fmincon Parallel Optimization: An Effective Method for Accelerating the Solution Process
发布时间: 2024-09-14 11:39:15 阅读量: 43 订阅数: 28 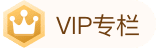
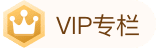
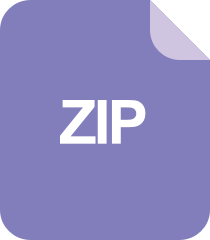
minJT.zip_The Method Method_matlab_minJT_optimal_design
# 1. An Introduction to fmincon Optimization
fmincon is a powerful function in MATLAB designed for solving constrained optimization problems. It employs the Sequential Quadratic Programming (SQP) algorithm, an iterative method that approximates the optimal solution by solving quadratic subproblems at each iteration. fmincon is capable of handling various types of constraints, including linear, nonlinear, bound, and integer constraints.
The syntax for the fmincon function is as follows:
```
x = fmincon(fun,x0,A,b,Aeq,beq,lb,ub,nonlcon,options)
```
Where:
* `fun`: Objective function
* `x0`: Initial guess
* `A`, `b`: Linear inequality constraints
* `Aeq`, `beq`: Linear equality constraints
* `lb`, `ub`: Bound constraints
* `nonlcon`: Nonlinear constraints
* `options`: Optimization options
# 2. Parallel Optimization Techniques
### 2.1 Principles and Advantages of Parallel Computing
Parallel computing is a technology that utilizes multiple processing units to perform tasks simultaneously, thereby improving computational efficiency. It achieves this by breaking down tasks into smaller subtasks and executing them in parallel on different processing units.
The advantages of parallel computing include:
***Increased computational speed**: By using multiple processing units simultaneously, parallel computing can significantly increase computational speed, especially when dealing with large-scale data or complex calculations.
***Shortened solution time**: Parallel computing can reduce solution time because multiple processing units can handle different parts simultaneously, reducing the total computation time.
***Higher resource utilization**: Parallel computing can improve resource utilization as it allows multiple processing units to work at the same time rather than idling.
### 2.2 Implementation Methods for fmincon Parallel Optimization
fmincon is a function in MATLAB for nonlinear constrained optimization. It supports parallel optimization, which can be implemented in two ways:
#### 2.2.1 Multicore Parallelism
Multicore parallelism utilizes the multicore CPU of a computer for parallel computing. It achieves this by breaking down tasks into multiple threads and executing these threads in parallel on different CPU cores.
```matlab
% Set up a parallel pool
parpool(4); % Use 4 CPU cores
% Define the optimization problem
fun = @(x) x(1)^2 + x(2)^2;
x0 = [0, 0];
lb = [-1, -1];
ub = [1, 1];
% Parallel optimization
options = optimoptions('fmincon', 'Display', 'iter', 'UseParallel', true);
[x, fval] = fmincon(fun, x0, [], [], [], [], lb, ub, [], options);
```
**Code Logic Analysis:**
* `parpool(4)`: Sets up a parallel pool with 4 worker processes.
* `fmincon`: Enables parallel optimization using the `UseParallel` option.
* The `Display` option is set to `iter` to display optimization progress after each iteration.
#### 2.2.2 GPU Parallelism
GPU parallelism utilizes the computer's Graphics Processing Unit (GPU) for parallel computing. The GPU has a large number of parallel processing units, making it well-suited for handling large-scale data and complex computations.
```matlab
% Set up a parallel pool
gpuDevice(1); % Use the first GPU
% Define the optimization problem
fun = @(x) x(1)^2 + x(2)^2;
x0 = [0, 0];
lb = [-1, -1];
ub = [1, 1];
% Parallel optimization
options = optimoptions('fmincon', 'Display', 'iter', 'Algorithm', 'interior-point', 'UseParallel', true);
[x, fval] = fmincon(fun, x0, [], [], [], [], lb, ub, [], options);
```
**Code Logic Analysis:**
* `gpuDevice(1)`: Selects and enables the first GPU.
* `fmincon`: Enables parallel optimization using the `UseParallel` option.
* The `Algorithm` option is set to `interior-point` as this algorithm supports GPU parallelism.
* The `Display` option is set to `iter` to display optimization progress after each iteration.
# 3. fmincon Parallel Optimization in Practice
### 3.1 Selection of Parallel Optimization Algorithms
When selecting a parallel optimization algorithm, consider the following factors:
- **Problem Type**: fmincon supports various types of optimization problems, including unconstrained optimization, constrained optimization, and nonlinear least squares problems. Different algorithms may be more effective for different types of optimization problems.
- **Parallelism Degree**: The degree of parallelism determines how many parallel threads an algorithm can use simultaneously. The higher the parallelism degree, the better the potential acceleration effect.
- **Code Complexity**: Some algorithms are more complex than others, which can affect the difficulty of coding and debugging.
fmincon supports multiple parallel optimization algorithms, including:
| Algorithm | Parallelism Degree | Code Complexity |
|---|
0
0
相关推荐








