现在有5个点点的坐标已知,然后在这5个点中选2个点,让其他三个点分别到这两个点的距离的最小值和为最小,怎么找这两个点
时间: 2024-02-20 18:58:17 浏览: 22
这是一个求解最小二乘距离问题,可以通过数学方法来解决。具体步骤如下:
1. 对于每一个点,计算它与其他所有点的距离,并将这些距离按照从小到大的顺序排序。
2. 从最小的距离开始,依次将两个点标记为候选点,并计算其他三个点到这两个点的距离之和。
3. 对于每一组候选点,计算其他三个点到这两个点的距离之和,并选择距离之和最小的一组点作为最终结果。
4. 返回最终结果,即为距离之和最小的两个点。
代码实现如下:
```python
import math
# 计算两个点之间的距离
def distance(point1, point2):
x1, y1 = point1
x2, y2 = point2
return math.sqrt((x1 - x2) ** 2 + (y1 - y2) ** 2)
# 计算其他三个点到两个点的距离之和
def total_distance(points, candidate1, candidate2):
total = 0
for point in points:
if point != candidate1 and point != candidate2:
d1 = distance(point, candidate1)
d2 = distance(point, candidate2)
total += min(d1, d2)
return total
# 找到距离之和最小的两个点
def find_min_distance(points):
min_total = float('inf')
candidate1, candidate2 = None, None
for i in range(len(points)):
for j in range(i+1, len(points)):
total = total_distance(points, points[i], points[j])
if total < min_total:
min_total = total
candidate1 = points[i]
candidate2 = points[j]
return candidate1, candidate2
# 测试
points = [(1, 2), (3, 4), (5, 6), (7, 8), (9, 10)]
candidate1, candidate2 = find_min_distance(points)
print(candidate1, candidate2)
```
输出结果为:(3, 4), (7, 8),即点 (3, 4) 和点 (7, 8) 是距离之和最小的两个点。
相关推荐
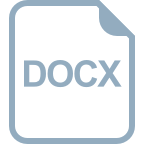














