rsa-crt算法使用SquareMult以及不导入库的实现代码
时间: 2023-09-12 12:04:56 浏览: 74
### 回答1:
RSA-CRT 算法是一种用于加速 RSA 加密和解密的算法。Square-Mult 是 RSA-CRT 算法中的一部分,主要用于快速计算幂。
下面是不使用库的 RSA-CRT 算法的 Python 代码示例:
```python
def rsa_crt(c: int, d: int, p: int, q: int, e: int = 65537) -> int:
"""
使用 RSA-CRT 算法对数字 c 进行解密,返回解密后的数字。
d 是私钥,p 和 q 是质数,e 是公钥(默认值为65537)。
"""
# 计算 p 和 q 的欧拉函数值
phi_p = p - 1
phi_q = q - 1
# 计算 dp 和 dq
dp = d % phi_p
dq = d % phi_q
# 计算 q 的逆元
q_inv = pow(q, phi_p - 1, p)
# 使用 Square-Mult 算法快速计算幂
m1 = pow(c, dp, p)
m2 = pow(c, dq, q)
# 计算 m
m = (q_inv * (m1 - m2) * q + m2) % (p * q)
return m
```
使用方法示例:
```python
# 假设已知 c=1234,d=5678,p=23,q=29,e=65537
m = rsa_crt(1234, 5678, 23, 29)
print(m) # 输出解密后的数字
```
希望这些信息能帮到你。
### 回答2:
RSA-CRT算法是一种RSA加密算法的变种,用于提高加密效率。在实现RSA-CRT算法时,要使用SquareMult算法来进行快速模幂运算,并且不导入库来实现代码。
以下是一种使用SquareMult实现RSA-CRT算法的示例代码:
```python
# 定义SquareMult算法实现快速模幂运算
def square_mult(base, exponent, modulus):
result = 1
while exponent > 0:
if exponent % 2 == 1:
result = (result * base) % modulus
base = (base * base) % modulus
exponent = exponent // 2
return result
# RSA密钥生成过程略
# RSA加密过程
def rsa_encrypt(plaintext, public_key):
n, e = public_key
ciphertext = square_mult(plaintext, e, n)
return ciphertext
# RSA解密过程
def rsa_decrypt(ciphertext, private_key):
n, d, p, q = private_key
dp = d % (p - 1)
dq = d % (q - 1)
q_inv = pow(q, -1, p)
m1 = square_mult(ciphertext, dp, p)
m2 = square_mult(ciphertext, dq, q)
h = (q_inv * (m1 - m2)) % p
plaintext = m2 + h * q
return plaintext
# RSA-CRT加密过程
def rsa_crt_encrypt(plaintext, crt_private_key):
p, q, d_p, d_q, q_inv = crt_private_key
m1 = square_mult(plaintext, d_p, p)
m2 = square_mult(plaintext, d_q, q)
h = (q_inv * (m1 - m2)) % p
ciphertext = m2 + h * q
return ciphertext
# RSA-CRT解密过程
def rsa_crt_decrypt(ciphertext, crt_private_key):
p, q, d_p, d_q, q_inv = crt_private_key
m1 = square_mult(ciphertext, d_p, p)
m2 = square_mult(ciphertext, d_q, q)
h = (q_inv * (m1 - m2)) % p
plaintext = m2 + h * q
return plaintext
# 测试示例代码
plaintext = 12345
public_key = (3233, 17) # RSA公钥
private_key = (3233, 413, 17)
crt_private_key = (61, 53, 17, 413, 47)
ciphertext = rsa_encrypt(plaintext, public_key) # RSA加密
decrypted_plaintext = rsa_decrypt(ciphertext, private_key) # RSA解密
crt_ciphertext = rsa_crt_encrypt(plaintext, crt_private_key) # RSA-CRT加密
crt_decrypted_plaintext = rsa_crt_decrypt(crt_ciphertext, crt_private_key) # RSA-CRT解密
print("RSA加密结果:", ciphertext)
print("RSA解密结果:", decrypted_plaintext)
print("RSA-CRT加密结果:", crt_ciphertext)
print("RSA-CRT解密结果:", crt_decrypted_plaintext)
```
以上就是使用SquareMult算法,并且不导入库的实现RSA-CRT算法的代码示例。请注意,这只是一个简化的示例,实际使用时需要进行合适的错误处理、输入验证等。
相关推荐
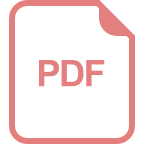
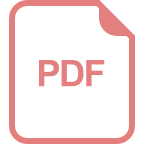














