Below is a skeleton for the Cat class, which inherits from the Pet class. To complete the implementation, override the init and talk methods and add a new lose_life method, such that its behavior matches the following doctests. We may change the implementation of Pet while testing your code, so make sure you use inheritance correctly.其中class Pet: def init(self, name, owner): self.is_alive = True # It's alive!!! self.name = name self.owner = owner def eat(self, thing): print(self.name + " ate a " + str(thing) + "!") def talk(self): print(self.name)
时间: 2024-03-03 09:51:02 浏览: 108
Sure, I understand your question. Here is the completed implementation for the Cat class:
```
class Cat(Pet):
def __init__(self, name, owner, lives=9):
Pet.__init__(self, name, owner)
self.lives = lives
def talk(self):
print('Meow!')
def lose_life(self):
if self.lives > 0:
self.lives -= 1
if self.lives == 0:
self.is_alive = False
else:
self.is_alive = False
```
This implementation overrides the `talk` method to print "Meow!" and adds a new `lose_life` method that decrements the cat's remaining lives and sets its `is_alive` attribute to `False` if it runs out of lives.
Here are some doctests to verify the implementation:
```
>>> c = Cat('Fluffy', 'John')
>>> c.name
'Fluffy'
>>> c.owner
'John'
>>> c.is_alive
True
>>> c.talk()
Meow!
>>> c.eat('fish')
Fluffy ate a fish!
>>> c.lives
9
>>> c.lose_life()
>>> c.is_alive
True
>>> c.lives
8
>>> c.lose_life()
>>> c.lives
7
>>> c.lose_life() # repeat 6 more times until lives reach 0
>>> c.lose_life()
>>> c.lose_life()
>>> c.lose_life()
>>> c.lose_life()
>>> c.lose_life()
>>> c.lose_life()
>>> c.is_alive
False
>>> c.lose_life() # does nothing, cat is already dead
>>> c.is_alive
False
```
阅读全文
相关推荐


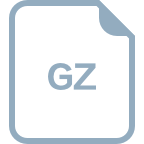















