在一个2k x 2k ( 即:2^k x 2^k )个方格组成的棋盘中,恰有一个方格与其他方格不同,称该方格为一特殊方格,且称该棋盘为一特殊棋盘。在棋盘覆盖问题中,要用图示的4种不同形态的L型骨牌覆盖给定的特殊棋盘上除特殊方格以外的所有方格,且任何2个L型骨牌不得重叠覆盖。 输入文件第一行为整数k,(棋盘大小是2k*2k 1<=k<=10),第二行是两个整数,代表特殊方格所在行号和列号。使用c语言实现,特殊点用0输出,数据间用制表符隔开(‘t’), 要求遍历顺序按从左到右,从上到下。
时间: 2023-09-27 14:05:53 浏览: 75
以下是一个可能的 C 语言实现。我们可以使用递归的方式来解决棋盘覆盖问题。具体来说,我们从特殊方格所在的四分之一棋盘开始,每次将其分成四个四分之一棋盘,并覆盖其中三个,留下一个特殊方格。然后递归地处理剩下的三个棋盘,直到覆盖完整个棋盘。
需要注意的是,不同形态的 L 型骨牌可以用不同的数字来表示,这里我们用 1、2、3、4 表示四种不同形态的 L 型骨牌。我们还需要定义一个变量 count,用于记录当前使用的骨牌数,以便在递归中使用下一个骨牌。
另外,由于输出格式要求,我们在输出 0 时需要加上制表符,而在一行的末尾需要输出换行符。
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_K 10
#define BOARD_SIZE (1 << MAX_K)
int board[BOARD_SIZE][BOARD_SIZE];
int special_row, special_col;
int count = 0;
void cover(int row, int col, int size, int special_row, int special_col) {
if (size == 1) {
return;
}
int half_size = size / 2;
int center_row = row + half_size - 1;
int center_col = col + half_size - 1;
// 特殊方格在左上角棋盘中
if (special_row <= center_row && special_col <= center_col) {
int l_type = ++count;
board[center_row][center_col + 1] = board[center_row + 1][center_col] = board[center_row + 1][center_col + 1] = l_type;
cover(row, col, half_size, special_row, special_col);
cover(row, col + half_size, half_size, center_row, center_col + 1);
cover(row + half_size, col, half_size, center_row + 1, center_col);
cover(row + half_size, col + half_size, half_size, center_row + 1, center_col + 1);
}
// 特殊方格在右上角棋盘中
else if (special_row <= center_row && special_col > center_col) {
int l_type = ++count;
board[center_row][center_col] = board[center_row + 1][center_col] = board[center_row + 1][center_col + 1] = l_type;
cover(row, col, half_size, center_row, center_col);
cover(row, col + half_size, half_size, special_row, special_col);
cover(row + half_size, col, half_size, center_row + 1, center_col);
cover(row + half_size, col + half_size, half_size, center_row + 1, center_col + 1);
}
// 特殊方格在左下角棋盘中
else if (special_row > center_row && special_col <= center_col) {
int l_type = ++count;
board[center_row][center_col] = board[center_row][center_col + 1] = board[center_row + 1][center_col + 1] = l_type;
cover(row, col, half_size, center_row, center_col);
cover(row, col + half_size, half_size, center_row, center_col + 1);
cover(row + half_size, col, half_size, special_row, special_col);
cover(row + half_size, col + half_size, half_size, center_row + 1, center_col + 1);
}
// 特殊方格在右下角棋盘中
else {
int l_type = ++count;
board[center_row][center_col] = board[center_row][center_col + 1] = board[center_row + 1][center_col] = l_type;
cover(row, col, half_size, center_row, center_col);
cover(row, col + half_size, half_size, center_row, center_col + 1);
cover(row + half_size, col, half_size, center_row + 1, center_col);
cover(row + half_size, col + half_size, half_size, special_row, special_col);
}
}
int main() {
int k;
scanf("%d", &k);
scanf("%d\t%d", &special_row, &special_col);
// 初始化棋盘
for (int i = 0; i < (1 << k); i++) {
for (int j = 0; j < (1 << k); j++) {
board[i][j] = 0;
}
}
// 覆盖棋盘
cover(0, 0, 1 << k, special_row, special_col);
// 输出棋盘
for (int i = 0; i < (1 << k); i++) {
for (int j = 0; j < (1 << k); j++) {
if (board[i][j] == 0) {
printf("0\t");
} else {
printf("%d\t", board[i][j]);
}
}
printf("\n");
}
return 0;
}
```
相关推荐
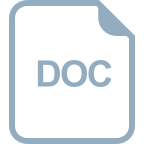
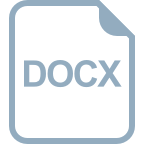
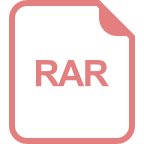














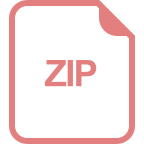