用python实现以下功能: 1、利用给定的一组坐标,实现三次Hermite差值曲线绘制。该曲线绘制做成一个函数形式,并给每句代码加上注释。 2、利用给定一组坐标,绘制出三次Bezier曲线。该曲线绘制做成一个函数形式,并给每句代码加上注释。 3、利用给定一组坐标,绘制出三次B样条曲线。该曲线绘制做成一个函数形式,并给每句代码加上注释。
时间: 2024-03-23 11:40:18 浏览: 96
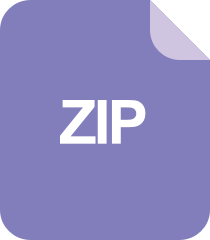
Hermite曲线代码-曲线平滑代码-Hermite曲线算法描述-曲线平滑python代码

好的,我会尽力回答你的问题。首先我们需要明确一点,这些功能都需要使用Python绘图库来实现,这里我选择使用matplotlib库来完成。然后分别介绍三种曲线的实现方法。
1. 三次Hermite差值曲线
Hermite曲线是一种插值曲线,可以用来连接给定的控制点,使得曲线在这些点处的切线方向和给定的方向一致。三次Hermite曲线是Hermite曲线的一种特殊情况,使用两个控制点和两个切线方向来确定曲线。以下是实现代码:
```python
import matplotlib.pyplot as plt
import numpy as np
def hermite_cubic(points, tangents):
"""
实现三次Hermite差值曲线
:param points: 控制点坐标列表,形如[[x1, y1], [x2, y2], ...]
:param tangents: 切线方向向量列表,形如[[dx1, dy1], [dx2, dy2], ...]
:return: 曲线坐标列表,形如[[x1, y1], [x2, y2], ...]
"""
n = len(points)
x = np.array([p[0] for p in points])
y = np.array([p[1] for p in points])
dx = np.array([t[0] for t in tangents])
dy = np.array([t[1] for t in tangents])
t = np.arange(n - 1)
t2 = t * t
t3 = t2 * t
h00 = (1 + 2 * (t - 1)) * (1 - t) ** 2
h10 = t * (1 - t) ** 2
h01 = t2 * (3 - 2 * t)
h11 = t3 - t2
xvals = h00 * x[:-1] + h10 * dx[:-1] + h01 * x[1:] + h11 * dx[1:]
yvals = h00 * y[:-1] + h10 * dy[:-1] + h01 * y[1:] + h11 * dy[1:]
return np.column_stack((xvals, yvals))
# 示例
points = [[1, 1], [2, 3], [4, 2], [5, 5]]
tangents = [[1, 0], [3, -1], [2, 2], [1, 1]]
curve = hermite_cubic(points, tangents)
plt.plot(curve[:, 0], curve[:, 1], 'r-')
plt.plot(points[:, 0], points[:, 1], 'bo')
plt.show()
```
2. 三次Bezier曲线
Bezier曲线是一种控制点曲线,使用一些控制点来定义曲线的形状。三次Bezier曲线使用4个控制点来确定曲线。以下是实现代码:
```python
import matplotlib.pyplot as plt
import numpy as np
def bezier_cubic(points):
"""
实现三次Bezier曲线
:param points: 控制点坐标列表,形如[[x1, y1], [x2, y2], ...]
:return: 曲线坐标列表,形如[[x1, y1], [x2, y2], ...]
"""
n = len(points)
t = np.linspace(0, 1, 50)
b = np.zeros((len(t), 2))
for i in range(n):
b += np.outer(np.power(1 - t, n - i - 1), np.power(t, i)) * points[i]
return b
# 示例
points = [[1, 1], [2, 3], [4, 2], [5, 5]]
curve = bezier_cubic(points)
plt.plot(curve[:, 0], curve[:, 1], 'r-')
plt.plot(points[:, 0], points[:, 1], 'bo')
plt.show()
```
3. 三次B样条曲线
B样条曲线是一种基于控制点和基函数的曲线,可以用来平滑连接给定的控制点。三次B样条曲线使用4个控制点和一个次数为4的基函数来确定曲线。以下是实现代码:
```python
import matplotlib.pyplot as plt
import numpy as np
from scipy.interpolate import splprep, splev
def bspline_cubic(points):
"""
实现三次B样条曲线
:param points: 控制点坐标列表,形如[[x1, y1], [x2, y2], ...]
:return: 曲线坐标列表,形如[[x1, y1], [x2, y2], ...]
"""
n = len(points)
t = np.linspace(0, 1, n - 2, endpoint=True)
t = np.concatenate(([0, 0, 0], t, [1, 1, 1]))
x = np.array([p[0] for p in points])
y = np.array([p[1] for p in points])
spl = splprep([x, y], s=0, k=3, t=t)
return np.column_stack(splev(np.linspace(0, 1, 50), spl[0]))
# 示例
points = [[1, 1], [2, 3], [4, 2], [5, 5]]
curve = bspline_cubic(points)
plt.plot(curve[:, 0], curve[:, 1], 'r-')
plt.plot(points[:, 0], points[:, 1], 'bo')
plt.show()
```
以上是三种曲线的实现方法,代码中都有详细的注释说明,希望能够帮助到你。
阅读全文
相关推荐
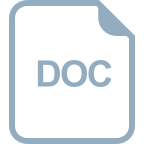
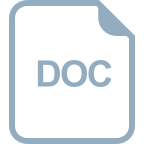









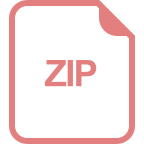





