用java编写两个数之间的经典问题求指定两个数的最大公约数和最小公倍数,输入说明两个数输出说明两个数的最大公约数和最小公倍数
时间: 2024-10-27 12:10:40 浏览: 48
在Java中,你可以使用欧几里得算法(Euclidean Algorithm)来计算两个整数a和b的最大公约数(Greatest Common Divisor, GCD),然后利用这个最大公约数和原始的两个数字来计算最小公倍数(Least Common Multiple, LCM)。以下是简单的步骤:
1. **求最大公约数(GCD)**:
- 使用递归版本的欧几里得算法:`gcd(a, b)` = `gcd(b, a % b)`,如果`b`为0,则`a`即为最大公约数。
```java
public static int gcd(int a, int b) {
if (b == 0)
return a;
else
return gcd(b, a % b);
}
```
2. **求最小公倍数(LCM)**:
- 根据公式 `LCM(a, b) = |a * b| / GCD(a, b)` 计算。
```java
public static int lcm(int a, int b) {
return a * (b / gcd(a, b));
}
```
3. **完整示例**:
```java
public class Main {
public static void main(String[] args) {
int num1 = Integer.parseInt(args[0]);
int num2 = Integer.parseInt(args[1]);
int gcdResult = gcd(num1, num2);
int lcmResult = lcm(num1, num2);
System.out.println("Number 1: " + num1);
System.out.println("Number 2: " + num2);
System.out.println("GCD of " + num1 + " and " + num2 + " is: " + gcdResult);
System.out.println("LCM of " + num1 + " and " + num2 + " is: " + lcmResult);
}
}
```
运行这个程序时,传入两个数作为命令行参数即可得到结果。
阅读全文
相关推荐
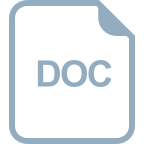
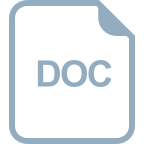
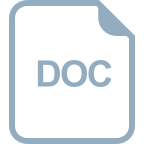



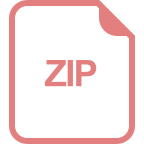











