输入完函数的指数和系数怎么输出函数c语言
时间: 2024-10-23 10:11:34 浏览: 20
在 C 语言中,如果你想要输入函数的指数(幂次)和系数,通常会使用标准输入流(如 `scanf` 函数)来获取用户的输入,并将它们存储到变量中。然后你可以创建一个表示多项式的结构体或者简单的数据结构来保存这些信息。下面是一个简单的例子:
```c
#include <stdio.h>
// 创建一个表示多项式项的数据结构
typedef struct {
double coefficient; // 系数
int exponent; // 指数
} PolynomialTerm;
// 定义计算多项式值的函数
double calculate_polynomial(PolynomialTerm terms[], int num_terms, double x) {
double result = 0;
for (int i = 0; i < num_terms; i++) {
result += terms[i].coefficient * pow(x, terms[i].exponent);
}
return result;
}
int main() {
PolynomialTerm terms[2]; // 假设最多支持两个项
int num_terms, index = 0;
printf("请输入第一个项的系数(例如:1):");
scanf("%lf", &terms[index++].coefficient);
printf("请输入第一个项的指数(例如:2):");
scanf("%d", &terms[index++].exponent); // 注意这里直接输入整数
if (index < 2) {
printf("请输入第二个项的系数(如果不需要,可以输入0):");
if (scanf("%lf", &terms[index].coefficient) != EOF && terms[index].coefficient != 0) {
scanf("%d", &terms[index++].exponent);
} else {
index--;
}
}
// 假设用户只输入了两项
num_terms = index;
double x; // 用户需要求解的x值
printf("请输入自变量x的值:");
scanf("%lf", &x);
double polynomial_value = calculate_polynomial(terms, num_terms, x);
printf("当x=%lf时,多项式的值为:%lf\n", x, polynomial_value);
return 0;
}
```
在这个示例中,用户会被提示输入每个项的系数和指数。然后,主函数会调用 `calculate_polynomial` 来计算给定 `x` 值下的多项式值。
阅读全文
相关推荐
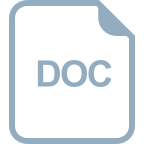
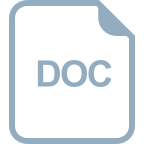
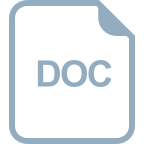
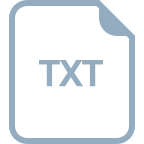














