实现 pow(x, n) 用c++写完整程序,要求在输入一个实数 x 和一个整数 n 后,能够计算出值,并进行最终输出。并用类进行封装,
时间: 2024-05-01 14:23:37 浏览: 162
下面是使用类封装的 C++ 实现 pow(x, n) 的完整程序:
```cpp
#include <iostream>
using namespace std;
class PowCalculator {
public:
double pow(double x, int n) {
if (n == 0) {
return 1.0;
}
double half = pow(x, n / 2);
if (n % 2 == 0) {
return half * half;
} else if (n > 0) {
return x * half * half;
} else {
return (1 / x) * half * half;
}
}
};
int main() {
double x;
int n;
cout << "Please input x and n: ";
cin >> x >> n;
PowCalculator calculator;
double result = calculator.pow(x, n);
cout << "Result: " << result << endl;
return 0;
}
```
在这个程序中,我们使用 `PowCalculator` 类来封装 pow(x, n) 的计算,其中类中的 `pow` 函数采用递归的方式来计算幂次,具体实现如下:
- 当 n = 0 时,返回 1.0。
- 当 n 为偶数时,将 pow(x, n) 转化为 pow(x, n/2) * pow(x, n/2)。
- 当 n 为奇数时,将 pow(x, n) 转化为 x * pow(x, n/2) * pow(x, n/2)。
- 当 n 为负数时,将 pow(x, n) 转化为 (1/x) * pow(x, -n)。
在 `main` 函数中,我们首先输入 x 和 n 的值,然后创建 `PowCalculator` 类的实例,使用 `pow` 函数计算 pow(x, n) 的值,最后输出结果。
需要注意的是,对于输入的 x 和 n 的值,我们没有进行太多的检查,比如 n 是否为负数或者 x 是否为 0。在实际应用中,这些情况需要进行检查并进行相应的处理。
阅读全文
相关推荐
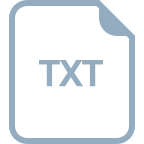













