堆结构与优先队列:Java中的高效实现与应用场景
发布时间: 2024-09-10 23:52:16 阅读量: 9 订阅数: 14 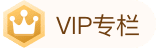
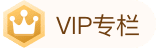
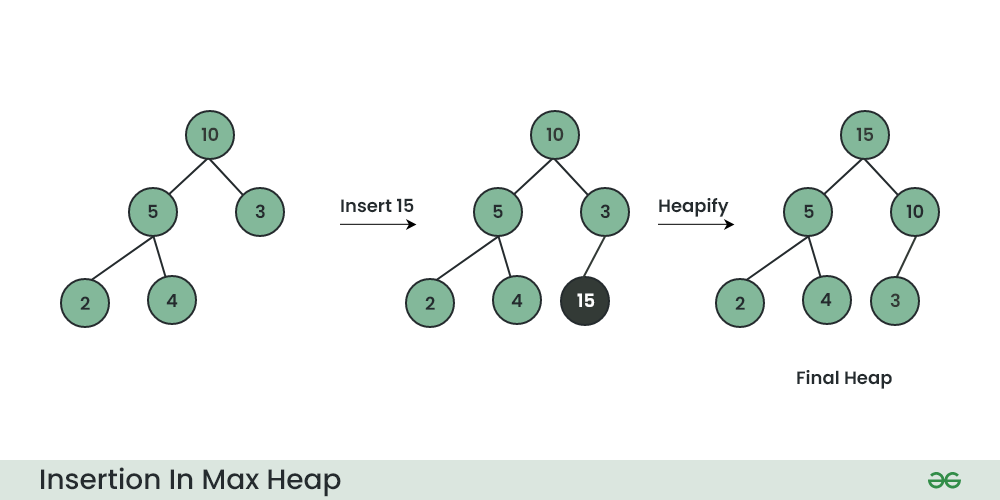
# 1. 堆结构的基本概念与原理
在计算机科学中,堆是一种特殊的树形数据结构,通常称为二叉堆。堆结构允许用户以满足堆属性的方式存储一组元素,使得能够高效地访问到集合中的“最大元素”或“最小元素”。堆的两个最常见的类型是最大堆和最小堆。在最大堆中,每个父节点的值都大于或等于其子节点的值,从而保证堆顶元素是集合中的最大值;而在最小堆中,则是每个父节点的值都小于或等于其子节点的值,保证堆顶元素是最小的。
## 堆的性质
堆结构具有以下重要性质:
- 形状性质:一个堆是一棵完全二叉树,这意味着所有的层次都是完全填满的,除了可能的最后一层,且该层的所有节点都尽可能地靠左排列。
- 堆性质:任何一个父节点的值都必须大于或等于(在最大堆中)或小于或等于(在最小堆中)其子节点的值。
## 堆的操作
堆结构支持一系列基本操作,主要包括:
- **插入(insert)**:在堆的末尾添加一个元素,然后通过上浮(或称为堆化)操作来恢复堆的性质。
- **删除堆顶(deleteMax或deleteMin)**:移除并返回堆顶元素,然后用堆中的最后一个元素替换堆顶,之后通过下沉(或称为堆化)操作恢复堆的性质。
- **查找堆顶(findMax或findMin)**:返回堆中的最大元素或最小元素,而不需要从堆中移除它。
这些操作的时间复杂度通常是对数级别的,O(log n),其中n是堆中元素的数量。这是因为堆是一种高度平衡的树结构,任何对堆性质的破坏都可以通过有限次数的元素交换来修复。由于其高度优化的操作,堆结构被广泛用于许多算法中,特别是在优先队列和排序算法中。
# 2. 优先队列的Java实现
优先队列是计算机科学中一个非常重要的数据结构,尤其在处理具有优先级的任务调度、事件驱动编程等领域有着广泛的应用。Java中的优先队列是一种基于优先级堆的无界队列,可用于实现任务调度器、事件队列等。这一章将详细介绍优先队列的内部机制、堆结构在优先队列中的应用以及优先队列的自定义扩展。
### 2.1 Java中优先队列的内部机制
#### 2.1.1 优先队列的数据结构基础
在Java中,优先队列是通过完全二叉堆实现的。堆是一种特殊的树形数据结构,每个节点都满足堆属性。在二叉堆中,任意一个非叶子节点的值都大于或等于其子节点的值(大顶堆),或者小于或等于其子节点的值(小顶堆)。
```java
class PriorityQueue<E> extends AbstractQueue<E>
implements java.io.Serializable {
// ... 实现细节 ...
}
```
优先队列允许插入任意类型的对象,并通过对象的自然顺序来管理这些对象。如果需要处理不同类型的对象,则可以使用`PriorityQueue`的构造函数,提供一个自定义的比较器(`Comparator`)来确定对象的优先级顺序。
#### 2.1.2 优先队列的操作与性能
Java的`PriorityQueue`提供了`offer()`、`poll()`、`peek()`等核心操作:
- `offer(E e)`:将元素插入到优先队列中。如果插入成功,则返回`true`。
- `poll()`:获取并移除队列头部的元素(即优先级最高的元素)。如果队列为空,则返回`null`。
- `peek()`:获取但不移除队列头部的元素。如果队列为空,则返回`null`。
优先队列的时间复杂度主要取决于堆的维护,插入和移除操作的时间复杂度为O(log n),其中n为堆中元素的数量。因此,优先队列在处理大量数据时仍然能够保持较好的性能。
### 2.2 堆结构在优先队列中的应用
#### 2.2.1 堆的构建过程与算法
堆的构建过程是优先队列初始化的基础,可以利用插入排序的方式构建堆。从最后一个非叶子节点开始,向上逐个调整节点以满足堆属性,这个过程称为上浮或堆化(heapify)。
```java
private void siftUpUsingComparator(int k, E x) {
while (k > 0) {
int parent = (k - 1) >>> 1;
Object e = queue[parent];
if (***pare(x, (E)e) >= 0)
break;
queue[k] = e;
k = parent;
}
queue[k] = x;
}
```
该代码段是优先队列中用于上浮的实现,通过比较器确保堆属性得以维持。
#### 2.2.2 堆的调整方法与优化技巧
当优先队列中的元素发生变化时,需要调整堆结构以维护优先级。这个过程通常涉及元素的上浮(siftUp)或下沉(siftDown)。下沉操作是从堆顶开始,逐层向下调整,确保每个节点都小于其子节点。
```java
private void siftDownUsingComparator(int k, E x) {
int half = size >>> 1;
while (k < half) {
Object c = queue[k];
int child = (k << 1) + 1;
Object swap = queue[child];
if (child + 1 < size && ***pare((E)swap, (E)queue[child + 1]) > 0)
swap = queue[++child];
if (***pare(x, (E)swap) <= 0)
break;
queue[k] = swap;
k = child;
}
queue[k] = x;
}
```
这段代码表示当需要下沉时的处理逻辑,确保下沉后的子树仍然满足堆的性质。
堆的优化通常关注堆调整的效率。Java的实现通过减少比较次数和提升数据局部性来提高性能,例如使用跳表结构或内存池技术来减少堆中节点的移动。
### 2.3 优先队列的自定义扩展
#### 2.3.1 自定义比较器实现复杂排序规则
在某些场景下,标准的自然顺序可能不足以满足优先级排序的需求。通过实现`Comparator`接口,可以定义任意复杂的优先级规则。
```java
PriorityQueue<Item> pq = new PriorityQueue<>(new Comparator<Item>() {
public int compare(Item i1, Item i2) {
// 自定义比较逻辑
}
});
```
在Java 8之后,可以利用lambda表达式简化代码:
```java
PriorityQueue<Item> pq = new PriorityQueue<>((i1, i2) -> {
// 自定义比较逻辑
});
```
这种方式使得优先队列的使用更加灵活,可以适应各种复杂的业务场景。
#### 2.3.2 集合框架中的优先队列应用
Java集合框架中的`PriorityQueue`并不支持随机访问操作,如`get(int index)`或`set(int index, E element)`。但优先队列在实现如定时任务等场景时,提供了非常便捷的机制。
例如,可以使用`ScheduledExecutorService`来实现定时任务:
```java
ScheduledExecutorService executor = Executors.newScheduledThreadPool(1);
executor.schedule(new RunnableTask(), delayTime, TimeUnit.SECONDS);
```
在这个例子中,任务将被放入优先队列中,并按照预定的延迟执行。优先队列根据任务的调度时间顺序进行排序,从而确保按时执行。
在下一章节,我们将深入探讨堆结构与优先队列在算法中的应用,以及它们如何解决复杂的算法问题。
# 3. 堆结构与优先队列在算法中的应用
堆结构不仅仅在数据结构中扮演着重要角色,它在算法设计和实际问题求解中也具有举足轻重的地位。本章节将深入探讨堆结构和优先队列在算法设计中的多样化应用,从基本的排序算法到复杂的图算法,再到优先队列与其他算法的结合。
## 3.1 堆排序算法详解
### 3.1.1 堆排序的基本原理
堆排序是一种选择排序,通过堆这种数据结构所设计的一种排序算法。堆是一种近似完全二叉树的结构,并同时满足堆积的性质:即子节点的键值或索引总是小于(或者大于)它的父节点。在堆结构中,最大的元素总是位于树的顶端,这个特性被称为堆性质。
堆排序算法包括两个主要步骤:
1. 构建一个堆结构。
2. 重复移除堆顶元素,并将其与堆的最后一个元素交换,然后调整剩余元素为新的堆。
这种排序算法的时间复杂度为O(nlogn),适合在内存受限的情况下使用。
```java
import java.util.Arrays;
public class HeapSort {
public void sort(int[] array) {
int n = array.length;
// 构建最大堆
for (int i = n / 2 - 1; i >= 0; i--) {
heapify(array, n, i);
}
// 一个个从堆顶取出元素
for (int i = n - 1; i >= 0; i--) {
// 将当前最大的元素移动到数组末尾
int temp = array[0
```
0
0
相关推荐
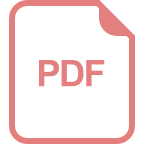
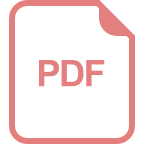
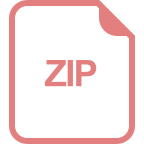





