C 语言悔棋代码
时间: 2023-08-06 10:20:43 浏览: 88
以下是一个简单的 C 语言悔棋代码的示例,使用了栈的数据结构来实现:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100
typedef struct {
int x; // 棋子的横坐标
int y; // 棋子的纵坐标
} Chess;
typedef struct {
Chess data[MAXSIZE];
int top;
} Stack;
void init(Stack *s) {
s->top = -1;
}
int isEmpty(Stack *s) {
return s->top == -1;
}
int isFull(Stack *s) {
return s->top == MAXSIZE - 1;
}
void push(Stack *s, Chess c) {
if (isFull(s)) {
printf("Stack is full!\n");
exit(0);
}
s->data[++s->top] = c;
}
Chess pop(Stack *s) {
if (isEmpty(s)) {
printf("Stack is empty!\n");
exit(0);
}
return s->data[s->top--];
}
int main() {
Stack s;
Chess c;
int i, j;
char board[15][15] = {0};
init(&s);
// 棋盘初始化
for (i = 0; i < 15; i++) {
for (j = 0; j < 15; j++) {
board[i][j] = '+';
}
}
// 下棋操作
while (1) {
printf("Please enter the coordinates of your move (x y): ");
scanf("%d %d", &c.x, &c.y);
if (board[c.x][c.y] == '+') {
board[c.x][c.y] = '*'; // 在棋盘上打印一个 *
push(&s, c); // 将当前棋子压入栈中
} else {
printf("This place has already been taken! Please re-enter.\n");
}
// 悔棋操作
if (!isEmpty(&s)) {
printf("Do you want to regret? (y/n): ");
char answer;
scanf(" %c", &answer);
if (answer == 'y' || answer == 'Y') {
c = pop(&s); // 弹出栈顶棋子
board[c.x][c.y] = '+'; // 在棋盘上将该棋子标记为 +
} else {
continue;
}
}
// 打印棋盘
for (i = 0; i < 15; i++) {
for (j = 0; j < 15; j++) {
printf("%c ", board[i][j]);
}
printf("\n");
}
}
return 0;
}
```
该代码实现了一个简单的五子棋游戏,可以在游戏过程中进行悔棋操作。其核心思想是使用栈来保存每一步的棋子坐标,如果需要悔棋,则弹出栈顶元素并在棋盘上标记为 +。
阅读全文
相关推荐
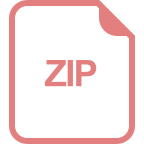
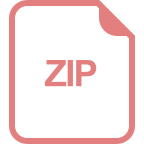
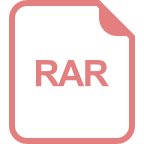















