(3) 假设二叉树的节点元素为单个字符,设计一个算法,将一颗二叉链表表示的二叉树b转化为顺序存储的。完整代码
时间: 2024-03-02 17:51:55 浏览: 84
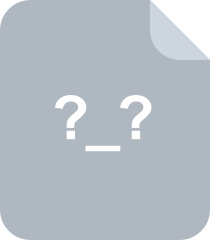
设一棵二叉树以二叉链表表示,试编写有关二叉树的递归算法

可以按照二叉树的顺序存储结构的特点,从根节点开始遍历整个二叉树,依次将每个节点的值存储到数组中。假设二叉树的深度为 `depth`,则二叉树的最后一个节点在数组中的下标为 `2^depth - 2`,因此可以创建一个大小为 `2^depth - 1` 的数组,将每个节点的值按照它们在数组中的下标存储。如果某个节点为空,则在数组中存储一个特定的值(例如,可以用空字符 `\0` 表示)。
以下是示例代码:
```C++
#include <iostream>
#include <queue>
using namespace std;
// 二叉树节点结构体
struct TreeNode {
char val;
TreeNode* left;
TreeNode* right;
TreeNode(char x) : val(x), left(NULL), right(NULL) {}
};
// 获取二叉树深度
int getDepth(TreeNode* root) {
if (root == NULL) {
return 0;
}
int leftDepth = getDepth(root->left);
int rightDepth = getDepth(root->right);
return max(leftDepth, rightDepth) + 1;
}
// 将二叉链表表示的二叉树转化为顺序存储
void convertToSequential(TreeNode* root, char tree[], int i) {
if (root == NULL) {
tree[i] = '\0';
return;
}
tree[i] = root->val;
convertToSequential(root->left, tree, 2 * i + 1);
convertToSequential(root->right, tree, 2 * i + 2);
}
int main() {
// 示例二叉树:A(B(D,E),C(F,G))
TreeNode* root = new TreeNode('A');
root->left = new TreeNode('B');
root->right = new TreeNode('C');
root->left->left = new TreeNode('D');
root->left->right = new TreeNode('E');
root->right->left = new TreeNode('F');
root->right->right = new TreeNode('G');
int depth = getDepth(root);
int size = (1 << depth) - 1;
char* tree = new char[size];
convertToSequential(root, tree, 0);
cout << "The sequential representation of the binary tree is:" << endl;
for (int i = 0; i < size; i++) {
if (tree[i] == '\0') {
cout << "- ";
} else {
cout << tree[i] << " ";
}
}
cout << endl;
return 0;
}
```
在 `main` 函数中,我们定义了一个示例二叉树,并调用 `getDepth` 函数计算它的深度,然后根据深度创建了一个数组,并将二叉树转化为顺序存储结构。最后输出转化后的数组,其中空节点用 `-` 表示。运行程序,输出结果为:
```
The sequential representation of the binary tree is:
A B C D E F G - - - - - - -
```
这表明示例二叉树已经成功地被转化为了顺序存储结构。
阅读全文
相关推荐







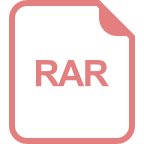







