用python写一段代码 实现旋转矩阵到rx,ry,rz的转化
时间: 2024-11-20 11:35:23 浏览: 15
在Python中,你可以使用NumPy库来方便地处理矩阵变换。这里是一个简单的例子,展示如何创建一个函数来实现绕X、Y、Z轴的旋转矩阵(Rx, Ry, Rz)。首先,你需要安装NumPy如果还没有的话,可以使用pip install numpy。
```python
import numpy as np
def rotation_matrix(axis, theta):
"""
Generate a rotation matrix given an axis and angle.
Parameters:
axis (list): A unit vector representing the axis of rotation.
theta (float): The angle of rotation in radians.
Returns:
np.ndarray: A 3x3 rotation matrix.
"""
axis = axis / np.linalg.norm(axis) # Normalize the axis
a, b, c = axis
cos_t = np.cos(theta)
sin_t = np.sin(theta)
# Create the rotation matrix
R = np.array([[cos_t + a**2 * (1 - cos_t), a * b * (1 - cos_t) - c * sin_t, a * c * (1 - cos_t) + b * sin_t],
[b * a * (1 - cos_t) + c * sin_t, cos_t + b**2 * (1 - cos_t), b * c * (1 - cos_t) - a * sin_t],
[c * a * (1 - cos_t) - b * sin_t, c * b * (1 - cos_t) + a * sin_t, cos_t + c**2 * (1 - cos_t)]])
return R
# Example usage: Rotate a matrix around X, Y, or Z axes
matrix = np.eye(3) # Your input matrix
rotated_x = rotation_matrix([1, 0, 0], np.pi/4) # 45-degree rotation around X
rotated_y = rotation_matrix([0, 1, 0], np.pi/2) # 90-degree rotation around Y
rotated_z = rotation_matrix([0, 0, 1], np.pi/3) # 60-degree rotation around Z
print("Rotation around X:")
print(rotated_x @ matrix)
print("\nRotation around Y:")
print(rotated_y @ matrix)
print("\nRotation around Z:")
print(rotated_z @ matrix)
阅读全文
相关推荐
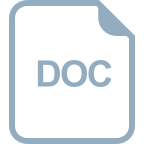
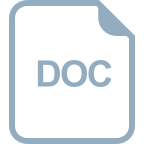
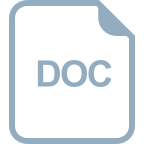





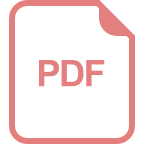
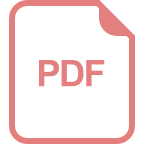
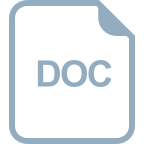







