std::vector<pcl::PointCloud<pcl::PointXYZ>> &cluster_cloud1解释一下这段代码
时间: 2023-04-12 10:01:27 浏览: 279
这是一个 C++ 中的 std::vector 容器,其中存储了 pcl::PointCloud<pcl::PointXYZ> 类型的元素。pcl::PointCloud<pcl::PointXYZ> 是一个点云数据结构,表示三维空间中的点。因此,这个 std::vector 存储了多个三维点云数据。
相关问题
上述提到的pcl::KMeans详细实现代码
下面是一个简单的使用pcl::KMeans实现k-means聚类的示例代码:
```c++
#include <pcl/features/normal_3d.h>
#include <pcl/filters/voxel_grid.h>
#include <pcl/kdtree/kdtree_flann.h>
#include <pcl/segmentation/extract_clusters.h>
#include <pcl/features/fpfh.h>
#include <pcl/kdtree/kmeans.h>
// 定义点云类型
typedef pcl::PointXYZ PointType;
typedef pcl::PointCloud<PointType> PointCloud;
typedef pcl::Normal NormalType;
typedef pcl::PointCloud<NormalType> NormalCloud;
typedef pcl::FPFHSignature33 FeatureType;
typedef pcl::PointCloud<FeatureType> FeatureCloud;
int main()
{
// 加载点云数据
PointCloud::Ptr cloud(new PointCloud);
pcl::io::loadPCDFile("input.pcd", *cloud);
// 下采样
pcl::VoxelGrid<PointType> grid;
grid.setLeafSize(0.01, 0.01, 0.01);
grid.setInputCloud(cloud);
PointCloud::Ptr cloud_downsampled(new PointCloud);
grid.filter(*cloud_downsampled);
// 计算法线
pcl::NormalEstimation<PointType, NormalType> ne;
ne.setInputCloud(cloud_downsampled);
pcl::search::KdTree<PointType>::Ptr tree(new pcl::search::KdTree<PointType>);
ne.setSearchMethod(tree);
NormalCloud::Ptr normals(new NormalCloud);
ne.setRadiusSearch(0.03);
ne.compute(*normals);
// 计算特征
pcl::FPFHEstimation<PointType, NormalType, FeatureType> fpfh;
fpfh.setInputCloud(cloud_downsampled);
fpfh.setInputNormals(normals);
fpfh.setSearchMethod(tree);
FeatureCloud::Ptr features(new FeatureCloud);
fpfh.setRadiusSearch(0.05);
fpfh.compute(*features);
// k-means聚类
int k = 3;
pcl::KMeans<FeatureType> kmeans;
kmeans.setClusterSize(k);
kmeans.setEuclideanDistance(true);
kmeans.setInputCloud(features);
std::vector<pcl::PointIndices> cluster_indices;
kmeans.extract(cluster_indices);
// 输出聚类结果
for (int i = 0; i < cluster_indices.size(); i++)
{
std::cout << "Cluster " << i << ":\n";
for (int j = 0; j < cluster_indices[i].indices.size(); j++)
{
std::cout << cluster_indices[i].indices[j] << " ";
}
std::cout << std::endl;
}
return 0;
}
```
这段代码首先加载了一个点云数据,然后进行下采样、计算法线和特征等操作。接着使用pcl::KMeans对特征向量进行聚类,得到聚类结果。最后将聚类结果输出到控制台中。需要注意的是这里的特征类型是pcl::FPFHSignature33,如果使用其他类型的特征,需要将pcl::KMeans的模板参数替换成对应的类型。
pcl::EuclideanClusterExtraction 实现
pcl::EuclideanClusterExtraction 是一个基于欧几里得距离的点云聚类算法,它可以将点云中距离较近的点分为同一类别。
使用 EuclideanClusterExtraction 的步骤如下:
1. 构建点云数据结构对象,例如 pcl::PointCloud<pcl::PointXYZ> cloud。
2. 创建 pcl::search::KdTree<pcl::PointXYZ>::Ptr 对象实例,用于搜索点云中的近邻点。
3. 将点云数据结构对象 cloud 传递给搜索对象,例如 pcl::search::KdTree<pcl::PointXYZ>::Ptr tree(new pcl::search::KdTree<pcl::PointXYZ>),tree->setInputCloud(cloud)。
4. 创建 pcl::EuclideanClusterExtraction<pcl::PointXYZ> 对象实例,设置聚类的参数,例如 pcl::EuclideanClusterExtraction<pcl::PointXYZ> ec,ec.setClusterTolerance(0.02)。
5. 通过调用 ec.extract() 函数进行聚类,例如 std::vector<pcl::PointIndices> cluster_indices,ec.extract(cluster_indices)。
6. 遍历 cluster_indices,获取每个聚类的索引值,例如 for (std::vector<pcl::PointIndices>::const_iterator it = cluster_indices.begin(); it != cluster_indices.end(); ++it)。
7. 遍历每个聚类的索引值,获取点云中对应的点,例如 for (std::vector<int>::const_iterator pit = it->indices.begin(); pit != it->indices.end(); ++pit)。
8. 对于每个聚类,可以对其进行后续的处理,例如计算聚类的中心点、协方差矩阵等。
相关推荐
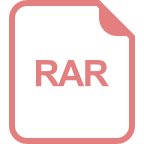
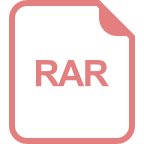












