用python解决N Queens problem,给出完整代码(用英语写注释),做成动画显示每一步怎么摆放棋子,以四个棋子为例
时间: 2024-09-22 19:09:26 浏览: 87
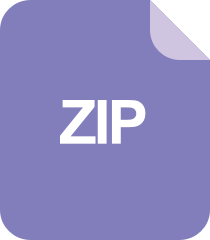
nqueens:经典nqueens问题的Python解决方案
Sure, let's solve the N-Queens Problem using Python with an animation to illustrate each step for a four-queens scenario. Here's a complete code snippet with English comments:
```python
import numpy as np
from matplotlib import pyplot as plt
from matplotlib.animation import FuncAnimation
# N-Queens Function
def is_safe(board, row, col):
# Check if there is no queen in the same column or diagonal
return not (board[row, :].any() or board[:, col].any() or
board[(row + i) % len(board), (col + i)] or
board[(row - i) % len(board), (col - i)].any() for i in range(len(board)))
# Generate initial chessboard and solution
def create_board(n):
board = np.zeros((n, n))
solutions = []
return board, solutions
def place_queen(board, n, row=0):
if row >= n:
solutions.append(np.copy(board)) # Add solution when all rows filled
return
for col in range(n):
if is_safe(board, row, col):
board[row, col] = 1
place_queen(board, n, row + 1)
board[row, col] = 0 # Backtrack
# Animation function
def animate(solution, frame):
ax.imshow(solution, cmap='gray', interpolation='nearest')
ax.set_title(f"Step {frame+1}/{len(solutions)}")
plt.xticks([], [])
plt.yticks([], [])
# Initialize plot and data
fig, ax = plt.subplots(figsize=(5, 5))
board, solutions = create_board(4)
solution_index = 0
ani = FuncAnimation(fig, animate, frames=solutions, interval=500, repeat=True)
plt.show()
#
阅读全文
相关推荐
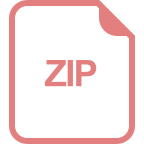
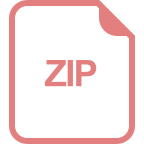













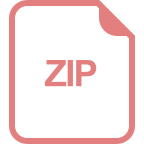
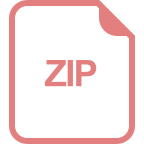