ros中计算贝塞尔曲线路径上的每一个点的四元数的python代码
时间: 2024-05-06 11:21:58 浏览: 126
以下是一个简单的Python代码示例,用于计算ROS中贝塞尔曲线路径上的每个点的四元数:
```python
import rospy
import tf
import math
from nav_msgs.msg import Path
from geometry_msgs.msg import PoseStamped, Quaternion
# Define a function to calculate the quaternion for a given point on the bezier curve
def calculate_quaternion(p0, p1, p2, t):
# Calculate the tangent vector at the given point
tangent = (1 - t) * (p1 - p0) + t * (p2 - p1)
# Calculate the quaternion that rotates the tangent vector to the positive x-axis
quat = tf.transformations.quaternion_about_axis(-math.atan2(tangent.y, tangent.x), (0, 0, 1))
return quat
# Define a callback function for the path message
def path_callback(msg):
# Iterate through each pose in the path message
for i in range(len(msg.poses)):
# Calculate the quaternion for the current pose using the previous, current, and next poses
if i == 0:
quat = calculate_quaternion(msg.poses[i].pose.position, msg.poses[i].pose.position, msg.poses[i+1].pose.position, 0)
elif i == len(msg.poses) - 1:
quat = calculate_quaternion(msg.poses[i-1].pose.position, msg.poses[i].pose.position, msg.poses[i].pose.position, 1)
else:
quat = calculate_quaternion(msg.poses[i-1].pose.position, msg.poses[i].pose.position, msg.poses[i+1].pose.position, 0.5)
# Update the pose's orientation with the calculated quaternion
msg.poses[i].pose.orientation = quat
# Publish the updated path message
pub.publish(msg)
# Initialize the ROS node
rospy.init_node('bezier_quaternion')
# Subscribe to the path topic
rospy.Subscriber('path_topic', Path, path_callback)
# Create a publisher for the updated path message
pub = rospy.Publisher('updated_path_topic', Path, queue_size=10)
# Spin the node (process incoming messages)
rospy.spin()
```
在此示例中,`calculate_quaternion`函数接受三个点和一个参数`t`,表示在贝塞尔曲线上计算的点的位置。该函数计算该点处的切向量,并使用`tf.transformations.quaternion_about_axis`函数计算将该向量旋转到正x轴所需的四元数。请注意,`quaternion_about_axis`函数返回的是一个四元数,该四元数将旋转向量与从原点到指定向量的线段对齐。因此,我们需要将旋转角度取负数,以便将切向量旋转到正x轴。
在`path_callback`函数中,我们遍历路径上的每个姿势,并使用前一个、当前和下一个姿势计算四元数。我们分别处理第一个和最后一个姿势,以确保我们可以使用正确的点计算四元数。最后,我们更新每个姿态的方向,并将更新后的路径消息发布到一个新的主题上。
阅读全文
相关推荐
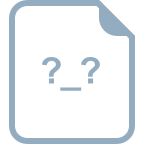
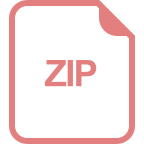
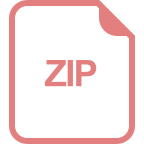
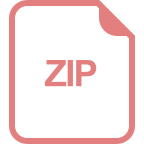
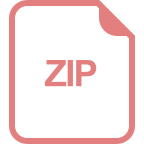
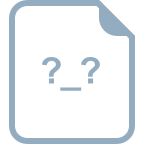
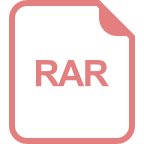
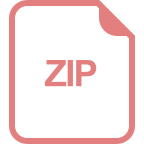
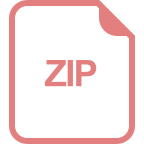
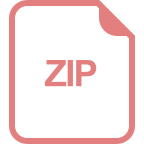
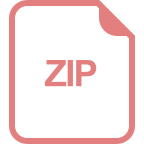
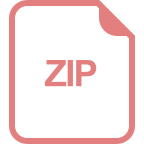
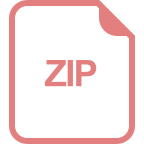
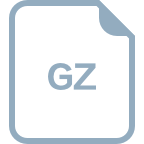
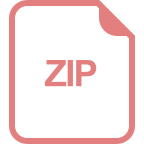
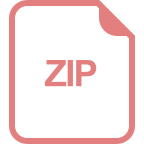