Must all of the values of an array be of the same type?
时间: 2024-05-20 19:15:29 浏览: 96
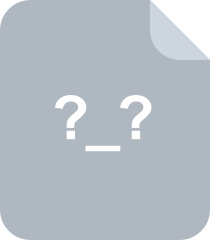
比较两个数组元素是否相同

Yes, all of the values in an array must be of the same data type. This is because an array is a collection of elements of the same data type that are stored in contiguous memory locations. When you declare an array, you specify the data type of its elements. All of the elements in the array must be of that same data type. If you try to store values of different data types in the same array, you will get a compilation error.
阅读全文