用c++编一个程序,给定长度为 N 的数列 A。 请找出满足以下条件的整数 i (1≤i≤N) 的数量: 对于任意整数 j (1≤j≤N),满足 i !=j 时,A_i不能被 A_j整除。
时间: 2024-09-16 21:03:28 浏览: 37
为了编写一个 C++ 程序解决这个问题,首先我们需要创建一个数组 `A` 来存储给定的数列,并遍历数组,同时维护一个计数器 `count` 来统计满足条件的 `i`。以下是一个简单的 C++ 代码示例:
```cpp
#include <iostream>
#include <vector>
int count_non_divisible(int length, std::vector<int>& A) {
int count = length;
// 遍历数组,对于每个元素,检查是否能被其他元素整除
for (size_t i = 0; i < length; ++i) {
if (i == 0 || A[i] % A[0] != 0) { // 如果第一个元素不需要考虑,或者当前元素不能被第一个元素整除
for (size_t j = i + 1; j < length; ++j) {
if (A[j] % A[i] == 0) { // 如果发现当前元素能被后面的某个元素整除,则减少计数
--count;
break;
}
}
}
}
return count;
}
int main() {
int N; // 输入数列的长度
std::cout << "Enter the length of the array: ";
std::cin >> N;
std::vector<int> A(N); // 创建并读入数列
std::cout << "Enter the elements of the array: ";
for (int& element : A) {
std::cin >> element;
}
int result = count_non_divisible(N, A);
std::cout << "The number of indices that satisfy the condition is: " << result << std::endl;
return 0;
}
```
阅读全文
相关推荐
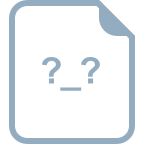
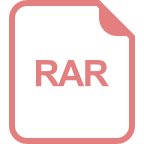












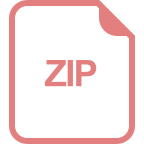
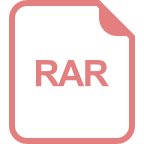
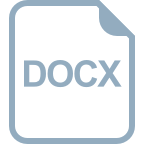