ValueError Traceback (most recent call last) <ipython-input-56-571552a9fb46> in <module>() ----> 1 prediction = model.predict(number_data_normalize) 2 print(prediction) 3 np.max(prediction) 4 np.argmax(prediction) D:\360downloads\Anaconda\envs\tensorflow_cpu\lib\site-packages\keras\engine\training.py in predict(self, x, batch_size, verbose, steps) 1147 'argument.') 1148 # Validate user data. -> 1149 x, _, _ = self._standardize_user_data(x) 1150 if self.stateful: 1151 if x[0].shape[0] > batch_size and x[0].shape[0] % batch_size != 0: D:\360downloads\Anaconda\envs\tensorflow_cpu\lib\site-packages\keras\engine\training.py in _standardize_user_data(self, x, y, sample_weight, class_weight, check_array_lengths, batch_size) 749 feed_input_shapes, 750 check_batch_axis=False, # Don't enforce the batch size. --> 751 exception_prefix='input') 752 753 if y is not None: D:\360downloads\Anaconda\envs\tensorflow_cpu\lib\site-packages\keras\engine\training_utils.py in standardize_input_data(data, names, shapes, check_batch_axis, exception_prefix) 126 ': expected ' + names[i] + ' to have ' + 127 str(len(shape)) + ' dimensions, but got array ' --> 128 'with shape ' + str(data_shape)) 129 if not check_batch_axis: 130 data_shape = data_shape[1:] ValueError: Error when checking input: expected conv2d_1_input to have 4 dimensions, but got array with shape (1, 784)
时间: 2024-02-14 15:27:07 浏览: 25
这个错误是因为模型期望输入有四个维度,而你的输入数据只有两个维度。在使用CNN进行图像预测时,通常需要将图像转换为四维张量,形状为(batch_size, height, width, channels)。
在你的情况下,你的输入数据只有一个样本,所以batch_size为1,但是缺少height、width和channels信息。根据你的代码,看起来你的图像是灰度图像,并且大小为28x28。
你可以将输入数据重塑为四维张量,如下所示:
```python
number_data_normalize = number_data_normalize.reshape(1, 28, 28, 1)
```
这里,我们将数据从(1, 784)重塑为(1, 28, 28, 1),其中1表示通道数。请注意,这里的通道数是1,因为灰度图像只有一个通道。
然后,你可以再次尝试使用模型进行预测:
```python
prediction = model.predict(number_data_normalize)
print(prediction)
np.max(prediction)
np.argmax(prediction)
```
这样应该就可以正确地进行预测了。记得确保加载了正确的模型,并且模型已经针对MINST数据集进行了训练。
相关问题
ValueError Traceback (most recent call last) <ipython-input-1-e77514c20961> in <module> 25 26 # 构建新的数据集 ---> 27 for user_id, group in tqdm(grouped): 28 songs = list(group['song_id']) 29 for i in range(len(songs)): ValueError: too many values to unpack (expected 2)
这是一个 Python 的 ValueError 异常,提示你的代码在执行时出现了错误。具体来说,是因为在第 27 行代码中,你使用了 unpacking 的方式,但是却期望得到 2 个返回值。而实际上,grouped 这个变量返回的值可能不是一个长度为 2 的 tuple。你可以检查一下 grouped 这个变量的类型和值,看看是否符合你的预期。如果不符合,你需要调整代码以适应实际情况。
ValueError Traceback (most recent call last) <ipython-input-9-30d4162d3ab9> in <module>() ----> 1 contours, hierarchy = cv2.findContours(image, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE) 2 3 for item in contours: 4 rect = cv2.boundingRect(item) 5 x = rect[0] ValueError: too many values to unpack (expected 2)
这个错误的原因是 `cv2.findContours()` 函数的返回值在 OpenCV 3 和 OpenCV 4 中有所不同。在 OpenCV 4 中, `cv2.findContours()` 返回两个值,分别是轮廓和层级信息。而在 OpenCV 3 中, `cv2.findContours()` 只返回轮廓信息。
因此,如果你使用的是 OpenCV 3,那么你需要将代码修改为:
```python
contours, _ = cv2.findContours(image, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
```
如果你使用的是 OpenCV 4,则可以保留原来的代码不变。
相关推荐
















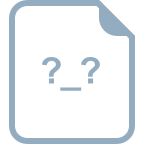