运用C语言编写一个完整的程序,用两个顺序表分别存储一元多项式p1=8+5x-7x^2+9x^3+12x^4-6x^7和p2=7-2x+6x^2,完成两个多项式的加法,依次将p1和p二对应下标的元素相加,结果存于p1,如果p2中的元素多余p1,将p2中多出的元素全部复制到p1。
时间: 2024-12-02 15:21:11 浏览: 18
在C语言中,我们可以创建结构体来表示多项式的项,然后定义两个链表来存储多项式。由于没有内置的数据结构支持多项式,我们通常会使用动态内存分配来模拟这种结构。下面是一个简单的示例程序,演示如何实现这个需求:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Term {
int coefficient; // 系数
int power; // 指数
} Term;
typedef struct Polynomial {
Term* terms;
int degree; // 最高次幂
int size;
} Polynomial;
void addTerm(Polynomial* p1, Term* new_term) {
if (p1->degree + new_term->power < p1->degree)
p1->degree = new_term->power;
if (p1->size == p1->degree + 1) { // 扩展链表
Term* temp = realloc(p1->terms, sizeof(Term) * (p1->size + 1));
if (!temp) {
printf("Memory allocation failed.\n");
return;
}
p1->terms = temp;
}
p1->terms[p1->size++] = *new_term;
}
void printPolynomial(Polynomial p) {
for (int i = 0; i <= p.degree; ++i) {
if (p.terms[i].coefficient != 0)
printf("%d*x^%d + ", p.terms[i].coefficient, i);
}
if (p.terms[0].coefficient == 0)
printf("0\n");
else
printf("\n");
}
void addPolynomials(Polynomial* p1, Polynomial* p2) {
for (int i = 0; i <= p2->degree; ++i) {
Term term = {p2->terms[i].coefficient, i};
addTerm(p1, &term);
}
}
// 初始化多项式
Polynomial createPolynomial(int coefficients[], int degree) {
Polynomial p;
p.degree = degree;
p.size = degree + 1;
p.terms = malloc(sizeof(Term) * p.size);
for (int i = 0; i <= degree; ++i) {
p.terms[i].coefficient = coefficients[i];
p.terms[i].power = i;
}
return p;
}
int main() {
int p1[] = {8, 5, -7, 9, 12, -6, 0}; // 一元多项式p1
int p2[] = {7, -2, 6, 0, 0, 0, 0}; // 一元多项式p2
Polynomial p1_poly = createPolynomial(p1, sizeof(p1) / sizeof(p1[0]));
Polynomial p2_poly = createPolynomial(p2, sizeof(p2) / sizeof(p2[0]));
addPolynomials(&p1_poly, &p2_poly);
printPolynomial(p1_poly); // 输出加法后的多项式p1
free(p1_poly.terms);
free(p2_poly.terms);
return 0;
}
```
在这个程序中,`addTerm` 函数用于添加一个多项式的项,`createPolynomial` 用于初始化多项式,`printPolynomial` 用于打印多项式。`addPolynomials` 函数实现了两个多项式的加法。
注意:这里假设输入的多项式数组只包含非负指数的项,如果可能存在负指数的情况,需要稍作修改以处理。运行此程序前确保有足够的内存可用。
阅读全文
相关推荐
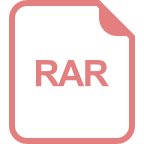
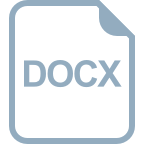
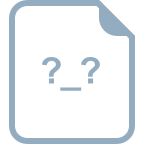











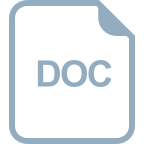



