python写代码:文件输入任意CFG,某句型a 输出:a的最左、最右推导,画出a的语法树,得到该句子的推导过程,并给出运行结果
时间: 2024-10-20 19:14:18 浏览: 44
在Python中处理上下文无关文法(CFG)和生成语法树通常需要结合`pyparsing`库来解析文法,以及`networkx`或`graphviz`库来绘制语法树。以下是一个简单的步骤概述:
1. 首先,你需要安装必要的库,如果尚未安装,可以使用pip安装:
```
pip install pyparsing networkx graphviz
```
2. 定义你的文法和非终结符(例如,用字典表示),这里假设有一个简单文法比如LL(1)文法:
```python
grammar = {
'S': ['A', 'B'],
'A': ['a'],
'B': ['b'],
}
terminals = ['a', 'b']
start_symbol = 'S'
```
3. 使用`pyparsing`解析输入的CFG:
```python
from pyparsing import Word
def parse_grammar(grammar_str):
rules = []
for rule in grammar_str.splitlines():
if ':' not in rule:
continue
lhs, rhs = rule.split(':')
rhs_tokens = [Word(terminals, exact=len(t)) for t in rhs.strip().split()]
rules.append((lhs, tuple(rhs_tokens)))
return rules
```
4. 输入文件内容,并解析:
```python
with open('input.cfg') as f:
grammar_str = f.read()
parsed_rules = parse_grammar(grammar_str)
```
5. 创建图结构并构建语法树:
```python
import networkx as nx
from networkx.drawing.nx_pydot import to_pydot
def build_tree(rules, input_string, start_symbol=start_symbol):
tree = nx.DiGraph()
stack = [(start_symbol, [])]
for token in input_string:
current_node, child_nodes = stack.pop()
child_nodes.append(token)
for rule, children in rules[current_node]:
if children == child_nodes:
next_node = rule
break
else:
raise ValueError(f"Invalid input string for {current_node}")
tree.add_edge(current_node, next_node, children=child_nodes)
stack.append((next_node, []))
return tree
input_string = "ab"
tree = build_tree(parsed_rules, input_string)
```
6. 绘制语法树:
```python
dot_graph = to_pydot(tree)
dot_graph.write_pdf("tree.pdf")
```
7. 最左、最右推导:
```python
def leftmost derivations(tree):
# 这里只是一个示例,实际实现可能更复杂,取决于文法的具体结构
pass
def rightmost derivations(tree):
# 同样,这只是一个示例
pass
leftmost_derivations(tree), rightmost_derivations(tree)
```
注意,由于上述代码是简化版的示例,实际处理复杂的文法可能需要更完整的错误检查和处理,包括处理文法无效的情况。此外,对于最左和最右推导的实现,具体算法会依据文法的性质有所不同。
阅读全文
相关推荐
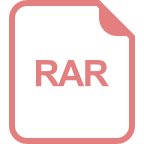
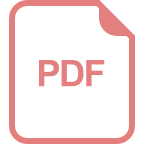
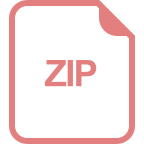




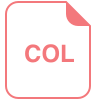
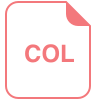

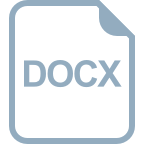
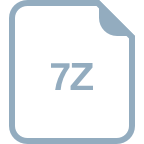
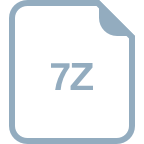
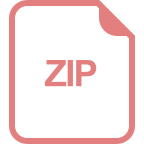
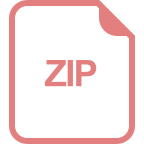