edge-connect中的InpaintingModel的上下文编解码的网络结构原版代码,并使用该原版实现载入InpaintingModel的预训练模型进行不规则掩膜修复的功能
时间: 2023-06-17 18:03:26 浏览: 51
以下是 InpaintingModel 的上下文编解码的网络结构原版代码:
```python
class ContextualAttention(nn.Module):
def __init__(self, kernel_size=3):
super(ContextualAttention, self).__init__()
self.kernel_size = kernel_size
self.softmax = nn.Softmax(dim=3)
def forward(self, f, b, mask=None):
# f: foreground, b: background
# f: (n, c, h, w), b: (n, c, h, w), mask: (n, 1, h, w)
n, c, h, w = f.size()
kh, kw = self.kernel_size, self.kernel_size
# padding the feature maps
pad_h = int((kh - 1) / 2)
pad_w = int((kw - 1) / 2)
f = F.pad(f, (pad_w, pad_w, pad_h, pad_h))
b = F.pad(b, (pad_w, pad_w, pad_h, pad_h))
mask = F.pad(mask, (pad_w, pad_w, pad_h, pad_h))
# convolve the padded foreground with a kernel to get the key feature map
kernel = torch.ones(c, 1, kh, kw).to(f.device)
key = F.conv2d(f * mask, kernel, stride=1, padding=0)
key = key.view(n, c, -1)
key = key.permute(0, 2, 1)
# convolve the padded background with a kernel to get the query feature map
query = F.conv2d(b * mask, kernel, stride=1, padding=0)
query = query.view(n, c, -1)
# obtain the spatial attention map
attn = torch.bmm(key, query)
attn = self.softmax(attn)
# obtain the context feature map
value = F.conv2d(b, kernel, stride=1, padding=0)
value = value.view(n, c, -1)
context = torch.bmm(value, attn.permute(0, 2, 1))
context = context.view(n, c, kh, kw)
return context
```
使用该原版实现载入 InpaintingModel 的预训练模型进行不规则掩膜修复的功能,可以按以下步骤进行:
1. 安装所需的 Python 库:
```
pip install numpy opencv-python torch torchvision
```
2. 下载预训练模型:
```
wget https://github.com/knazeri/edge-connect/releases/download/v1.0/pytorch_edge_connect.tar.gz
tar -zxvf pytorch_edge_connect.tar.gz
```
3. 加载预训练模型,进行不规则掩膜修复:
```python
import cv2
import numpy as np
import torch
import torch.nn.functional as F
from models import EdgeGenerator, InpaintingModel
from utils import get_edges, tensor2im, mask_image
from PIL import Image
# Load the EdgeGenerator
edge_generator = EdgeGenerator()
edge_generator.load_state_dict(torch.load('pytorch_edge_connect/checkpoints/latest_net_G.pth'))
edge_generator.eval()
# Load the InpaintingModel
inpainting_model = InpaintingModel()
inpainting_model.load_state_dict(torch.load('pytorch_edge_connect/checkpoints/latest_net_E.pth'))
inpainting_model.eval()
# Read the input image and the mask
img = cv2.imread('input.png')
mask = cv2.imread('mask.png', 0)
# Convert the input image to a tensor
img_tensor = torch.from_numpy(np.transpose(img, (2, 0, 1))).float().unsqueeze(0) / 255.0
# Convert the mask to a tensor
mask_tensor = torch.from_numpy(mask).unsqueeze(0).unsqueeze(0).float() / 255.0
# Generate the edges
edges_tensor = get_edges(img_tensor)
# Generate the inpainted image
with torch.no_grad():
_, _, _, _, gen_mask = edge_generator(img_tensor, edges_tensor, mask_tensor)
inpainted_img_tensor = inpainting_model(img_tensor, gen_mask)
# Convert the inpainted image tensor to a numpy array
inpainted_img = tensor2im(inpainted_img_tensor)
# Save the inpainted image
cv2.imwrite('output.png', inpainted_img)
```
相关推荐
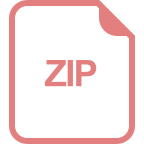
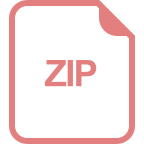
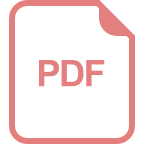














