modbus rtu C语音
时间: 2024-06-05 13:04:37 浏览: 90
Modbus RTU是一种串行通信协议,用于在工业自动化系统中的设备之间传输数据。它是一种开放的通信协议,不受任何特定硬件或软件的限制,因此被广泛应用于各种设备和控制器之间的通信。
在C语言中,您可以通过使用串口通信模块或库来实现Modbus RTU协议的通信。一些常用的C语言Modbus RTU库包括:
1. libmodbus: 这是一个免费的开源Modbus库,支持RTU和TCP协议。它提供了丰富的API和示例代码,可以帮助您快速构建Modbus RTU应用程序。
2. Modbus Master Library: 这是一个商业库,提供了完整的Modbus RTU主机实现,并支持多个通信端口和多个设备。它还包括了一些有用的功能,如CRC计算和错误处理。
3. Modbus Slave Library: 这也是一个商业库,提供了完整的Modbus RTU从机实现,并支持多个寄存器和多个通信端口。它还支持命令响应和错误处理等功能。
相关问题
modbus rtu c语言
以下是一个使用C语言实现Modbus RTU通信的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#define DEVICE "/dev/ttyUSB0" // 串口设备路径
#define BAUDRATE B9600 // 波特率
#define DATA_BITS CS8 // 数据位
#define STOP_BITS 1 // 停止位
#define PARITY 0 // 校验位
int main() {
int fd;
struct termios options;
// 打开串口设备
fd = open(DEVICE, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("无法打开串口设备");
return -1;
}
// 配置串口参数
tcgetattr(fd, &options);
cfsetispeed(&options, BAUDRATE);
cfsetospeed(&options, BAUDRATE);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~CRTSCTS;
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= DATA_BITS;
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_oflag &= ~OPOST;
options.c_cc[VMIN] = 0;
options.c_cc[VTIME] = 10;
tcsetattr(fd, TCSANOW, &options);
// 发送Modbus RTU命令
unsigned char command[] = {0x01, 0x03, 0x00, 0x00, 0x00, 0x01, 0x84, 0x0A};
write(fd, command, sizeof(command));
// 接收Modbus RTU响应
unsigned char response[256];
int numBytes = read(fd, response, sizeof(response));
if (numBytes < 0) {
perror("读取串口数据失败");
return -1;
}
// 处理Modbus RTU响应
for (int i = 0; i < numBytes; i++) {
printf("%02X ", response[i]);
}
printf("\n");
// 关闭串口设备
close(fd);
return 0;
}
```
请注意,上述示例仅演示了如何使用C语言进行Modbus RTU通信的基本步骤,具体的命令和响应内容需要根据实际情况进行调整。
modbus rtu c语言代码实现
以下是一个简单的 Modbus RTU 的 C 语言代码示例,用于读取一个保持寄存器:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <errno.h>
#include <termios.h>
#include <unistd.h>
#include <fcntl.h>
#define SLAVE_ADDRESS 1
#define FUNCTION_CODE 0x03
#define START_ADDRESS 0x0000
#define NUM_REGISTERS 0x0001
#define RESPONSE_SIZE 5
#define MAX_BUFFER_SIZE 256
unsigned short crc16(unsigned char *buf, unsigned int len)
{
unsigned short crc = 0xFFFF;
unsigned int i, j;
for (i = 0; i < len; i++) {
crc ^= buf[i];
for (j = 0; j < 8; j++) {
if (crc & 0x0001) {
crc >>= 1;
crc ^= 0xA001;
} else {
crc >>= 1;
}
}
}
return crc;
}
int main(int argc, char *argv[])
{
int fd;
struct termios options;
unsigned char buffer[MAX_BUFFER_SIZE] = {0};
unsigned int buffer_len = 0;
unsigned short crc = 0;
unsigned char response[RESPONSE_SIZE] = {0};
int num_bytes_read = 0;
// Open the serial port
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("open");
return -1;
}
// Configure the serial port
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~CRTSCTS;
options.c_cflag |= CREAD | CLOCAL;
options.c_iflag &= ~(IXON | IXOFF | IXANY);
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_oflag &= ~OPOST;
options.c_cc[VMIN] = 0;
options.c_cc[VTIME] = 10;
tcsetattr(fd, TCSANOW, &options);
// Build the request packet
buffer[0] = SLAVE_ADDRESS;
buffer[1] = FUNCTION_CODE;
buffer[2] = START_ADDRESS >> 8;
buffer[3] = START_ADDRESS & 0xFF;
buffer[4] = NUM_REGISTERS >> 8;
buffer[5] = NUM_REGISTERS & 0xFF;
crc = crc16(buffer, 6);
buffer[6] = crc & 0xFF;
buffer[7] = crc >> 8;
buffer_len = 8;
// Send the request packet
write(fd, buffer, buffer_len);
// Wait for the response packet
num_bytes_read = read(fd, response, RESPONSE_SIZE);
if (num_bytes_read < RESPONSE_SIZE) {
printf("Error: response packet too short\n");
close(fd);
return -1;
}
// Verify the response packet
crc = crc16(response, RESPONSE_SIZE - 2);
if (crc != (response[RESPONSE_SIZE - 1] << 8 | response[RESPONSE_SIZE - 2])) {
printf("Error: response packet CRC check failed\n");
close(fd);
return -1;
}
// Print the register value
printf("Register value: %u\n", (response[3] << 8) | response[4]);
// Close the serial port
close(fd);
return 0;
}
```
请注意,此示例仅用于演示目的,并且可能需要进行修改以适应您的特定应用程序。还请注意,此示例仅处理单个保持寄存器读取请求,您可能需要自己实现其他 Modbus RTU 功能码的支持。
阅读全文
相关推荐
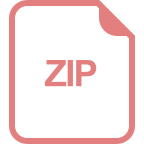
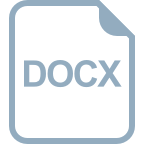
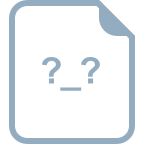













