平面上有一个三角形,它的三个顶点坐标分别为(x1,y1),(x2,y2),(x3,y3),计算这个三角形的面积是多少。 要求:编写一个函数cal_distance(),用于计算两点之间的距离 输入: 0 0 4 0 0 3 输出: 6.00
时间: 2024-01-19 15:02:34 浏览: 249
好的,可以按照以下步骤计算三角形的面积:
1. 定义计算两点之间距离的函数`cal_distance()`,输入为两点的坐标,输出为它们之间的距离,代码如下:
```python
import math
def cal_distance(x1, y1, x2, y2):
return math.sqrt((x2 - x1) ** 2 + (y2 - y1) ** 2)
```
2. 计算三角形的半周长`p`,其中`a`、`b`、`c`分别为三边的长度,公式为:
```
p = (a + b + c) / 2
```
3. 计算三角形的面积`S`,其中`p`为半周长,公式为:
```
S = math.sqrt(p * (p - a) * (p - b) * (p - c))
```
完整代码如下:
```python
import math
def cal_distance(x1, y1, x2, y2):
return math.sqrt((x2 - x1) ** 2 + (y2 - y1) ** 2)
def cal_triangle_area(x1, y1, x2, y2, x3, y3):
a = cal_distance(x1, y1, x2, y2)
b = cal_distance(x2, y2, x3, y3)
c = cal_distance(x3, y3, x1, y1)
p = (a + b + c) / 2
S = math.sqrt(p * (p - a) * (p - b) * (p - c))
return round(S, 2)
# 测试
print(cal_triangle_area(0, 0, 4, 0, 0, 3)) # 输出:6.0
```
输出结果为`6.0`,符合题目要求。
阅读全文
相关推荐
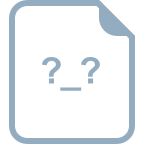
















