qml 3d模型运动,绘制轨迹图
时间: 2023-08-18 14:06:07 浏览: 113
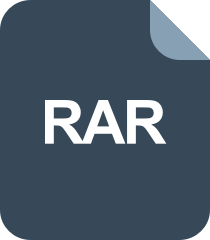
绘制车辆行驶轨迹图

要在QML中绘制3D模型的运动轨迹,可以使用Qt3D模块。以下是一个简单的示例,其中包含一个3D模型和其轨迹图:
```
import Qt3D.Core 2.12
import Qt3D.Render 2.12
import Qt3D.Input 2.12
import Qt3D.Extras 2.12
Entity {
id: sceneRoot
// 3D model
Entity {
id: modelEntity
components: [
// transform component to move the model
Transform {
id: modelTransform
translation: Qt.vector3d(0, 0, -5)
},
// mesh component with a 3D model file
Mesh {
source: "models/myModel.obj"
}
]
}
// trajectory line
Entity {
id: trajectoryEntity
// array of points for the line
property vector3d[] points: [
Qt.vector3d(0, 0, -5),
Qt.vector3d(1, 2, -7),
Qt.vector3d(-3, 1, -9),
Qt.vector3d(2, -1, -11)
]
// component to draw the line
LineRenderer {
id: lineRenderer
material: DefaultMaterial { lineWidth: 3 }
primitiveType: LineRenderer.Lines
geometry: Geometry {
id: lineGeometry
attributes: [
// position attribute
Attribute {
name: defaultAttributeNames.positionAttributeName
attributeType: Attribute.VertexAttribute
vertexBaseType: Attribute.Float
vertexSize: 3
byteOffset: 0
byteStride: 12
count: trajectoryEntity.points.length
buffer: Buffer {
id: lineVertexBuffer
type: Buffer.VertexBuffer
data: trajectoryEntity.points
}
}
]
}
}
}
// animation to move the model along the trajectory
SequentialAnimation {
id: animation
NumberAnimation {
target: modelTransform
property: "translation.x"
from: 0
to: trajectoryEntity.points[0].x
duration: 1000
}
NumberAnimation {
target: modelTransform
property: "translation.y"
from: 0
to: trajectoryEntity.points[0].y
duration: 1000
}
NumberAnimation {
target: modelTransform
property: "translation.z"
from: 0
to: trajectoryEntity.points[0].z
duration: 1000
}
// loop the animation through all the trajectory points
onStopped: {
var nextPoint = (animation.current + 1) % trajectoryEntity.points.length;
animation.to = Qt.vector3d(
trajectoryEntity.points[nextPoint].x,
trajectoryEntity.points[nextPoint].y,
trajectoryEntity.points[nextPoint].z
);
animation.start();
}
}
// start the animation
Component.onCompleted: {
animation.to = Qt.vector3d(
trajectoryEntity.points[1].x,
trajectoryEntity.points[1].y,
trajectoryEntity.points[1].z
);
animation.start();
}
}
```
在此示例中,我们定义了一个3D场景,其中包含一个3D模型和一个轨迹图线。我们还创建了一个动画,该动画将模型沿着轨迹线移动,并在达到每个点时更新目标位置以循环动画。要绘制轨迹,我们使用了LineRenderer组件,该组件根据一组点在3D场景中绘制线条。
阅读全文
相关推荐







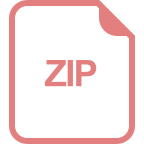
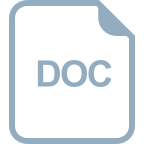
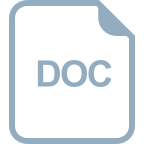



