用c语言实现背包问题的分支限界算法
时间: 2024-11-03 19:05:36 浏览: 66
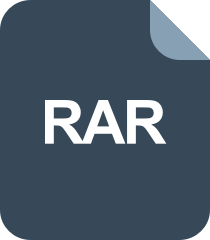
分支限界法求01背包c语言

在C语言中实现背包问题的分支限界法(Branch and Bound)通常涉及动态规划和贪心策略,但为了简化,这里我们将展示一个基于递归的简单版本。背包问题是计算机科学中的经典问题,目标是在给定容量限制下,选择物品使得总价值最大。
首先,我们需要定义几个函数:
1. `is_feasible()` - 检查当前状态(包含哪些物品、每个物品的数量)是否在容量范围内。
2. `value(item, weight, capacity)` - 计算包含特定物品的价值。
3. `branch_and_bound(state, capacity)` - 主函数,通过递归分治策略查找解。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义物品结构
typedef struct {
int value;
int weight;
} Item;
int is_feasible(Item *items, int num_items, int capacity) {
int total_weight = 0;
for (int i = 0; i < num_items; ++i)
total_weight += items[i].weight;
return total_weight <= capacity;
}
int value(Item *items, int item_index, int capacity) {
if (item_index == -1 || !capacity)
return 0;
else
return items[item_index].value + value(items, item_index + 1, capacity - items[item_index].weight);
}
int branch_and_bound(Item *items, int num_items, int capacity, int current_capacity, int best_value) {
// 如果达到最大深度或无法继续,则返回最佳值
if (!is_feasible(items, num_items, current_capacity))
return best_value;
// 遍历所有可能的选择
for (int i = 0; i < num_items && current_capacity >= items[i].weight; ++i) {
int new_capacity = current_capacity - items[i].weight;
int new_value = value(items, i, new_capacity);
// 更新当前最优解
if (new_value > best_value)
best_value = new_value;
// 递归处理剩余情况
best_value = branch_and_bound(items, num_items, capacity, new_capacity, best_value);
}
return best_value;
}
int main() {
// 初始化物品列表...
Item items[] = { /* ... */ };
int num_items = sizeof(items) / sizeof(items[0]);
int capacity = ...; // 背包容量
int optimal_value = branch_and_bound(items, num_items, capacity, capacity, 0);
printf("Optimal value with a %d-capacity backpack: %d\n", capacity, optimal_value);
return 0;
}
```
阅读全文
相关推荐
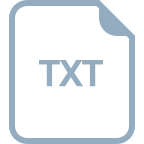
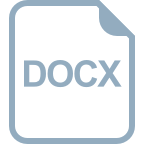








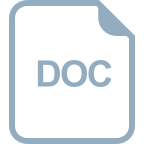

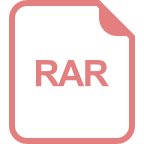




