pcl::visualization::PointCloudColorHandlerGenericField<pcl::PointXYZ>对象怎么手动释放
时间: 2023-07-02 13:15:49 浏览: 44
虽然不建议手动释放`pcl::visualization::PointCloudColorHandlerGenericField<pcl::PointXYZ>`对象,但是如果确实需要手动释放,可以使用C++的delete关键字来释放对象。示例代码如下:
```
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>);
// 假设有一个PointCloudColorHandlerGenericField对象指向cloud的curvature字段
pcl::visualization::PointCloudColorHandlerGenericField<pcl::PointXYZ>::Ptr color_handler(
new pcl::visualization::PointCloudColorHandlerGenericField<pcl::PointXYZ>(cloud, "curvature"));
// 使用完对象后手动释放
delete color_handler;
```
需要注意的是,如果在程序的其他地方还有指向这个对象的指针,那么手动释放会导致内存错误。因此,在使用delete手动释放对象之前,应该检查是否还有其他指针指向这个对象。同时,建议使用智能指针来管理对象的生命周期,不需要手动释放对象。
相关问题
pcl::visualization::PointCloudColorHandlerGenericFieldpcl::PointXYZ color_handler 怎么手动释放
同样不建议手动释放`pcl::visualization::PointCloudColorHandlerGenericField<pcl::PointXYZ>`对象,但如果确实需要手动释放,可以使用`delete`关键字来释放对象指针,示例代码如下:
```
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>);
// 假设有一个PointCloudColorHandlerGenericField对象指向cloud的curvature字段
pcl::visualization::PointCloudColorHandlerGenericField<pcl::PointXYZ>* color_handler_ptr =
new pcl::visualization::PointCloudColorHandlerGenericField<pcl::PointXYZ>(cloud, "curvature");
// 在使用完color_handler_ptr之后,手动释放
delete color_handler_ptr;
```
需要注意的是,在手动释放对象指针之前,应该确保该指针不再被使用,否则可能会导致内存错误。另外,当使用智能指针时,不需要手动释放对象,因为智能指针会自动在需要的时候释放对象。
用VTK 显示 pcl::PointCloud<pcl::PointXYZ>::Ptr 类型点云
要使用 VTK 显示 PCL 的点云,需要将 PCL 的点云数据转换为 VTK 的数据类型。可以使用 `pcl::visualization::PCLVisualizer` 类来实现这个功能。以下是一个简单的示例程序,展示如何使用 VTK 来显示 PCL 点云数据:
```cpp
#include <pcl/point_types.h>
#include <pcl/visualization/pcl_visualizer.h>
#include <vtkRenderWindow.h>
int main ()
{
// 创建一个 PCL 的点云
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>);
cloud->width = 5;
cloud->height = 1;
cloud->is_dense = false;
cloud->points.resize(cloud->width * cloud->height);
for (size_t i = 0; i < cloud->points.size(); ++i)
{
cloud->points[i].x = 1024 * rand() / (RAND_MAX + 1.0f);
cloud->points[i].y = 1024 * rand() / (RAND_MAX + 1.0f);
cloud->points[i].z = 1024 * rand() / (RAND_MAX + 1.0f);
}
// 创建一个可视化窗口
pcl::visualization::PCLVisualizer viewer("Cloud Viewer");
// 将 PCL 的点云数据转换为 VTK 的数据类型
vtkSmartPointer<vtkPoints> vtk_points = vtkSmartPointer<vtkPoints>::New();
for (size_t i = 0; i < cloud->points.size(); ++i)
{
vtk_points->InsertNextPoint(cloud->points[i].x, cloud->points[i].y, cloud->points[i].z);
}
vtkSmartPointer<vtkPolyData> vtk_polydata = vtkSmartPointer<vtkPolyData>::New();
vtk_polydata->SetPoints(vtk_points);
// 将 VTK 的数据类型添加到可视化窗口中
viewer.addModelFromPolyData(vtk_polydata, "cloud");
// 显示可视化窗口
while (!viewer.wasStopped())
{
viewer.spinOnce();
}
return 0;
}
```
在上面的示例程序中,我们首先创建了一个 PCL 的点云数据,并使用 `pcl::visualization::PCLVisualizer` 类创建了一个 VTK 的可视化窗口。然后,我们将 PCL 的点云数据转换为 VTK 的数据类型,并将其添加到可视化窗口中。最后,我们进入了一个循环,显示可视化窗口,直到用户关闭窗口。
相关推荐
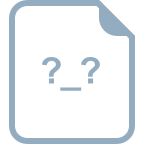












