01:11:05 阅读量: 31 订阅数: 21 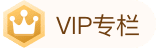
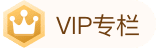
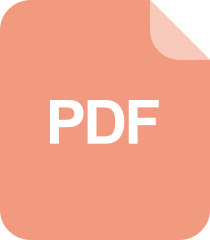
Smart City and Green Growth
# YOLOv8 Application in Smart City Construction: Technological Innovations in Urban Management and Environmental Monitoring
## 1. Overview of YOLOv8
YOLOv8 is a real-time object detection algorithm released in 2022, known for its outstanding performance and speed. It is based on the YOLOv7 architecture and incorporates several improvements, including:
- **Bag-of-Freebies (BoF)**: A series of verified training techniques that improve model accuracy and speed.
- **Deep Supervision**: Supervision loss added at different stages of the network to enhance feature learning.
- **Path Aggregation Network (PAN)**: A feature fusion module used to aggregate features of different scales.
- **Spatial Attention Module (SAM)**: A spatial attention mechanism to highlight target areas.
These improvements enable YOLOv8 to achieve an AP (Average Precision) of 76.8% on the COCO dataset while maintaining an inference speed of up to 160 FPS. The combination of speed and accuracy makes YOLOv8 an ideal choice for applications such as urban management, environmental monitoring, and smart city construction.
## 2. Application of YOLOv8 in Urban Management
### 2.1 Analysis of Crowd Density
#### 2.1.1 Crowd Counting and Distribution Analysis
An important application of YOLOv8 in urban management is the analysis of crowd density, including crowd counting and distribution analysis. By deploying cameras in key urban areas, the YOLOv8 model can detect and count crowds in real-time and analyze their distribution patterns.
```python
import cv2
import numpy as np
# Load the YOLOv8 model
net = cv2.dnn.readNet("yolov8.weights", "yolov8.cfg")
# Initialize video stream
cap = cv2.VideoCapture("city_street.mp4")
while True:
# Read a frame
ret, frame = cap.read()
if not ret:
break
# Preprocess the frame
blob = cv2.dnn.blobFromImage(frame, 1/255.0, (640, 640), (0,0,0), swapRB=True, crop=False)
# Set the input to the model
net.setInput(blob)
# Forward propagation
detections = net.forward()
# Post-process the detection results
for detection in detections:
# Get the detection box and confidence
x, y, w, h, conf = detection[0:5]
# Filter out low-confidence detections
if conf > 0.5:
# Calculate the center point and size of the detection box
cx, cy = x + w/2, y + h/2
width, height = w, h
# Draw the detection box and label
cv2.rectangle(frame, (int(cx - width/2), int(cy - height/2)), (int(cx + width/2), int(cy + height/2)), (0, 255, 0), 2)
cv2.putText(frame, "Person", (int(cx), int(cy - 10)), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# Display the frame
cv2.imshow("Crowd Density Analysis", frame)
# Exit by pressing 'q'
if cv2.waitKey(1) & 0xFF == ord("q"):
break
# Release resources
cap.release()
cv2.destroyAllWindows()
```
**Code Logic Analysis:**
1. Load the YOLOv8 model.
2. Initialize video stream.
3. Loop to read frames.
4. Preprocess the frame into the format required by the YOLOv8 model.
5. Set the model input.
6. Forward propagation to obtain detection results.
7. Post-process detection results, filtering out low-confidence detections.
8. Calculate the center point and size of the detection box.
9. Draw the detection box and label.
10. Display the frame.
11. Exit by pressing 'q'.
12. Release resources.
**Parameter Description:**
* `yolov8.weights`: Path to the YOLOv8 model weight file.
* `yolov8.cfg`: Path to the YOLOv8 model configuration file.
* `city_street.mp4`: Input video file path.
* `0.5`: Detection confidence threshold.
#### 2.1.2 Crowd Flow Monitoring and Abnormal Behavior Detection
In addition to crowd counting and distribution analysis, YOLOv8 can also be used for crowd flow monitoring and abnormal behavior recognition. By analyzing the movement patterns of crowds, YOLOv8 can identify abnormal behaviors such as crowding, stampedes, or violent incidents.
```python
import cv2
import numpy as np
# Load the YOLOv8 model
net = cv2.dnn.readNet("yolov8.weights", "yolov8.cfg")
# Initialize video stream
cap = cv2.VideoCapture("city_street.mp4")
# Initialize background subtractor
bg_subtractor = cv2.createBackgroundSubtractorMOG2()
while True:
# Read a frame
ret, frame = cap.read()
if not ret:
break
# Preprocess the frame
blob = cv2.dnn.blobFromImage(frame, 1/255.0, (640, 640), (0,0,0), swapRB=True, crop=False)
# Set the model input
net.setInput(blob)
# Forward propagation
detections = net.forward()
# Post-process the detection results
for detection in detections:
# Get the detection box and confidence
x, y, w, h, conf = detection[0:5]
# Filter out low-confidence detections
if conf > 0.5:
# Calculate the center point and size of the detection box
cx, cy = x + w/2, y + h/2
width, height = w, h
# Draw the detection box and label
cv2.rectangle(frame, (int(cx - width/2), int(cy - height/2)), (int(cx + width/2), int(cy + height/2)), (0, 255, 0), 2)
cv2.putText(frame, "Person", (int(cx), int(cy - 10)), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# Background subtraction
fg_mask = bg_subtractor.apply(frame)
# Morphological operations of dilation and erosion
kernel = np.ones((5,5),np.uint8)
fg_mask = cv2.dilate(fg_mask, kernel, iterations=2)
fg_mask = cv2.erode(fg_mask, kernel, iterations=2)
# Find contours
contours, _ = cv2.findContours(fg_mask, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# Analyze contours
for contour in contours:
# Calculate the area of the contour
area = cv2.contourArea(contour)
# Filter out small area contours
if area < 1000:
continue
# Calculate the bounding rectangle of the contour
x, y, w, h = cv2.boundingRect(contour)
# Draw the bounding rectangle and label
cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 0, 255), 2)
cv2.putText(frame, "Abnormal Behavior", (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 0, 255), 2)
# Display the frame
cv2.imshow("Crowd Flow Monitoring and Abnormal Behavior Detection", frame)
# Exit by pressing 'q'
if cv2.waitKey(1) & 0xFF == ord("q"):
break
# Release resources
cap.release()
cv2.destroyAllWindows()
```
**Code Logic Analysis:**
1. Load the YOLOv8 model.
2. Initialize video stream.
3. Initialize background subtractor.
4. Loop to read frames.
5. Preprocess the frame into the format required by the YOLOv8 model.
6. Set the model input.
7. Forward propagation to obtain detection results.
8. Post-process detection results, filtering out low-confidence detections.
9. Calculate the center point and size of the detection box.
10. Draw the detection box and label.
11. Perform background subtraction.
12. Apply morphological operations.
13. Find contours.
14. Analyze contours, filtering out small area contours.
15. Calculate the bounding rectangle of the contour.
16. Draw the bounding rectangle and label.
17. Display the frame.
18. Exit by pressing 'q'.
19. Release resources.
**Parameter Description:**
* `yolov8.weights`: Path to the YOLOv8 model weight file.
* `yolov8.cfg`: Path to the YOLOv8 model configuration file.
* `city_street.mp4`: Input video file path.
* `1000`: Area threshold for filtering small area contours.
## ***r Quality Monitoring with YOLOv8
### 3.1 Air Pollution Source Identification
The application of YOLOv8 in air pollution source identification is mainly reflected in the real-time monitoring of pollution sources such as chi
0
0