fmincon Memory Consumption Optimization: Strategies to Reduce Memory Footprint
发布时间: 2024-09-14 11:42:49 阅读量: 9 订阅数: 18 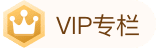
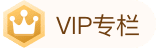
# 1. Overview of fmincon Memory Consumption
fmincon is a MATLAB function used to solve nonlinear constrained optimization problems. It employs internal algorithms to find the optimal solution that satisfies the constraints. However, when dealing with large-scale or complex problems, fmincon can consume a significant amount of memory, which can affect its performance and stability.
This guide delves into the memory consumption characteristics of fmincon, analyzes the factors causing high memory usage, and provides a series of optimization strategies to effectively reduce memory consumption. By optimizing fmincon's memory usage, users can significantly improve their solving efficiency and reliability, achieving better results when solving large and complex optimization problems.
# 2. Memory Consumption Optimization Strategies
## 2.1 Reducing Variable Count
### 2.1.1 Identifying and Eliminating Redundant Variables
Redundant variables are those that appear multiple times during the optimization process but whose values can be calculated from other variables. Identifying and eliminating redundant variables can substantially reduce memory consumption.
**Operational Steps:**
1. Analyze the optimization model to identify correlated variables.
2. Determine whether any variables can be computed from others.
3. Remove redundant variables and update the model to use the calculated variables.
**Code Example:**
```matlab
% Original Model
x = optimvar('x', 3);
y = optimvar('y', 3);
f = x(1) + x(2) + x(3) + y(1) + y(2) + y(3);
% Optimized Model
x = optimvar('x', 3);
y = optimvar('y', 2);
f = x(1) + x(2) + x(3) + y(1) + y(2);
```
**Logical Analysis:**
In the original model, variables `x(3)` and `y(3)` are redundant since their values can be calculated from `x(1) + x(2)` and `y(1) + y(2)` respectively. The optimized model eliminates these two redundant variables, reducing memory consumption.
### 2.1.2 Using More Concise Data Structures
Complex data structures, such as nested lists and dictionaries, consume a lot of memory. Using more concise data structures like arrays and tuples can effectively reduce memory consumption.
**Operational Steps:**
1. Analyze the data structure to identify parts that can be simplified.
2. Replace complex data structures with more concise ones.
3. Ensure the simplified data structure meets the requirements of the optimization model.
**Code Example:**
```matlab
% Original Data Structure
data = {
{'a', 'b', 'c'},
{'d', 'e', 'f'},
{'g', 'h', 'i'}
};
% Optimized Data Structure
data = ['a', 'b', 'c'; 'd', 'e', 'f'; 'g', 'h', 'i'];
```
**Logical Analysis:**
The original data structure is a nested list, which occupies more memory. The optimized data structure is an array, which is not only more concise but also consumes less memory.
## 2.2 Optimizing Data Types
### 2.2.1 Choosing Appropriate Numeric Types
Different numeric types occupy different amounts of memory. Choosing an appropriate numeric type can effectively reduce memory consumption.
**Operational Steps:**
1. Analyze the value range of variables in the optimization model.
2. Select an appropriate numeric type based on the value range, such as `int8`, `int16`, `int32`, `float32`, `float64`, etc.
3. Ensure the selected numeric type meets the precision requirements of the optimization model.
**Code Example:**
```matlab
% Original Model
x = optimvar('x', 3, 'LowerBound', -10, 'UpperBound', 10);
% Optimized Model
x = optimvar('x', 3, 'LowerBound', -10, 'UpperBound', 10, 'Type', 'int16');
```
**Logical Analysis:**
In the original model, the value range of variable `x` is [-10, 10], making the use of `double` type (occupying 8 bytes) unnecessary. The optimized model sets the type of `x` to `int16` (occupying 2 bytes), reducing memory consumption.
### 2.2.2 Avoiding Complex Data Structures
Complex data structures, such as nested lists and dictionaries, consume a lot of memory. Using more concise data structures like arrays and tuples can effectively reduce memory consumption.
**Operational Steps:**
1. Analyze the data structure to identify parts that can be simplified.
2. Replace complex data structures with more concise ones.
3. Ensure the simplified data structure meets the requirements of the optimization model.
**Code Example:**
```matlab
% Original Data Structure
data = {
{'a', 'b', 'c'},
{'d', 'e', 'f'},
{'g', 'h', 'i'}
};
% Optimized Data Structure
data = ['a', 'b', 'c'; 'd', 'e', 'f'; 'g', 'h', 'i'];
```
**Logical Analysis:**
The original data structure is a nested list, which occupies more memory. The optimized data structure is an array, which is not only more concise but also consumes less memory.
## 2.3 Reducing Function Calls
### 2.3.1 Inlining Small Functions
The invocation of small functions incurs additional overhead, including the overhead of the function call itself and the allocation and release of internal variables. Inlining small functions can eliminate these overheads, reducing memory consumption.
**Operational Steps:**
1. Analyze the functions called in the optimization model.
2. Identify functions that can be inlined, meaning those with a small body and no side effects.
3. Copy the inline function code directly into the calling function.
**Code Example:**
```matlab
% Original Model
function y = my_function(x)
y = x + 1;
end
x = optimvar('x', 3);
y = my_function(x);
% Optimized Model
x = optimvar('x', 3);
y = x + 1;
```
**Logical Analysis:**
`my_function` is a small function that adds 1 to the input value. The optimized model inlines `my_function` into the main function, eliminating the function call overhead and reducing memory consumption.
### 2.3.2 Caching Function Call Results
For functions that are called frequently, caching their result
0
0
相关推荐
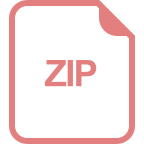
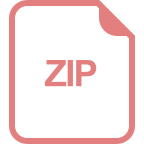
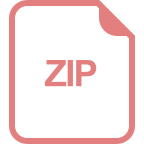
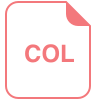
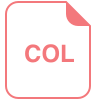
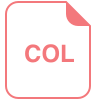
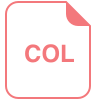

